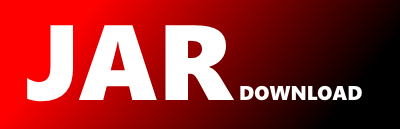
com.day.cq.analytics.testandtarget.PerformanceReportRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2014 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.analytics.testandtarget;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
/**
* The {@code PerformanceReportRequest} encapsulates all needed data for a
* {@link TestandtargetService#getPerformanceReport(com.day.cq.wcm.webservicesupport.Configuration, PerformanceReportRequest)}
* call
*
*/
public class PerformanceReportRequest {
private SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd'T'HH");
private long campaignId;
private Date start;
private Date end;
private String successMetric;
private String step;
/**
* Creates a new instance for the specified campaignId
*
* @param campaignId the id of the Test&Target campaign
* @return a new request instance
*/
public static PerformanceReportRequest forCampaignId(long campaignId) {
if (campaignId <= 0) {
throw new IllegalArgumentException("Expected campaignId > 0, but got " + campaignId);
}
PerformanceReportRequest request = new PerformanceReportRequest();
request.campaignId = campaignId;
return request;
}
public PerformanceReportRequest setStart(Date start) {
this.start = start;
return this;
}
public PerformanceReportRequest setEnd(Date end) {
this.end = end;
return this;
}
public PerformanceReportRequest setSuccessMetric(String successMetric) {
this.successMetric = successMetric;
return this;
}
public PerformanceReportRequest setStep(String step) {
this.step = step;
return this;
}
public String getSuccessMetric() {
return successMetric;
}
public String getStep() {
return step;
}
public long getCampaignId() {
return campaignId;
}
public Date getStart() {
return start;
}
public Date getEnd() {
return end;
}
/**
* Gets the TnT report parameter map for this request
*
* @return a {@code Map} containing the report request parameters
*/
public Map getParameters() {
Map paramMap = new HashMap();
addParameter(paramMap, "successMetric", successMetric);
addParameter(paramMap, "step", step);
return paramMap;
}
private void addParameter(Map destMap, String key, Object value) {
if (destMap != null
&& key != null
&& value != null) {
String valueStr = null;
if (value instanceof Date) {
valueStr = sdf.format((Date)value);
} else {
valueStr = value.toString();
}
destMap.put(key, valueStr);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy