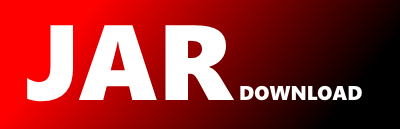
com.day.cq.statistics.util.RequestHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.statistics.util;
import javax.servlet.http.HttpServletRequest;
import java.text.SimpleDateFormat;
import java.text.ParseException;
import java.io.UnsupportedEncodingException;
/**
* RequestHelper
provides utility methods for request handling.
*/
public class RequestHelper {
/**
* Returns the long parameter with name paramName
. This method
* returns the defaultValue
if the parameter value is malformed
* or missing.
*
* @param request the request.
* @param paramName the parameter name.
* @param defaultValue a default value.
* @return the parameter value as a long or the default if missing or
* malformed.
*/
public static long getLongParameter(HttpServletRequest request,
String paramName,
long defaultValue) {
long value = defaultValue;
String s = request.getParameter(paramName);
if (s != null) {
try {
value = Long.parseLong(s);
} catch (NumberFormatException e) {
// ignore
}
}
return value;
}
/**
* Returns the date parameter with name paramName
. This method
* returns the defaultValue
if the parameter value is malformed
* or missing.
*
* The expected date pattern is: 'yyyyMMdd'.
*
* @param request the request.
* @param paramName the parameter name.
* @param defaultValue a default value.
* @return the parameter value as a long date or the default if missing or
* malformed.
*/
public static long getDateParameter(HttpServletRequest request,
String paramName,
long defaultValue) {
long value = defaultValue;
String s = request.getParameter(paramName);
if (s != null) {
try {
SimpleDateFormat format = new SimpleDateFormat("yyyyMMdd");
value = format.parse(s).getTime();
} catch (ParseException e) {
// ignore
}
}
return value;
}
/**
* Returns the String parameter with name paramName
. This
* method returns the defaultValue
if the parameter value is
* missing.
*
* @param request the request.
* @param paramName the parameter name.
* @param defaultValue a default value.
* @return the parameter value or the default if missing.
*/
public static String getStringParameter(HttpServletRequest request,
String paramName,
String defaultValue) {
String value = defaultValue;
String s = request.getParameter(paramName);
if (s != null) {
try {
value = new String(s.getBytes("ISO-8859-1"), "UTF-8");
} catch (UnsupportedEncodingException e) {
// will never happen
}
}
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy