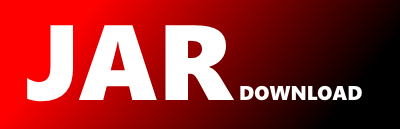
com.day.cq.rewriter.htmlparser.SAXWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.rewriter.htmlparser;
import java.io.IOException;
import java.io.PrintWriter;
import org.apache.felix.scr.annotations.Component;
import org.xml.sax.Attributes;
import org.xml.sax.Locator;
import org.xml.sax.SAXException;
import com.day.cq.rewriter.htmlparser.impl.HtmlParserTransformer;
import com.day.cq.rewriter.pipeline.Serializer;
import com.day.cq.rewriter.processor.ProcessingComponentConfiguration;
import com.day.cq.rewriter.processor.ProcessingContext;
/**
* Writer passed on to other Sling components. Removes or marks bad links
* in HTML data.
* @deprecated Use the Apache Sling Html Serializer instead.
*/
@Component(factory="com.day.cq.rewriter.pipeline.Serializer/htmlwriter")
@Deprecated
public class SAXWriter implements Serializer {
private PrintWriter delegatee;
/**
* @see com.day.cq.rewriter.pipeline.Serializer#init(com.day.cq.rewriter.processor.ProcessingContext, com.day.cq.rewriter.processor.ProcessingComponentConfiguration)
*/
public void init(ProcessingContext pipelineContext, ProcessingComponentConfiguration config)
throws IOException {
final PrintWriter writer = pipelineContext.getWriter();
if (writer == null) {
throw new IllegalArgumentException("Writer must not be null");
}
this.delegatee = writer;
}
/**
* @see org.xml.sax.ContentHandler#endDocument()
*/
public void endDocument() throws SAXException {
this.delegatee.flush();
}
/**
* @see org.xml.sax.ContentHandler#startElement(java.lang.String, java.lang.String, java.lang.String, org.xml.sax.Attributes)
*/
public void startElement(String uri, String localName, String name,
Attributes atts) throws SAXException {
boolean endSlash = false;
this.delegatee.write('<');
this.delegatee.write(localName);
final String quotesString = atts.getValue(DocumentHandlerToSAXAdapter.NAMESPACE, DocumentHandlerToSAXAdapter.QUOTES_ATTR);
for(int i=0; i i ) {
quoteChar = quotesString.charAt(i);
} else {
quoteChar = '\"';
}
this.delegatee.write(quoteChar);
this.delegatee.write(value);
this.delegatee.write(quoteChar);
}
}
}
if (endSlash) {
// XHTML
this.delegatee.write("/");
}
this.delegatee.write(">");
}
/**
* @see org.xml.sax.ContentHandler#endElement(java.lang.String, java.lang.String, java.lang.String)
*/
public void endElement(String uri, String localName, String name)
throws SAXException {
if (!HtmlParserTransformer.DEFAULT_EMPTY_TAGS.contains(localName)) {
this.delegatee.write("");
this.delegatee.write(localName);
this.delegatee.write('>');
}
}
/**
* Called by HtmlParser if character data and tags are to be output for which no
* special handling is necessary.
*
* @param buffer Character data
* @param offset Offset where character data starts
* @param length The length of the character data
*/
public void characters(char[] buffer, int offset, int length)
throws SAXException {
// special hack for flush request, see bug #20068
if (length == 0) {
this.delegatee.flush();
} else {
this.delegatee.write(buffer, offset, length);
}
}
/**
* @see org.xml.sax.ContentHandler#endPrefixMapping(java.lang.String)
*/
public void endPrefixMapping(String prefix) throws SAXException {
// not used atm
}
/**
* @see org.xml.sax.ContentHandler#ignorableWhitespace(char[], int, int)
*/
public void ignorableWhitespace(char[] ch, int start, int length)
throws SAXException {
// not used atm
}
/**
* @see org.xml.sax.ContentHandler#processingInstruction(java.lang.String, java.lang.String)
*/
public void processingInstruction(String target, String data)
throws SAXException {
// not used atm
}
/**
* @see org.xml.sax.ContentHandler#setDocumentLocator(org.xml.sax.Locator)
*/
public void setDocumentLocator(Locator locator) {
// not used atm
}
/**
* @see org.xml.sax.ContentHandler#skippedEntity(java.lang.String)
*/
public void skippedEntity(String name) throws SAXException {
// not used atm
}
/**
* @see org.xml.sax.ContentHandler#startDocument()
*/
public void startDocument() throws SAXException {
// not used atm
}
/**
* @see org.xml.sax.ContentHandler#startPrefixMapping(java.lang.String, java.lang.String)
*/
public void startPrefixMapping(String prefix, String uri)
throws SAXException {
// not used atm
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy