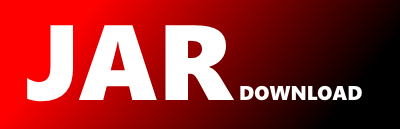
com.day.cq.dam.api.s7dam.set.SpinSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2013 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.api.s7dam.set;
import org.apache.sling.api.resource.Resource;
import org.osgi.annotation.versioning.ProviderType;
import com.day.cq.dam.api.Asset;
/**
* Represents a Scene7 2D SpinSet
* @deprecated Not for public use - this API will be replaced in a future release
*/
@Deprecated
@ProviderType
public interface SpinSet extends Resource {
/**
* Add the matrix of assets
*
* @param assets The 2D matrix consisting of assets
* @throws IllegalArgumentException if null array is passed.
*/
public void setAssets(Asset[][] assets);
/**
* Set the given asset at specified position in matrix.
*
* @param asset Asset to be added.
* @param row Row at which asset needs to be added.
* @param column Column at which asset needs to be added.
* @throws IllegalArgumentException if invalid value is passed for row or
* column
*/
public void setAsset(Asset asset, int row, int column);
/**
* Removes all the assets from set
*/
public void removeAssets();
/**
* Removes the specified row from the set. 0 th index is the first row.
*
* @param row Index of the row to be removed
* @throws IllegalArgumentException if invalid value is passed for row.
*/
public void removeRow(int row);
/**
* Removes the specified column from the set. 0th index is the first column
*
* @param column Index of the column to be removed.
* @throws IllegalArgumentException if invalid value is passed for column.
*/
public void removeColumn(int column);
/**
* Returns the asset at specified index in the matrix
*
* @param row The row index.
* @param column The column index
* @return Asset at specified column, null if it cannot find row.
* @throws IllegalArgumentException if invalid value is passed for row or
* column.
*/
public Asset getAsset(int row, int column);
/**
* Returns the number of rows in 2D spin set
*
* @return Number of rows
*/
public int getRowCount();
/**
* Returns the number of columns in 2D spin set
*
* @return Number of columns
*/
public int getColumnCount();
/**
* Returns the 2D array of assets which consists of spin set.
*
* @return The array of assets.
*/
public Asset[][] getAssets();
/**
* Adds row to the Set.
*
* @param row Array containing row to be added.
* @throws IllegalArgumentException if the dimension of row does not match
* with existing matrix
*/
public void addRow(Asset[] row);
/**
* Adds column to the set.
*
* @param column Array containing column to be added.
* @throws IllegalArgumentException if the dimension of column does not
* match with existing matrix.
*/
public void addColumn(Asset[] column);
/**
* Checks if the given asset is valid member type of Media Set
*
* @param asset The asset whose type needs to be checked for membership
* @return True if asset type is valid for set, false otherwise.
*/
boolean isValidMemberType(Asset asset);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy