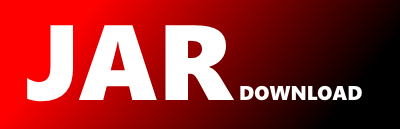
com.day.cq.dam.commons.util.PathToIDValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.day.cq.dam.commons.util;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.StringJoiner;
import javax.jcr.ItemNotFoundException;
import javax.jcr.Node;
import javax.jcr.RepositoryException;
import javax.jcr.Session;
import org.apache.commons.lang.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class PathToIDValidator {
private static final Logger LOG = LoggerFactory.getLogger(PathToIDValidator.class);
public static Map getActualPaths(Session session, String[] paths, String[] ids) {
if( paths == null ) {
throw new IllegalStateException("Paths must not be null");
}
if( ids == null ) {
throw new IllegalStateException("Ids must not be null");
}
if( paths.length != ids.length) {
String joiner = new StringJoiner(" ")
.add("Length Mismatch: Paths:")
.add(String.valueOf(paths.length))
.add("ids:")
.add(String.valueOf(ids.length)).toString();
throw new IllegalStateException(joiner);
}
Map result = new LinkedHashMap();
for( int i = 0; i< paths.length; i++ ) {
result.put(paths[i], getActualPath(session, paths[i], ids[i]));
}
return result;
}
public static String getActualPath(Session session, String path, String id) {
try {
if(session.nodeExists(path)) {
Node node = session.getNode(path);
String identifier = node.getIdentifier();
if( StringUtils.equals(id, identifier) || StringUtils.isEmpty(identifier)) {
return path;
}
else {
return getSafePath(session, id);
}
}
else {
return getSafePath(session, id);
}
}
catch( RepositoryException e) {
LOG.warn("Exception mapping '{}' to '{}'", new String[]{path, id}, e);
}
return null;
}
private static String getSafePath(Session session, String identifier) throws ItemNotFoundException, RepositoryException {
Node nodeById = session.getNodeByIdentifier(identifier);
if( nodeById != null ) {
return nodeById.getPath();
}
else {
LOG.debug("Node not found by id '{}'", identifier);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy