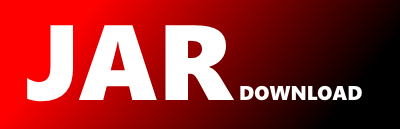
com.day.cq.dam.commons.util.S7SetHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2013 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.commons.util;
import java.util.Map;
import java.util.HashMap;
import org.apache.sling.api.resource.ModifiableValueMap;
import org.apache.sling.api.resource.PersistenceException;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import org.apache.sling.api.resource.ValueMap;
import com.adobe.granite.asset.api.Asset;
import com.adobe.granite.asset.api.AssetManager;
import com.day.cq.commons.jcr.JcrConstants;
import com.day.cq.dam.api.DamConstants;
import com.day.cq.dam.api.s7dam.constants.S7damConstants;
import com.day.cq.dam.api.s7dam.set.ImageSet;
import com.day.cq.dam.api.s7dam.set.MediaSet;
import com.day.cq.dam.api.s7dam.set.SpinSet;
import com.day.cq.dam.api.s7dam.set.SwatchSet;
/**
* Helper methods for S7 Sets
*/
public class S7SetHelper {
private static String MIME_TYPE = "Multipart/Related; type=application/x-";
/**
* Helper method to create S7 ImageSet
*
* @param parent
* parent {@link Resource} where new set to be created
* @param name
* name of the set to be created.
* @param props
* additional properties to be saved i.e. jcr:title
* @return an instance of {@link ImageSet}
* @throws PersistenceException if there is any issue in creating
* the set.
* IllegalArgumentException if the parent resource
* is null or name is null
*/
public static ImageSet createS7ImageSet(Resource parent, String name,
Map props) throws PersistenceException {
Resource resource = createS7Set(parent, name, props,
S7damConstants.S7_IMAGE_SET);
return resource.adaptTo(ImageSet.class);
}
private static Resource createS7Set(Resource parent, String name,
Map props, String type) throws PersistenceException {
return createS7Set(parent, name, props, type, null);
}
private static Resource createS7Set(Resource parent, String name,
Map props,
String type,
Map metadata) throws PersistenceException {
// may be type can be enum.
if (parent != null && name != null) {
ResourceResolver resolver = parent.getResourceResolver();
String path = parent.getPath() + "/" + name;
AssetManager am = resolver.adaptTo((AssetManager.class));
Asset asset = am.createAsset(path);
ValueMap vm = asset.adaptTo(ValueMap.class);
vm.put(S7damConstants.PN_S7_TYPE, type);
if (props != null) {
vm.putAll(props);
}
Resource metadataResource = resolver.getResource(asset,
JcrConstants.JCR_CONTENT + "/"
+ DamConstants.METADATA_FOLDER);
// set the mime type
ModifiableValueMap metadataVm = metadataResource
.adaptTo(ModifiableValueMap.class);
metadataVm.put(DamConstants.DC_FORMAT, MIME_TYPE + type);
if (metadata != null) {
metadataVm.putAll(metadata);
}
resolver.commit();
return resolver.getResource(path);
} else {
throw new IllegalArgumentException(
"parent resource or name can not be null");
}
}
/**
* Helper method to create S7 SwatchSet
*
* @param parent
* parent {@link Resource} where new set to be created
* @param name
* name of the set to be created.
* @param props
* additional properties to be saved i.e. jcr:title
* @return an instance of {@link SwatchSet}
* @throws PersistenceException if there is any issue in creating
* the set.
* IllegalArgumentException if the parent resource
* is null
*/
public static SwatchSet createS7SwatchSet(Resource parent, String name,
Map props) throws PersistenceException {
Resource resource = createS7Set(parent, name, props,
S7damConstants.S7_SWATCH_SET);
return resource.adaptTo(SwatchSet.class);
}
/**
* Helper method to create S7 VideoSet
*
* @param parent
* parent {@link Resource} where new set to be created
* @param name
* name of the set to be created.
* @param props
* additional properties to be saved i.e. jcr:title
* @return an instance of {@link MediaSet}
* @throws PersistenceException if there is any issue in creating
* the set.
* IllegalArgumentException if the parent resource
* is null
*/
public static MediaSet createS7VideoSet(Resource parent, String name,
Map props) throws PersistenceException {
Resource resource = createS7Set(parent, name, props,
S7damConstants.S7_VIDEO_SET);
return resource.adaptTo(MediaSet.class);
}
/**
* Helper method to create S7 Mixed Media Set
*
* @param parent
* parent {@link Resource} where new set to be created
* @param name
* name of the set to be created.
* @param props
* additional properties to be saved i.e. jcr:title
* @return an instance of {@link MediaSet}
* @throws PersistenceException if there is any issue in creating
* the set.
* IllegalArgumentException if the parent resource
* is null
*/
public static MediaSet createS7MixedMediaSet(Resource parent, String name,
Map props) throws PersistenceException {
Resource resource = createS7Set(parent, name, props,
S7damConstants.S7_MIXED_MEDIA_SET);
return resource.adaptTo(MediaSet.class);
}
/**
* Helper method to create spin Set
*
* @param parent
* parent {@link Resource} where new set to be created
* @param name
* name of the set to be created.
* @param props
* additional properties to be saved i.e. jcr:title
* @return an instance of {@link MediaSet}
* @throws PersistenceException if there is any issue in creating
* the set.
* IllegalArgumentException if the parent resource
* is null
*/
public static SpinSet createS7SpinSet(Resource parent, String name,
Map props) throws PersistenceException {
Resource resource = createS7Set(parent, name, props,
S7damConstants.S7_SPIN_SET);
return resource.adaptTo(SpinSet.class);
}
/**
* Checks whether the given resource represent a S7 set or not.
* @param resource resource instance
* @return true
if the resource represent a S7 set
* false
if the resource is not a S7 set
*
* @throws NullPointerException if resource is null
*/
public static boolean isS7Set(Resource resource) {
Resource contentResource = resource.getChild(JcrConstants.JCR_CONTENT);
if (contentResource != null) {
ValueMap vm = contentResource.adaptTo(ValueMap.class);
String s7Type = vm.get(S7damConstants.PN_S7_TYPE, "");
if (S7damConstants.S7_IMAGE_SET.equals(s7Type)
|| S7damConstants.S7_SWATCH_SET.equals(s7Type)
|| S7damConstants.S7_VIDEO_SET.equals(s7Type)
|| S7damConstants.S7_MIXED_MEDIA_SET.equals(s7Type)
|| S7damConstants.S7_SPIN_SET.equals(s7Type)
|| "CarouselSet".equals(s7Type)
|| "ECatalog".equals(s7Type)) {
return true;
}
}
return false;
}
/**
* Checks if the resource represents an S7 video.
* @param resource resource instance
* @return true
if the resource represent a S7 set
* false
if the resource is not a S7 set
*/
public static boolean isS7Video(Resource resource) {
Resource contentResource = resource.getChild(JcrConstants.JCR_CONTENT);
if (contentResource != null) {
ValueMap vm = contentResource.adaptTo(ValueMap.class);
String s7Type = vm.get(S7damConstants.PN_S7_TYPE, "");
boolean isVideo = (S7damConstants.S7_VIDEO.equals(s7Type) || S7damConstants.S7_VIDEO_AVS.equals(s7Type));
return isVideo;
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy