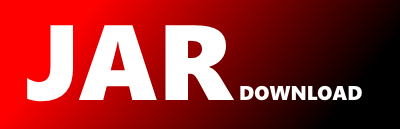
com.day.cq.dam.api.s7dam.scene7.ImageUrlApi Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2016 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*/
package com.day.cq.dam.api.s7dam.scene7;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import org.jetbrains.annotations.Nullable;
import org.osgi.annotation.versioning.ProviderType;
import com.day.cq.dynamicmedia.api.NotNullApi;
@ProviderType
@NotNullApi
public interface ImageUrlApi {
/**
* Retrieve the preview server url of the asset if it is on the ips server
* @param resource Resource for asset instance
* @param resolver Resource's resolver
* @return The url of the publish server, or null if could not get
* @deprecated Use getImageServerPreviewURL(Resource resource)
*/
@Deprecated
@Nullable
String getImageServerPreviewURL(Resource resource, ResourceResolver resolver);
/**
* Retrieve the delivery server url of the asset if it is on the ips server
* @param resource Resource for asset instance
* @param resolver Resource's resolver
* @return The url of the publish server, or null if could not get
* @deprecated Use getImageServerDeliveryURL(Resource resource)
*/
@Deprecated
@Nullable
String getImageServerDeliveryURL(Resource resource, ResourceResolver resolver);
/**
* Retrieve the path of the asset on the ips server
* @param resource Resource for asset instance
* @param resolver Resource's resolver
* @return The path of the resource, or null if could not get
* @deprecated Use getImageRootPath(Resource resource)
*/
@Deprecated
@Nullable
String getImageRootPath(Resource resource, ResourceResolver resolver);
/**
* Retrieve the preview server url of the asset if it is on the ips server
* @param resource Resource for asset instance
* @return The url of the publish server, or null if could not get
*/
@Nullable
String getImageServerPreviewURL(Resource resource);
/**
* Retrieve the auth token for preview server for the asset
* @param resource Resource for asset instance
* @return The jwt for secure preview, or null if could not get
*/
@Nullable
String getImageServerPreviewToken(Resource resource);
/**
* Retrieve the delivery server url of the asset if it is on the ips server
* @param resource Resource for asset instance
* @return The url of the publish server, or null if could not get
*/
@Nullable
String getImageServerDeliveryURL(Resource resource);
/**
* Retrieve the path of the asset on the ips server
* @param resource Resource for asset instance
* @return The path of the resource, or null if could not get
*/
@Nullable
String getImageRootPath(Resource resource);
/**
* Format of remote preview/publish URL link to DM asset. Possible values:
* {@link RemoteUrlType#SHORT} [Default]
*
{@link RemoteUrlType#LONG}
*/
enum RemoteUrlType {
/**
* Flat form of remote preview/publish URL link to DM asset. It is default for most uses:
* {@code http[s]://server[:port]/is/(image|content)//}
*/
SHORT,
/**
* Long form of remote preview/publish URL link to DM asset:
* {@code http[s]://server[:port]/is/(image|content)///..//}
*/
LONG
}
/**
* Returns published ("delivery") world-visible URL link to remote DM asset linked to the given JCR Resource.
*
* @param resource JCR resource pointing to the asset
* @param urlType format of URL
* @return publish URL string, or {@code null} if given resource is not DM-synchronized asset,
* or asset is not published, or any other error.
*/
@Nullable
String getRemoteAssetPublishURL(Resource resource, RemoteUrlType urlType);
/**
* Returns secure-preview URL link to remote DM asset linked to the given JCR Resource.
*
* @param resource JCR resource pointing to the asset
* @param urlType format of URL
* @return preview URL string, or {@code null} if given resource is not DM-synchronized asset,
* or any other error.
*/
@Nullable
String getRemoteAssetPreviewURL(Resource resource, RemoteUrlType urlType);
}