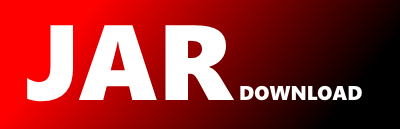
com.day.cq.dam.scene7.api.Scene7APIClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.scene7.api;
import com.day.cq.dam.scene7.api.constants.Scene7JobType;
import com.day.cq.dam.scene7.api.model.IpsApiException;
import com.day.cq.dam.scene7.api.model.MetadataCondition;
import com.day.cq.dam.scene7.api.model.Scene7Asset;
import com.day.cq.dam.scene7.api.model.Scene7CompanyMembership;
import com.day.cq.dam.scene7.api.model.Scene7ConfigSetting;
import com.day.cq.dam.scene7.api.model.Scene7ImageFieldUpdate;
import com.day.cq.dam.scene7.api.model.Scene7ImageMapDefinition;
import com.day.cq.dam.scene7.api.model.Scene7MetadataUpdate;
import com.scene7.ipsapi.ConfigSettingArray;
import com.scene7.ipsapi.GetCompanyInfoReturn;
import com.scene7.ipsapi.GetCompanySettingsReturn;
import com.scene7.ipsapi.GetPublishContextsReturn;
import com.scene7.ipsapi.ImageSetMemberUpdateArray;
import com.scene7.ipsapi.LanguageFileNameMappingArray;
import com.scene7.ipsapi.PropertyArray;
import com.scene7.ipsapi.SetCompanySettingsParam;
import com.scene7.ipsapi.SmartCropAddArray;
import com.scene7.ipsapi.SmartCropUpdateArray;
import com.scene7.ipsapi.ThumbAssetUpdate;
import com.scene7.ipsapi.UrlArray;
import org.apache.sling.api.resource.ResourceResolver;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.joda.time.Instant;
import org.osgi.annotation.versioning.ProviderType;
import org.w3c.dom.Document;
import java.io.IOException;
import java.io.InputStream;
import java.net.URISyntaxException;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* The Scene7APIClient provides the Scene7 SPS API methods used by CQ DAM. For more details about the methods please consult the SPS API.
*
* Return values and parameters are @NotNull by default.
*/
@ProviderType
public interface Scene7APIClient {
/**
* default content-type (i.e. 'application/soap+xml'), defaults to 'text/xml'
*/
static final String CONTENTTYPE = "text/xml";
static final int RECORDS_PER_PAGE_NO_FILTER = 1000;
static final int RECORDS_PER_PAGE_WITH_FILTER = 10000;
/**
* Deletes an asset from Scene7.
*
* @param assetHandle
* the handle to the asset to delete
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document deleteAsset(String assetHandle, S7Config s7Config)
throws IpsApiException;
/**
* Delete multiple assets from Scene7.
*
* @param assetHandleArr
* the handle to the asset to delete
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document deleteAssets(String[] assetHandleArr, S7Config s7Config)
throws IpsApiException;
/**
* Deletes a folder from Scene7.
*
* @param folderHandle
* the handle to the folder to delete
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document deleteFolder(String folderHandle, S7Config s7Config)
throws IpsApiException;
/**
* Gets all jobs that are currently active in the system.
*
* @param jobHandle
* the handle to the job
* @param originalName
* the original name of the job
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getActiveJobs(String jobHandle, String originalName,
S7Config s7Config) throws IpsApiException;
/**
* Gets assets from IPS.
*
* @param assetHandles
* the asset handles
* @param responseFields
* a list of fields and subfields for inclusion in the response
* @param excludeFields
* a list of fields and subfields for exclusion from the response
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getAssets(String[] assetHandles, String[] responseFields,
String[] excludeFields, S7Config s7Config) throws IpsApiException;
/**
* Gets assets associated with the specified asset and details about their
* relationship.
*
* @param assetHandle
* the handle to the asset
* @param responseFields
* a list of fields and subfields for inclusion in the response
* @param excludeFields
* a list of fields and subfields for exclusion from the response
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getAssociatedAssets(String assetHandle, String[] responseFields,
String[] excludeFields, S7Config s7Config) throws IpsApiException;
/**
* Gets assets by name.
*
* @param companyHandle
* the handle to the company
* @param responseFields
* a list of fields and subfields for inclusion in the response
* @param names a string array of asset names
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
List getAssetsByName(String companyHandle, String[] responseFields,
String[] names) throws IpsApiException;
/**
* Gets assets by name.
*
* @param companyHandle
* the handle to the company
* @param responseFields
* a list of fields and subfields for inclusion in the response
* @param names a string array of asset names
* @param s7config Scene7 config
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
List getAssetsByName(String companyHandle, String[] responseFields,
String[] names, S7Config s7config) throws IpsApiException;
/**
* Gets assethandle
* @param assetName a String array of asset names
* @return String assetHandle
* @throws IpsApiException
*/
String getAssetHandleByName(String assetName) throws IpsApiException;
/**
* Search asset by name and return its handle, if exists.
*
* @param s7Config configuration to access Scene7 server
* @param assetName asset name to look up
* @return asset handle, or null if not found
* @throws IpsApiException on error at Scene7
*/
@Nullable
String getAssetHandleByName(S7Config s7Config, String assetName) throws IpsApiException;
/**
* Gets a user's memberships.
*
* @param resourceResolver
* resource resolver used to access Scene7 configuration
* @param userHandle
* the handle to the user whose memberships you want to obtain
* @param email
* the user's email
* @param password
* the user's password
* @param region
* the user's region
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getCompanyMembership(ResourceResolver resourceResolver,
String userHandle, String email, String password, String region)
throws IpsApiException;
/**
* Gets folders and sub-folders in a hierarchical tree structure. The
* getFolderTree response is limited to a maximum of 100000 folders.
*
* @param folderPath
* the root folder to retrieve folders and all subfolders to the
* leaflevel; if excluded, the company root is used
* @param depth
* a value of zero gets the top-level folder; any other value
* specifies the depth to descend into the tree
* @param responseFields
* a list of fields and subfields for inclusion in the response
* @param excludeFields
* a list of fields and subfields for exclusion from the response
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getFolderTree(String folderPath, int depth,
String[] responseFields, String[] excludeFields, S7Config s7Config)
throws IpsApiException;
/**
* Gets the details of job logs of a company.
*
* @param jobHandle
* a handle to an active or completed job
* @param originalName
* the original name of the job log
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getJobLogDetails(String jobHandle, String originalName,
S7Config s7Config) throws IpsApiException;
/**
* Gets the details of job logs of a company with specified parameters
*
* @param jobHandle
* a handle to an active or completed job
* @param originalName
* the original name of the job log
* @param resultsPage
* page number of recordsPerPage-results to return
* @param recordsPerPage
* maximum number of detailArray items to return
* @param sortBy
* one of the Job Detail Sort Field constant values (Date or
* LogType)
* @param sortDirection
* one of the Sort Direction string constants
* @param s7Config
* the Scene7 configuration
* @return the jobLogDetails API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getJobLogDetails(String jobHandle, String originalName,
int resultsPage, int recordsPerPage, String sortBy,
String sortDirection, S7Config s7Config) throws IpsApiException;
/**
* Gets property sets associated with a type handle.
*
* @param appSettingsTypeHandle
* the handle to the property set type
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getPropertySets(String appSettingsTypeHandle, S7Config s7Config)
throws IpsApiException;
/**
* Create PropertySet of given type, and store given Properties to it.
*
* @param typeHandle PropertySet type handle
* @param props array of Properties to put into new PropertySet
* @param s7Config the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException .
*/
Document createPropertySet(String typeHandle, PropertyArray props, S7Config s7Config) throws IpsApiException;
/**
* Update PropertySet with the new set of Properties, replacing existing ones.
*
* @param setHandle PropertySet handle
* @param props array of Properties to put into PropsertySet
* @param s7Config the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException .
*/
Document updatePropertySet(String setHandle, PropertyArray props, S7Config s7Config) throws IpsApiException;
/**
* Update single Property in the PropertySet.
*
* @param setHandle
* the PropertySet handle for the company settings
* @param propKey
* property key name
* @param propValue
* property key value
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document updatePropertySet(String setHandle, String propKey, String propValue, S7Config s7Config)
throws IpsApiException;
/**
* Delete PropertySet by the given handle.
*
* @param propertySetHandle
* the PropertySet handle of the company settings
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException .
*/
Document deletePropertySet(String propertySetHandle, S7Config s7Config) throws IpsApiException;
/**
* Gets the property set types associated with the specified company from
* the {@code S7Config} object.
*
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getPropertySetTypes(S7Config s7Config) throws IpsApiException;
/**
* Gets the SPS image presets
*
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getImagePresets(S7Config s7Config) throws IpsApiException;
/**
* Gets the preview or publish server settings based on contextHandle.
* If contextHandle is null, it fetches publish Serving settings by default.
* @param s7Config
* the Scene7 configuration
* @param contextHandle Nullable context handle
* @return a map containing publish server settings like MaxPix, jpegQuality and others
* @throws IpsApiException
*/
Map getImageServerSettings(S7Config s7Config,
@Nullable String contextHandle) throws IpsApiException;
/**
*
* @param s7Config the Scene7 configuration
* @return a List of Publish server context parameters
* @throws IpsApiException
*/
GetPublishContextsReturn getPublishContexts(S7Config s7Config)
throws IpsApiException;
/** Sets the publish or preview Image server settings based on contextHandle
* @param s7Config the Scene7 configuration
* @param contextHandle Nullable context handle
* @param publishSettingArray Array of settings params
* @return Document response
* @throws IpsApiException
*/
Document setImageServerSettings(S7Config s7Config, String contextHandle,
ConfigSettingArray publishSettingArray) throws IpsApiException;
/**
* Gets information about a user.
*
* @param resourceResolver
* resource resolver used to access Scene7 configuration
* @param email
* the user's email
* @param password
* the user's password
* @param region
* the user's region
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getUserInfo(ResourceResolver resourceResolver, String email,
String password, String region) throws IpsApiException;
/**
* Searches for assets based on one or more filter criteria.
*
* @param folder
* the root path for searching for assets; if omitted, the
* company root folder will be used
* @param includeSubfolders
* if true, the search includes sub-folders
* @param published
* if true, the search will include only published assets
* @param assetTypes
* array of Asset Types to include in search
* @param assetSubTypes
* array of sub Asset Types to include in search
* @param responseFields
* a list of fields and subfields for inclusion in the response
* @param excludeFields
* a list of fields and subfields for exclusion from the response
* @param recordsPerPage
* maximum number of results to return
* @param resultsPage
* specifies the page of results to return, based on
* {@code recordsPerPage} page size
* @param fileName
* the filename for search asset
* @param name
* the Scene7 name used for search asset
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document searchAssets(String folder, Boolean includeSubfolders, Boolean published, String[] assetTypes, String[] assetSubTypes,
String[] responseFields, String[] excludeFields,
int recordsPerPage, int resultsPage, String fileName, String name,
S7Config s7Config) throws IpsApiException;
/**
* Searches for assets based on one or more filter criteria.
*
* @param folder
* the root path for searching for assets; if omitted, the
* company root folder will be used
* @param includeSubfolders
* if true, the search includes sub-folders
* @param published
* if true, the search will include only published assets
* @param assetTypes
* array of Asset Types to include in search
* @param assetSubTypes
* array of sub Asset Types to include in search
* @param responseFields
* a list of fields and subfields for inclusion in the response
* @param excludeFields
* a list of fields and subfields for exclusion from the response
* @param recordsPerPage
* maximum number of results to return
* @param resultsPage
* specifies the page of results to return, based on
* {@code recordsPerPage} page size
* @param fileName
* the filename for search asset
* @param name
* the Scene7 name used for search asset
* @param conditionMode
* filename and asset name string comparison operator
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document searchAssets(String folder, Boolean includeSubfolders,
Boolean published, String[] assetTypes, String[] assetSubTypes,
String[] responseFields, String[] excludeFields,
int recordsPerPage, int resultsPage, String fileName, String name,
String conditionMode, S7Config s7Config) throws IpsApiException;
/**
* Searches the metadata index repository for the given search terms.
*
* @param folder
* the root path for searching for assets; if omitted, the
* company root folder will be used
* @param includeSubfolders
* if true, the search includes sub-folders
* @param assetTypes
* array of Asset Types to include in search
* @param assetSubTypes
* array of sub Asset Types to include in search
* @param published
* if true, the search will include only published assets
* @param conditions
* one or more query conditions that define the search criteria
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document searchAssetsByMetadata(String folder, Boolean includeSubfolders, String[] assetTypes, String[] assetSubTypes,
Boolean published,
MetadataCondition[] conditions, S7Config s7Config)
throws IpsApiException;
/**
* Searches the metadata index repository for the given search terms,
* support loading assets by page
*
* @param folder
* the root path for searching for assets; if omitted, the
* company root folder will be used
* @param includeSubfolders
* if true, the search includes sub-folders
* @param assetTypes
* array of Asset Types to include in search
* @param assetSubTypes
* array of sub Asset Types to include in search
* @param published
* if true, the search will include only published assets
* @param conditions
* one or more query conditions that define the search criteria
* @param recordsPerPage
* maximum number of results to return
* @param resultsPage
* specifies the page of results to return, based on
* {@code recordsPerPage} page size
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document searchAssetsByMetadata(String folder, Boolean includeSubfolders, String[] assetTypes,
String[] assetSubTypes, Boolean published, MetadataCondition[] conditions, int recordsPerPage,
int resultsPage, S7Config s7Config) throws IpsApiException;
/**
* Submits a job to the system.
*
* @param jobName
* the name of the job
* @param jobType
* the job's type
* @param s7Config
* the Scene7 configuration
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
* @see Scene7JobType
*/
Document submitJob(String jobName, Scene7JobType jobType, S7Config s7Config)
throws IpsApiException;
/**
* Submit a job to S7 for reprocessing video thumbnail to a specified video frame time
* @param jobName the name of the job
* @param s7Config the Scene7 configuration
* @param assetHandle the asset handle
* @param thumbnailTime the frame time
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document submitReprocessAssetsThumbnailJob(@NotNull final String jobName, @NotNull final S7Config s7Config, final String assetHandle,
final long thumbnailTime) throws IpsApiException;
/**
* Retrieves the system properties XML document served by the GetSystemProperties SPS servlet.
* Deprecated
*
* @param s7Config the Scene7 configuration
* @return the SPS system properties wrapped in a {@link Document}
*/
@Deprecated
Document getSystemProperties(S7Config s7Config);
/**
* Provides a way to get the originally uploaded file to the Scene7 servers
* after an asset has been processed.
*
* @param assetHandle
* the asset's handle (unique id generated by Scene7)
* @param s7Config
* the Scene7 configuration
* @return an InputStream from which the file can be retrieved; the
* InputStream can be null if any error occurred
* @throws IpsApiException
*/
InputStream getOriginalFile(String assetHandle, S7Config s7Config)
throws IpsApiException;
/**
* Provides a way to get the published image for a Scene7 asset
*
* @deprecated Incompatible with Scene7 IPS SSL endpoint. Please use
* getOriginalFile() instead.
*
* @param asset
* the Scene7Asset
asset object
* @param s7Config
* the S7Config
configuration
* @return an InputStream
of the published image asset
*/
@Deprecated
InputStream getIPSFile(Scene7Asset asset, S7Config s7Config);
/**
* Retrieves a JPEG image in an InputStream that can be used as a thumbnail
* in CQ DAM. The image has the same size as the originally uploaded asset
* from Scene7.
*
* @param scene7Asset
* the Scene7 asset abstractization
* @param s7Config
* the Scene7 configuration
* @return an InputStream from which the file can be retrieved; the
* InputStream can be null if any error occurred
* @throws IpsApiException
*/
InputStream getThumbnailForAsset(Scene7Asset scene7Asset, S7Config s7Config)
throws IpsApiException;
/**
* Sets the publish state of a given Scene7 asset
*
* @param assetHandle
* the Scene7 asset handle
* @param publishState
* the asset publish state
* @param s7Config
* the Scene7 configuration
* @return the setAssetPublishState API's response wrapped in a
* {@link Document}
* @throws IpsApiException
*/
Document setAssetPublishState(String assetHandle, S7Config s7Config,
String publishState) throws IpsApiException;
/**
* Sets the publish state of a given Scene7 assets
*
* @param assetHandles
* the Scene7 asset handles
* @param publishState
* the asset publish state
* @param s7Config
* the Scene7 configuration
* @return the setAssetPublishState API's response wrapped in a
* {@link Document}
* @throws IpsApiException
*/
Document setAssetsPublishState(Set assetHandles, S7Config s7Config,
String publishState) throws IpsApiException;
/**
* Create an ImageSet in a given Scene7 folder
*
* @param s7Config
* the Scene7 configuration
* @param folderHandle
* Scene7 folder handle
* @param name
* name of image set
* @param type
* type of new image set
* @param thumbAssetHandle
* (optional) asset handle of user-defined thumbnail image for
* set
* @return the IPS API response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document createImageSet(S7Config s7Config, String folderHandle, String name, String type,
@Nullable String thumbAssetHandle) throws IpsApiException;
/**
* Update an ImageSet
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle of the image set to update
* @param members
* (optional) list of imageset members (Null = not updated)
* @param thumbAssetHandle
* (optional) asset handle of user-defined thumbnail image for
* set (Null = not updated)
* @return the IPS API response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document updateImageSet(S7Config s7Config, String assetHandle,
@Nullable ImageSetMemberUpdateArray members,
@Nullable String thumbAssetHandle) throws IpsApiException;
/**
* Update an image set of a given a list of Scene7 assets
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle of the image set member array
* @param setMemberHandles
* list of image set member handles
* @param thumbAssetHandle
* optional parameter to define set thumbnail
* @return the updateImageSet API's response wrapped in a {@link Document}
* @deprecated Use {@link #updateImageSet(S7Config, String, ImageSetMemberUpdateArray, String)}
*/
@Deprecated
Document updateImageSet(S7Config s7Config, String assetHandle,
List setMemberHandles, String thumbAssetHandle);
/**
* Get members of legacy ImageSet
*
* @param s7Config the Scene7 configuration
* @param assetHandle asset handle of the ImageSet
* @return the IPS API response as XML {@link Document}
* @throws IpsApiException on error at IPS
*/
Document getImageSetMembers(S7Config s7Config, String assetHandle) throws IpsApiException;
/**
* Create an AssetSet in a given Scene7 folder
*
* @param s7Config
* the Scene7 configuration
* @param folderHandle
* Scene7 folder handle
* @param name
* name for new asset set
* @param type
* type of new asset set
* @param setDefinition
* (optional) set's definition string
* @param thumbAssetHandle
* (optional) asset handle of user-defined thumbnail image for
* set
* @return the IPS API response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document createAssetSet(S7Config s7Config, String folderHandle, String name, String type,
@Nullable String setDefinition,
@Nullable String thumbAssetHandle) throws IpsApiException;
/**
* Update an AssetSet
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle of the asset set to update
* @param setDefinition
* (optional) set's definition string (Null = not updated)
* @param thumbAssetHandle
* (optional) asset handle of user-defined thumbnail image for
* set (Null = not updated)
* @return the IPS API response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document updateAssetSet(S7Config s7Config, String assetHandle,
@Nullable String setDefinition, @Nullable String thumbAssetHandle)
throws IpsApiException;
/**
* Create a folder on Scene7 server
*
* @param folderPath
* The root folder used to retrieve folders and all subfolders to
* the leaf level. If excluded, the company root is used.
* @param s7Config
* the Scene7 configuration
* @return the createFolder API response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document createFolder(String folderPath, S7Config s7Config)
throws IpsApiException;
/**
* Create a preset view to determine what users can see.
*
* The preset view is applied when assets are published.
*
* @param s7Config
* the Scene7 configuration
* @param folderHandle
* folder handle of the folder that contains the assets
* @param name
* name for the viewer
* @param type
* type of any available viewer in IPS
* @param configSettings
* list containing {@link Scene7ConfigSetting} values to apply
* @return the createViewerPreset API response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document createViewerPreset(
S7Config s7Config,
String folderHandle,
String name,
String type,
@Nullable List configSettings)
throws IpsApiException;
/**
* Get all viewer configuration for the specified asset
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle for the asset in question
* @return the getViewerConfigSettings API response wrapped in a
* {@link Document}
* @throws IpsApiException
*/
Document getViewerConfigSettings(S7Config s7Config, String assetHandle)
throws IpsApiException;
/**
* Attach viewer configuration settings to an asset
*
* Can be viewer preset or the source asset for the viewer
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle for the asset to modify
* @param name
* name of the asset
* @param type
* type of any available viewer in IPS
* @param configSettings
* list containing {@link Scene7ConfigSetting} values to apply
* @throws IpsApiException
*/
Document setViewerConfigSettings(
S7Config s7Config,
String assetHandle,
String name,
String type,
List configSettings)
throws IpsApiException;
/**
* Updates SWF viewer configuration settings.
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle for the asset to modify
* @param configSettings
* list containing {@link Scene7ConfigSetting} values to apply
* @throws IpsApiException
*/
Document updateViewerConfigSettings(S7Config s7Config, String assetHandle,
List configSettings) throws IpsApiException;
/**
* Creates a user account and adds the account to one of more companies
*
* @param s7Config
* The Scene7 configuration
* @param firstName
* The user's first name
* @param lastName
* The user's last name
* @param email
* The user's email address
* @param defaultRole
* The role for the user in the companies they belong, the
* IpsAdmin role overrides other per-company settings
* @param password
* The user's password
* @param passwordExpires
* The instant of expiration of the password
* @param isValid
* Determine the user's validity
* @param membershipArray
* List of {@link Scene7CompanyMembership} entries enumerating
* the membership of the user.
* @return
* @throws IpsApiException
*/
Document addUser(
S7Config s7Config,
String firstName,
String lastName,
String email,
String defaultRole,
String password,
@Nullable Instant passwordExpires,
boolean isValid,
List membershipArray)
throws IpsApiException;
/**
* Return all users
*
* @param s7Config
* The Scene7 configuration
* @param includeInvalid
* Indicate if invalid users should be included
* @return
* @throws IpsApiException
*/
Document getAllUsers(@NotNull S7Config s7Config, boolean includeInvalid)
throws IpsApiException;
/**
* Return a optionally sorted, filtered and paged list user results
*
* @param s7Config
* The Scene7 config
* @param includeInactive
* Include or exclude inactive members
* @param includeInvalid
* Include or exclude invalid members
* @param companyHandleArray
* Filter results be company
* @param groupHandleArray
* Filter results by group
* @param userRoleArray
* Filter results by user role
* @param charFilterField
* Filter results by field's string prefix
* @param charFilter
* Filter results by a specific character
* @param sortBy
* Choice of user sort field
* @param recordsPerPage
* Number of records per page to return
* @param resultsPage
* The desired result page
* @return
* @throws IpsApiException
*/
Document getUsers(
@NotNull S7Config s7Config,
@Nullable Boolean includeInactive,
@Nullable Boolean includeInvalid,
@Nullable List companyHandleArray,
@Nullable List groupHandleArray,
@Nullable List userRoleArray,
@Nullable String charFilterField,
@Nullable String charFilter,
@Nullable String sortBy,
@Nullable Integer recordsPerPage,
@Nullable Integer resultsPage) throws IpsApiException;
/**
* Set user attributes
*
* @param s7Config
* The Scene7 config
* @param userHandle
* User handle
* @param firstName
* First name
* @param lastName
* Last name
* @param email
* Email address
* @param defaultRole
* The role for the user in the companies they belong, the
* IpsAdmin role overrides other per-company settings
* @param passwordExpires
* The password's expiration
* @param isValid
* Determine if valid IPS user
* @param membershipArray
* List of {@link Scene7CompanyMembership} entries enumerating
* the membership of the user
* @return
* @throws IpsApiException
*/
Document setUserInfo(
S7Config s7Config,
@Nullable String userHandle,
String firstName,
String lastName,
String email,
String defaultRole,
@Nullable Instant passwordExpires,
boolean isValid,
List membershipArray)
throws IpsApiException;
/**
* Deletes an image format.
*
* @param s7Config
* The Scene7 config
* @param imageFormatHandle
* Handle to the image format. (This is returned from
* {@link #saveImageFormat(S7Config, String, String, String)})
* @throws IpsApiException
*/
Document deleteImageFormat(S7Config s7Config, String imageFormatHandle)
throws IpsApiException;
/**
* Returns image formats, such as PDF, EPS, SWF, and others.
*
* @param s7Config
* The Scene7 config
* @return
* @throws IpsApiException
*/
Document getImageFormats(@NotNull S7Config s7Config) throws IpsApiException;
/**
* Create an image format.
*
* @param s7Config
* The Scene7 config
* @param imageFormatHandle
* Image format handle you want to save
* @param name
* Image format name
* @param urlModifier
* IPS protocol query string
*
* @return
* @throws IpsApiException
*/
Document saveImageFormat(S7Config s7Config,
@Nullable String imageFormatHandle, String name, String urlModifier)
throws IpsApiException;
/**
* Deletes an image map
*
* @param s7Config
* The Scene7 config
* @param imageMapHandle
* Handle for the image map to delete
* @throws IpsApiException
*/
Document deleteImageMap(S7Config s7Config, String imageMapHandle)
throws IpsApiException;
/**
* Create a new image map or edit an existing map
*
* @param s7Config
* The Scene7 config
* @param assetHandle
* Handle to the asset the map belongs to
* @param imageMapHandle
* Handle to the image map.
* @param name
* Name of the image map
* @param shapeType
* Choice of region shape
* @param region
* A comma delimited list of points that define the region
* @param action
* The href value associated with the image map
* @param position
* The order in the list of image maps
* @param enabled
* Whether this map is enabled
* @return
* @throws IpsApiException
*/
Document saveImageMap(
S7Config s7Config,
String assetHandle,
@Nullable String imageMapHandle,
String name,
String shapeType,
String region,
String action,
int position,
boolean enabled)
throws IpsApiException;
/**
* Sets the image map for an asset.
*
* You must have already created the image maps. Image maps are applied in
* order of retrieval from the array. This means the second image map
* overlays the first, the third overlays the second, and so on.
*
* @param s7Config
* The Scene7 config
* @param assetHandle
* Handle to the asset
* @param imageMap
* list of predefined {@link Scene7ImageMapDefinition} objects
* @return
* @throws IpsApiException
*/
Document setImageMaps(S7Config s7Config, String assetHandle,
List imageMap) throws IpsApiException;
/**
* Sets asset metadata using batch mode.
*
* @param s7Config
* The Scene7 config
* @param updates
* List of {@link Scene7ImageFieldUpdate} to be applied
* @throws IpsApiException
*/
Document batchSetAssetMetadata(
S7Config s7Config,
List updates) throws IpsApiException;
/**
* Sets asset metadata using batch mode.
*
* @param s7Config
* The Scene7 config
* @param updates
* List of {@link Scene7ImageFieldUpdate} to be applied
* @throws IpsApiException
*/
Document batchSetImageFields(
S7Config s7Config,
List updates) throws IpsApiException;
/**
* Retrieves Scene7 upload job processing status
*
* @param jobHandle
* Scene7 upload job handle
* @param s7Config
* Scene7 configuration object
* @return the API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document getUploadTaskStatus(String jobHandle, S7Config s7Config)
throws IpsApiException;
/**
* Moves an asset to a specific folder
*
* @param s7Config
* Scene7 configuration object
* @param assetHandle
* Handle to the asset you want to move
* @param folderHandle
* Handle to the destination folder
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document moveAsset(S7Config s7Config, String assetHandle,
String folderHandle) throws IpsApiException;
/**
* Moves multiple assets independently of each other. It accomplishes this
* using the AssetMove type contained in the assetMoveArray. Each AssetMove
* field contains a destination folder
*
* @param s7Config
* Scene7 configuration object
* @param assetFolderHandles
* Map that contains an asset and an asset destination folder
*
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document moveAssets(S7Config s7Config,
Map assetFolderHandles) throws IpsApiException;
/**
* Move a folder to a new location
*
* @param s7Config
* Scene7 configuration object
* @param sourceFolderHandle
* Handle to the source folder
* @param destinationFolderHandle
* Handle to the destination folder
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document moveFolder(S7Config s7Config, String sourceFolderHandle,
String destinationFolderHandle) throws IpsApiException;
/**
* Rename an asset
*
* @param s7Config
* Scene7 configuration object
* @param assetHandle
* Handle to the asset you want to move
* @param name
* Asset's new name
* @param validateName
* If the validateName is true and the asset type requires a
* unique IPS ID, then the new name is checked for global
* uniqueness and renameAsset throws a fault if it is not unique.
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document renameAsset(S7Config s7Config, String assetHandle, String name,
boolean validateName) throws IpsApiException;
/**
* Rename a folder
*
* @param s7Config
* Scene7 configuration object
* @param folderHandle
* Handle to the folder
* @param folderName
* New folder name
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document renameFolder(S7Config s7Config, String folderHandle,
String folderName) throws IpsApiException;
/**
* Tries to rename given asset to the new name, maintaining the requirement of
* no duplicate asset names can exists in S7 for a given Company.
* If attempt was failed, it tries adding "-1" to the name, and repeat, then "-2" and so on.
* The maximum attempts count is 100.
* Note: when name ends with "-AVS" suffix - numbers are added before "-AVS" (S7 requirement).
*
* @param s7Config configuration for access to Scene7 server
* @param assetHandle asset handle (ID)
* @param newDesiredName new asset name, case-sensitive
* @return actual name which was able to rename
* @throws IpsApiException on error at Scene7
*/
String renameAssetToUniqueName(S7Config s7Config, String assetHandle, String newDesiredName) throws IpsApiException;
/**
* Sets the thumbnail image for one or more assets
*
* @param s7Config
* Scene7 configuration object
* @param thumbnailUpdates
* List of {@link ThumbAssetUpdate} objects to be applied
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException
* in case of IPS failures or undefined data format
*/
Document batchSetThumbAsset(S7Config s7Config,
List thumbnailUpdates) throws IpsApiException;
/**
* Update one or more Smart Crop sub asset(s)
*
* @param s7Config
* Scene7 configuration object
* @param updateArray
* Array of SmartCropUpdate objects containing subasset handles
* and NormalizedCropRect values
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document updateSmartCrops(S7Config s7Config,
SmartCropUpdateArray updateArray) throws IpsApiException;
/**
* Add one or more Smart Crop sub asset(s)
*
* @param s7Config
* Scene7 configuration object
* @param assetHandle
* asset handle for asset to which smart crops are to be added
* @param addSmartCropArray
* Array of SmartCropUpdate objects NormalizedCropRect values and their respective names
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException
*/
Document addSmartCrops(S7Config s7Config, String assetHandle,
SmartCropAddArray addSmartCropArray) throws IpsApiException;
/**
* Retrieves an XMP Metadata packet for the specified asset.
* @param s7Config
* Scene7 configuration object
* @param assetHandle
* Handle to the asset you want to get XMP metadata
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException
* in case of IPS failures or undefined data format
*/
Document getXMPPacket(S7Config s7Config, String assetHandle) throws IpsApiException;
/**
* Sets or updates an XMP metadata packet for an asset.
* @param s7Config
* Scene7 configuration object
* @param assetHandle
* Handle to the asset you want to update XMP metadata
* @param xmpPacketAsXml
* updated XMP packet to update asset metadata in XML format
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException
* in case of IPS failures or undefined data format
*/
Document updateXMPPacket(S7Config s7Config, String assetHandle, String xmpPacketAsXml) throws IpsApiException;
/**
* Checks the login in Scene7 with the given credentials
* @param resourceResolver
* Resource Resolver used to access Scene7 configuration
* @param email
* Email id of the user
* @param password
* Password of the user
* @param region
* the user's region
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException in case of IPS failures or undefined data format
*/
Document getLoginStatus(ResourceResolver resourceResolver,String email, String password, String region) throws IpsApiException;
/**
* Sets the password for a given user with an expiry of 100 years ahead from the current date.
* @param s7Config
* Scene7 configuration object
* @param userHandle
* Scene7 User Handle of the user
* @param password
* Password to be set
* @param validityInDays Password validity in number of days. Set it to null/zero for default expiry of 100 years.
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException in case of IPS failures or undefined data format
*/
Document setPassword(S7Config s7Config,String userHandle, String password,@Nullable Integer validityInDays) throws IpsApiException;
/**
* Forwards the supplied list of URLs to the Scene7 CDN (Content Distribution Network) provider
* to invalidate their existing cache of HTTP responses
* @param s7Config Scene7 configuration object
* @param urlArray List of up to 1000 URLs to invalidate from the CDN cache
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException in case of IPS failures or undefined data format
*/
Document cdnCacheInvalidation(S7Config s7Config, UrlArray urlArray) throws IpsApiException;
/**
* Forwards the supplied list of companyAliasURLs to the Scene7 CDN (Content Distribution Network) provider
* to invalidate their existing cache of HTTP responses.
* @param s7Config Scene7 configuration object
* @param companyAliasUrlArray List of up to 1000 URLs to invalidate from the CDN cache
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException in case of IPS failures or undefined data format
*/
Document flushCdnCacheByAlias(S7Config s7Config, UrlArray companyAliasUrlArray) throws IpsApiException;
/**
* Gets the token for a given company to access secure preview IS.
* @param s7Config
* Scene7 configuration object
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException in case of IPS failures or undefined data format
*/
Document getSecureISAuthToken(S7Config s7Config) throws IpsApiException;
/**
* Deletes the sub assets for an asset
* @param companyHandle the scene7 handle to the company
* @param assetHandle the scene7 handle to the main asset
* @param subAssetHandles list of scene7 handles to the sub assets
* @param s7Config the scene7 configuration
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException in case of IPS failures or undefined data format
*/
Document deleteSubAssets(String companyHandle,String assetHandle, List subAssetHandles, S7Config s7Config)
throws IpsApiException;
/**
* @param param set company setting request
* @param s7Config the scene7 configuration
* @return The API's response wrapped in a {@link Document}
* @throws IpsApiException in case of IPS failures or undefined data format
*/
Document setCompanySettings(SetCompanySettingsParam param,
S7Config s7Config) throws IpsApiException;
/**
* @param s7Config the scene7 configuration
* @return response of company settings params
* @throws IpsApiException in case of IPS failures or undefined data format
*/
GetCompanySettingsReturn getCompanySettings(S7Config s7Config)
throws IpsApiException;
/**
* Get the CompanyInfo given any one of the mandatory parameter - companyName or companyHandle
* @param companyName the name of the company
* @param companyHanlde the handle of the company
* @param s7Config the scene7 configuration
*
* @return response of company info params
* @throws IpsApiException in case of IPS failures or undefined data format
*/
GetCompanyInfoReturn getCompanyInfo(String companyName, String companyHanlde, S7Config s7Config) throws IpsApiException;
/**
* check wether PrePackagedStreaming is enabled for the given video.
* PrePackagedStreaming refers to streaming of video using fragmented mp4 and byte range headers
* @param avsFileName The avsHandle for given video
* @param previewServer preview Server url
* @param previewServerToken preview Server token
* @return
*/
boolean isPrepackagedStreamingEnabled(String avsFileName, String previewServer, String previewServerToken) throws IOException;
/**
* reprocess asset from DM with provided job options
* @param resolver resolver with correct permission to fetch node data
* @param s7Config the scene7 configuration
* @param batchId batch id
* @param assets list of asset
* @param jobOptions parameter based on that asset need to be reprocessed
* @return
*/
List reprocessAssets(ResourceResolver resolver, S7Config s7Config, String batchId, Iterable assets, String jobOptions) throws Exception;
/**
* Update audio and captions metadata
* @param audioCaptionsMetadata set of audio captions metadata
* @param s7Config the scene7 configuration
* @return document result
* @throws IpsApiException in case of IPS failures or undefined data format
*/
Document updateAudioCaptionsMetadata(Map> audioCaptionsMetadata, S7Config s7Config) throws IpsApiException;
/**
* Set URL modifier for the template asset
* @param assetHandle the scene7 handle to the template asset
* @param s7Config the scene7 configuration
* @return document result
* @throws IpsApiException in case of IPS failures or undefined data format
*/
Document setUrlModifier(String assetHandle, String modifier, S7Config s7Config) throws IpsApiException;
/**
* Create a template asset
* @param templateProperties the template properties
* @param s7Config the scene7 configuration
* @return document result
* @throws IllegalArgumentException in case of invalid arguments
* @throws IpsApiException in case of IPS failures or undefined data format
*/
Document createTemplate(Map templateProperties, S7Config s7Config) throws IllegalArgumentException, IpsApiException;
/**
* Transcribe or Translate asset from DM with provided job options
* @param s7Config the scene7 configuration
* @param sourceLanguage source language
* @param sourceFileAssetHandle source file asset handle
* @param targetLanguage target language
* @param videoAssetHandle video asset handle
* @return
*/
String transcribeTranslateAsset(S7Config s7Config, String sourceLanguage, String sourceFileAssetHandle, LanguageFileNameMappingArray targetLanguage, String videoAssetHandle) throws Exception;
/**
* Return video AVS content
* @param avsFileName avs name
* @param previewServer preview server url
* @param previewServerToken preview server token
* @return document result
* @throws URISyntaxException
* @throws IOException
*/
String getAVSContent(String avsFileName, String previewServer, String previewServerToken) throws URISyntaxException, IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy