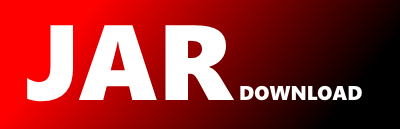
com.day.cq.analytics.testandtarget.TestandtargetCallOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*******************************************************************************
* ADOBE CONFIDENTIAL
* __________________
* Copyright 2014 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
******************************************************************************/
package com.day.cq.analytics.testandtarget;
import org.osgi.annotation.versioning.ProviderType;
/**
* Provides a nice way of grouping the options of an API call. Those options include (but are not limited to) the API
* location, the API method (GET, POST etc.), the API version to use etc.
*/
@ProviderType
public class TestandtargetCallOptions {
private TestandtargetHttpClient.TestandtargetMethodType callMethod = TestandtargetHttpClient.TestandtargetMethodType.GET;
private String clientCode;
private String location;
private TestandtargetHttpClient.TestandtargetSolution solution = TestandtargetHttpClient.TestandtargetSolution.TARGET;
private TestandtargetHttpParameters httpParameters;
private String apiVersion;
/**
* Adds the call method to the options.
*
* @param callMethod the HTTP method (GET, POST etc)
* @return an instance of this object
*/
public TestandtargetCallOptions withCallMethod(TestandtargetHttpClient.TestandtargetMethodType callMethod) {
this.callMethod = callMethod;
return this;
}
/**
* Sets the client code
*
* @param clientCode the client code
* @return an instance of this object
*/
public TestandtargetCallOptions withClientCode(String clientCode) {
this.clientCode = clientCode;
return this;
}
/**
* Sets the API location to invoke.
*
* @param location the API location to invoke (e.g. {@code /rest/v1/campaign} or {@code /rest/v5/segments}
* @return an instance of this object
*/
public TestandtargetCallOptions withLocation(String location) {
this.location = location;
return this;
}
/**
* Sets the Adobe Target solution for which the options object is invoked
*
* @param solution the Adobe Target solution to use (Target or Target Recommendation)
* @return an instance of this object
*/
public TestandtargetCallOptions withSolution(TestandtargetHttpClient.TestandtargetSolution solution) {
this.solution = solution;
return this;
}
/**
* Sets the HTTP parameters
*
* @param httpParameters the additional HTTP parameters
* @return an instance of this object
*/
public TestandtargetCallOptions withParameters(TestandtargetHttpParameters httpParameters) {
this.httpParameters = httpParameters;
return this;
}
/**
* Sets the API version to invoke.
*
* @param apiVersion the API version to invoke. If {@code null} is supplied then the v1 version is used.
* @return an instance of this object
*/
public TestandtargetCallOptions withApiVersion(String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Returns the call method (GET, POST etc.)
* @return a {@link TestandtargetHttpClient.TestandtargetMethodType} object representing the method
*/
public TestandtargetHttpClient.TestandtargetMethodType getCallMethod() {
return callMethod;
}
/**
* Returns the client code.
* @return a {@link String} object representing the client code
*/
public String getClientCode() {
return clientCode;
}
/**
* Returns API location to use
* @return a {@link String} object representing the location (e.g. {@code campaign/23.json}
*/
public String getLocation() {
return location;
}
/**
* Returns the solution to be used.
* @return a {@link TestandtargetHttpClient.TestandtargetSolution} object representing the method
*/
public TestandtargetHttpClient.TestandtargetSolution getSolution() {
return solution;
}
/**
* Returns the HTTP parameters.
* @return a {@link TestandtargetHttpParameters} object representing the additional HTTP parameters.
*/
public TestandtargetHttpParameters getHttpParameters() {
return httpParameters;
}
/**
* Returns the API version used.
* @return a {@link String} object representing the API version.
*/
public String getApiVersion() {
return apiVersion;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy