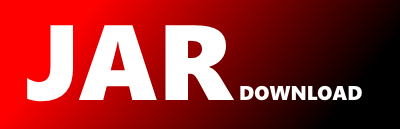
com.adobe.xfa.ut.MsgFormatPos Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.ut;
import java.util.ArrayList;
import java.util.List;
/**
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public final class MsgFormatPos {
private boolean mbResolved;
private int mnResId;
private MsgFormat moFmt;
private final List moParmPosns = new ArrayList();
private final List moParms = new ArrayList();
protected MsgFormatPos() {
}
/**
* Copy Constructor
*
* @param oSource
* MsgFormatPos to be copied
*/
public MsgFormatPos(MsgFormatPos oSource) {
moFmt = new MsgFormat(oSource.moFmt);
assign(oSource);
}
/**
* Resource Id Constructor
*
* @param nResId
* Resource Id representing a string to be position-sensitive
* formatted
*/
public MsgFormatPos(int nResId) {
moFmt = new MsgFormat(nResId);
mnResId = nResId;
init();
}
/**
* Resource Id Constructor
*
* @param nResId
* Resource Id representing a string to be position-sensitive
* formatted
* @param sOut
* input value to be applied to the format
*/
public MsgFormatPos(int nResId, String sOut) {
mnResId = nResId;
moFmt = new MsgFormat(mnResId);
init();
format(sOut);
}
/**
* String Constructor
*
* @param sSource
* string to be position-sensitive formatted
*/
public MsgFormatPos(String sSource) {
moFmt = new MsgFormat(sSource);
//mnResId = 0;
init();
}
/**
* String Constructor
*
* @param sSource
* string to be position-sensitive formatted
* @param sOut
* input value to be applied to the format
*/
public MsgFormatPos(String sSource, String sOut) {
moFmt = new MsgFormat(sSource, sOut);
//mnResId = 0;
init();
format(sOut);
}
/**
* Assignment operator.
*
* @param oSource
* jfFormat to be copied
*/
public MsgFormatPos assign(MsgFormatPos oSource) {
moFmt = oSource.moFmt;
mbResolved = oSource.mbResolved;
moParmPosns.addAll(oSource.moParmPosns);
moParms.addAll(oSource.moParms);
mnResId = oSource.mnResId;
return this;
}
/**
* Formatting operator.
*
* @param nResId
* Resource Id representing a string.
*/
public MsgFormatPos format(int nResId) {
return format(new MsgFormat(nResId).toString());
}
/**
* Formatting operator.
*
* @param sOut
* input value to be applied to the format
*/
public MsgFormatPos format(String sOut) {
mbResolved = false;
// keep this parameter for later when we resolve
moParms.add(sOut);
return this;
}
/**
* Retrieve the nth parameter as a string
*
* @return the nth parameter as a string
*/
public String getParm(int nIndex) {
// need to resolve, otherwise parameters won't be there or in the proper
// order
resolve();
return moFmt.getParm(nIndex);
}
/**
* Retrieve the size of the parameter list
*
* @return the size of the parameter list
*/
public int getParmCount() {
// need to resolve, otherwise parameters won't be there or in the proper
// order
resolve();
return moFmt.getParmCount();
}
// ------------------------------------------------------------------
//
// Init: Replace our format string with one that jfFormat will like
//
// ------------------------------------------------------------------
void init() {
mbResolved = false;
StringBuilder sSrc = new StringBuilder(moFmt.sourceString());
//String sSpecifiers = "s";
// Replace all our % formatting with %s, and track positions
// Similarly replace all our %[l][dfusxX] formatting with %s.
int nLen = sSrc.length();
for (int i = 0; i < nLen; i++) {
if (sSrc.charAt(i) == '%' && i < nLen) {
i++;
if ('0' <= sSrc.charAt(i) && sSrc.charAt(i) <= '9') {
// keep track of this position
moParmPosns.add(Integer.valueOf(sSrc.charAt(i) - '0'));
// Replace the digit with a string format character
sSrc.setCharAt(i, 's');
}
//
// Handle %s specifier as well.
// This way, resource strings originally formatted
// for MsgFormat can now be used with MsgFormatPos.
//
else if (sSrc.charAt(i) == 's') {
int nPos = moParmPosns.size();
moParmPosns.add(Integer.valueOf(nPos));
// Replace the specifier with a string format character
sSrc.setCharAt(i, 's');
}
}
}
moFmt = new MsgFormat(sSrc.toString());
}
/**
* Retrieve the resource id
*
* @return the resource id for this
*/
public int resId() {
return mnResId;
}
// --------------------------------------------------------------
//
// Resolve: Apply our parameters to the underlying jfFormat in
// the proper sequence
// --------------------------------------------------------------
private void resolve() {
mbResolved = true;
int nParms = moParms.size();
int nSize = moParmPosns.size();
if (nSize == 0 || nParms == 0)
return;
// Keep track of the lowest numbered parameter so that we can properly
// offset into the parameter array
int nMin = moParmPosns.get(0).intValue();
for (int i = 1; i < nSize; i++) {
if (moParmPosns.get(i).intValue() < nMin)
nMin = moParmPosns.get(i).intValue();
}
int i = 0;
for (; i < nSize; i++) {
// Normalize our position (zero based)
int n = moParmPosns.get(i).intValue() - nMin;
// Make sure we're in range
if (n >= nParms)
break;
moFmt.format(moParms.get(n));
}
// fill in any left over parameters
// with the empty string, otherwise
// text following missing parameters
// is lost
for (; i < nSize; i++) {
moFmt.format("");
}
moParms.clear();
moParmPosns.clear();
}
/**
* Retrieve the string representation
*
* @return the string version of this
*/
public String toString() {
resolve();
return moFmt.toString();
}
// TODO Figure out what to do with jfAtom
// /**
// * Resource Id Constructor
// *
// * @param nResId Resource Id representing a string to be
// position-sensitive formatted
// * @param aOut input value to be applied to the format
// */
// public MsgFormatPos( ResId nResId, jfAtom aOut)
// {
// moFmt = new MsgFormat(nResId);
// mnResId = nResId;
// init();
// format(aOut);
// }
//
// TODO Figure out what to do with jfAtom
// /**
// * String Constructor
// *
// * @param sSource string to be position-sensitive formatted
// * @param aOut input value to be applied to the format
// */
// public MsgFormatPos( String sSource, jfAtom aOut)
// {
// moFmt = new MsgFormat(sSource, aOut);
// mnResId = new ResId(0);
// init();
// format(aOut);
// }
//
// TODO figure out Atoms
// /**
// * Formatting operator.
// *
// * @param aOut input value to be applied to the format
// */
// public MsgFormatPos format (jfAtom aOut)
// {
// String sVal(aOut);
// return format(sVal);
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy