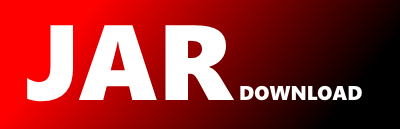
com.day.cq.mcm.api.MCMFacade Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.mcm.api;
import java.util.Collection;
import java.util.Iterator;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
/**
* This is the central entry point for accessing plugins and configuration info
* on MCM.
* Provides accessors to the known component types, too.
*
*/
public interface MCMFacade {
/**
* Get a plugin for a resource found using component types returned by {@link MCMFacade}.
* Returns null
if the resource's type isn't known.
* @param r
* @return
*/
public MCMPlugin getPlugin(Resource r);
/**
* Get the complete list of registered plugins.
* @return
*/
public Collection getPlugins();
/**
* Get a plugin if the type is known to the {@link MCMFacade}. Otherwise
* null
is returned.
* @param componentTypeToProvideFrom
* @return
*/
MCMPlugin getPluginForType(String componentTypeToProvideFrom);
/**
* Gets the type of a given sling:resourceType
.
* @param resourceTypeToTest
*/
public MCMResourceType getMCMType(String resourceTypeToTest);
/**
* The component types known to be used for {@link Experience} components.
* Might contain indirections, too.
* @return
*/
public Collection getRegisteredExperienceComponents();
/**
* The component types known to be used for {@link Touchpoint} components.
* Might contain indirections, too.
*
* @return
*/
public Collection getRegisteredTouchpointComponents();
/**
* Finds all posts that are related to the given account,
* in campaigns and under account.
* @param rr
* @param t
* @return
*/
public Iterator findExperiences(ResourceResolver rr, Touchpoint t);
/**
* Find all social feed resources underneath a given path.
*
* @param rootComponents
* @param path
* @param additionalPropertyCondition can be null
, or a additional condition
* to be added to the property checking.
* @return
*/
public Iterator findResources(ResourceResolver rr, Collection rootComponents,
String path, String additionalPropertyCondition);
/**
* Change configuration by adding an indirection. No checking done
* for the existence of the toCompType.
* @param fromCompType
* @param toCompType
*/
public void addExperienceIndirection(String fromCompType, String toCompType);
/**
* Change configuration by adding an indirection. No checking done
* for the existence of the toCompType.
* @param fromCompType
* @param toCompType
*/
public void addTouchpointIndirection(String fromCompType, String toCompType);
/**
* Change configuration by removing an indirection. If the indirection
* is inexistent or if the fromCompType is not an indirection nothing is done.
*
* @param fromCompType
*/
public void removeExperienceIndirection(String fromCompType);
/**
* Change configuration by removing an indirection. If the indirection
* is inexistent or if the fromCompType is not an indirection nothing is done.
*
* @param fromCompType
*/
public void removeTouchpointIndirection(String fromCompType);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy