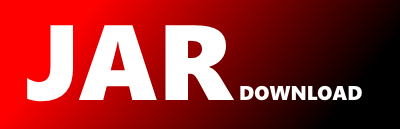
com.day.cq.search.suggest.Suggester Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* Copyright 1997-2010 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.search.suggest;
import javax.jcr.Session;
import com.day.cq.search.suggest.builder.SearchIndexSuggestionExtractor;
import aQute.bnd.annotation.ProviderType;
/**
* A service interface for retrieving search suggestions and spell checking.
* Uses a pre-built {@link SuggestionIndex index} to look up the suggestions.
* The spell check is done by leveraging the JCR full text search index.
*
*
* The index can be created in various ways: by analysing most frequently used
* terms in the full text index (see {@link SearchIndexSuggestionExtractor}), by
* tracking actual search statistics (see {@link QueryTracker}, requires
* configuration to synchronize with multiple publish instances) or by a custom
* index creator (use {@link SuggestionIndexManager} to create indexes).
*
*
* Given that the indexes won't change very often, they should be created in
* the background, and they can be distributed to publish instances via
* content packages.
*/
@ProviderType
public interface Suggester {
/**
* Returns suggestions for a given term, which can be any partial word of at
* least one character. Depending on the given context path, different
* suggestion indexes can be addressed.
*
* @param session
* user session to access index
* @param indexName
* a path or name for the suggestion index
* @param term
* partial word to look up suggestions for
* @param spellCheck
* if true
, the term will be spell checked if
* nothing was found and the suggestion look up will be retried
* with the spell check result
*
* @return a list of search suggestions or an empty array if none could
* be found
*/
String[] getSuggestions(Session session, String indexName, String term, boolean spellCheck);
/**
* Uses the JCR search index to run a spellcheck for the given word. Uses
* the custom rep:spellcheck()
Xpath function. Will return a
* single spell checked term or null
if the term is already
* correctly spelled or if no match was found.
*
* @param session
* user session to run spellcheck on repository index(es)
* @param term
* the term to spell check
* @return a correctly spelled term or null
*/
String spellCheck(Session session, String term);
/**
* Returns suggestions for a given term, which can be any partial word of at
* least one character. Depending on the given context path, different
* suggestion indexes can be addressed.
*
* @param indexName
* a path or name for the suggestion index
* @param term
* partial word to look up suggestions for
* @param spellCheck
* if true
, the term will be spell checked if
* nothing was found and the suggestion look up will be retried
* with the spell check result
*
* @return a list of search suggestions or an empty array if none could
* be found
*
* @deprecated use the variant with a user session argument: {@link #getSuggestions(Session, String, String, boolean)}
*/
@Deprecated
String[] getSuggestions(String indexName, String term, boolean spellCheck);
/**
* Uses the JCR search index to run a spellcheck for the given word. Uses
* the custom rep:spellcheck()
Xpath function. Will return a
* single spell checked term or null
if the term is already
* correctly spelled or if no match was found.
*
* @param term
* the term to spell check
* @return a correctly spelled term or null
*
* @deprecated use the variant with a user session argument: {@link #spellCheck(Session, String)}
*/
@Deprecated
String spellCheck(String term);
}