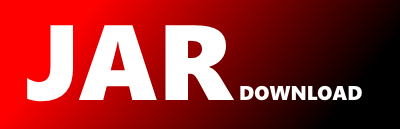
com.adobe.xmp.schema.model.rules.ClosedChoice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
// =================================================================================================
// ADOBE SYSTEMS INCORPORATED
// Copyright 2011 Adobe Systems Incorporated
// All Rights Reserved
//
// NOTICE: Adobe permits you to use, modify, and distribute this file in accordance with the terms
// of the Adobe license agreement accompanying it.
// =================================================================================================
package com.adobe.xmp.schema.model.rules;
import java.util.ArrayList;
import java.util.List;
import com.adobe.xmp.schema.model.SchemaVisitor;
import com.adobe.xmp.schema.model.TypeRule;
import com.adobe.xmp.schema.model.XMPSchemaException;
import com.adobe.xmp.schema.model.SimpleType.BasicType;
import com.adobe.xmp.schema.model.rules.Vocable.Type;
/**
* The rule covers the XMP types "closed choice".
* An arbitrary number of vocabulary can be added.
*
* @author Stefan Makswit
*/
public class ClosedChoice implements TypeRule
{
private static final long serialVersionUID = 1L;
/** List of allowed vocabulary */
private ArrayList vocabulary = new ArrayList();
/** the type of vocabulary */
private Type vocablularyType;
/**
* Constructor gets the type information of the Vocabulary.
*/
public ClosedChoice(Vocable.Type vocablularyType)
{
this.vocablularyType = vocablularyType;
}
/**
* Add a vocable.
*
* @param xmpValue the XMP value, usually a String,
* but in some cases it can also be of another type.
* @param label the display label for the vocable.
* @throws XMPSchemaException Throws an exception if a vocable with the wrong type is added
*/
public void addVocable(Object xmpValue, String label) throws XMPSchemaException
{
checkType(xmpValue);
vocabulary.add(new Vocable(xmpValue, label));
}
/**
* Adds a vocable.
*
* @param vocable a Vocable
* @throws XMPSchemaException Throws an exception if a vocable with the wrong type is added
*/
public void addVocable(Vocable vocable) throws XMPSchemaException
{
checkType(vocable.getXmpValue());
vocabulary.add(vocable);
}
/**
* @return Returns all vocabulary.
*/
public List getVocabulary()
{
return vocabulary;
}
/**
* @see com.adobe.xmp.schema.model.TypeRule#accept(com.adobe.xmp.schema.model.SchemaVisitor)
*/
public void accept(SchemaVisitor schemaVisitor) throws XMPSchemaException
{
schemaVisitor.visit(this);
}
/**
* @param xmpValue
* @throws XMPSchemaException
*/
private void checkType(Object xmpValue) throws XMPSchemaException
{
switch (vocablularyType)
{
case TEXT:
if (!(xmpValue instanceof String))
{
throw new XMPSchemaException("Vocable has to be of type '" + BasicType.TEXT.toString() + "'");
}
break;
case INTEGER:
if (!(xmpValue instanceof Integer) && !(xmpValue instanceof Long))
{
throw new XMPSchemaException("Vocable has to be of type '" + BasicType.INTEGER.toString() + "' (Integer in Java)");
}
break;
case REAL:
if (!(xmpValue instanceof Float) && !(xmpValue instanceof Double))
{
throw new XMPSchemaException("Vocable has to be of type '" + BasicType.REAL.toString() + "' (Double in Java)");
}
break;
case BOOLEAN:
if (!(xmpValue instanceof Boolean))
{
throw new XMPSchemaException("Vocable has to be of type '" + BasicType.BOOLEAN.toString() + "' (Boolean in Java)");
}
break;
case DATE:
if (!(xmpValue instanceof String))
{
throw new XMPSchemaException("Vocable has to be of type '" + BasicType.DATE.toString() + "' (a String in ISO8601 format in Java)");
}
break;
case ARRAY_OF_INTEGER:
if (!(xmpValue instanceof Integer[]) && !(xmpValue instanceof int[]))
{
throw new XMPSchemaException("Vocable has to an array of integer, ie. Integer[] or int[] in java)");
}
break;
default:
// IGNORE
break;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy