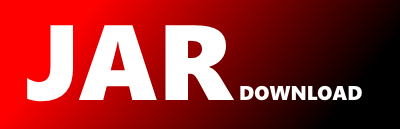
com.day.cq.wcm.api.components.Component Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.api.components;
import java.util.Collection;
import java.util.Map;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ValueMap;
import org.apache.sling.api.adapter.Adaptable;
import com.day.cq.commons.LabeledResource;
/**
* Defines a CQ5 Component.
*
* Note: for performance reasons, most of the component data is provided using
* the underlying system component. in order to check whether a user has
* access to a component, {@link #isAccessible()} should be called.
*/
public interface Component extends Adaptable, LabeledResource {
/**
* Checks if this component is accessible, i.e. if the current session can
* access the underlying resource.
* @return true
if the component is accessible.
*
* @since 5.4
*/
boolean isAccessible();
/**
* Returns the cell name of this component.
*
* If the cell name is an empty string is returned, the wcm request filter
* will not create a new edit context.
*
* @return the cell name
*/
String getCellName();
/**
* Checks if this component is editable. This is the case if it defines or
* inherits a dialog
* @return true
if the component is editable.
*/
boolean isEditable();
/**
* Checks if this component is designable. This is the case if it defines or
* inherits a design dialog
* @return true
if the component is designable.
*/
boolean isDesignable();
/**
* Checks if this component is a container component. For example a
* paragraph system component. Container components affect the cell name
* generation of included resources where the cell name of the respective
* component is used instead of the name of the addressed resource.
*
* @return true
if this component is a container.
*/
boolean isContainer();
/**
* Checks if this component is analyzable. This is
* the case if it defines an analytics node.
*
* @return true
if the component is analyzable.
*/
boolean isAnalyzable();
/**
* Checks if this component does not need decoration when included.
* @return true
if this component does not need decoration
* when included.
*/
boolean noDecoration();
/**
* Returns the dialog path of this component or of an inherited one.
* Please note, that inheriting a dialog is not always desirable, since
* (currently) the resource type is defined as hidden field in the
* dialog. so extending a component without defining an own dialog would
* result in content having the base component's resource type.
* @return the dialog path or null
*/
String getDialogPath();
/**
* Returns the design dialog path of this component or of an inherited one.
* @return the design dialog path or null
*/
String getDesignDialogPath();
/**
* Returns the path to an icon for this component or null
if
* the component does not provide an icon.
* If this component does not define a path the one of the super component
* is returned.
* Note: the path is a webapp relative URL. eg: /docroot/c1.gif
* @return the path to an icon or null
*/
String getIconPath();
/**
* Returns the path to a thumbnail for this component or null
* if the component does not provide a thumbnail.
* If this component does not define a path the one of the super component
* is returned.
* Note: the path is a webapp relative URL. eg: /docroot/c1.gif
* @return the path to a thumbnail or null
*/
String getThumbnailPath();
/**
* Returns the name of the component group. This is merely informational
* for dialogs that want to group the components.
* @return the name of the component group or null
if not defined.
*/
String getComponentGroup();
/**
* Returns the {@link ValueMap properties} of
* this Component.
*
* @return the component properties
*/
ValueMap getProperties();
/**
* Returns the edit config of this component
* @return the edit config or null
if not available
*/
ComponentEditConfig getDeclaredEditConfig();
/**
* Returns the child edit config of this component
* @return the child edit config or null
if not available
*/
ComponentEditConfig getDeclaredChildEditConfig();
/**
* Returns the edit config of this component or the one of it's super
* component.
* @return the edit config or null
if not available
*/
ComponentEditConfig getEditConfig();
/**
* Returns the child edit config of this component or the one of it's
* super component.
* @return the edit config or null
if not available
*/
ComponentEditConfig getChildEditConfig();
/**
* Returns the design edit config for the given cell name
* @param cellName name of the cell
* @return the design edit config
*/
ComponentEditConfig getDesignEditConfig(String cellName);
/**
* Returns additional tag attributes that are added to the surrounding
* html tag.
*
* @return map of additional tag attributes.
*/
Map getHtmlTagAttributes();
/**
* Returns the super component if the super resource type is defined and
* points to a valid component.
* @return the super component or null
*/
Component getSuperComponent();
/**
* Returns the resource type to be used for this component. this is usually
* the path of this component without the first path segment, but can be
* overridden by setting the "sling:resourceType".
*
* @return the resource type
*/
String getResourceType();
/**
* Finds the resource with the given name that is stored "in" this component.
* If the resource cannot be found, the super component is asked.
*
* This is usually used in image scripts that need to load additional images.
*
* @param name name of the resource
* @return resource or null
*/
Resource getLocalResource(String name);
/**
* Returns a collection of virtual components that are configured for this
* component.
*
* @return a collection of virtual components.
*/
Collection getVirtualComponents();
/**
* Returns the default view for this component
* @return the default view or null
is not defined.
*/
String getDefaultView();
/**
* Returns the path to the template for the component's content.
*
* @return path to the template for the component's content.
* null
if not defined
*/
String getTemplatePath();
/**
* Returns a collection of info provider names that are configured for this
* page-level component.
* @return the provider names
*/
String[] getInfoProviders();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy