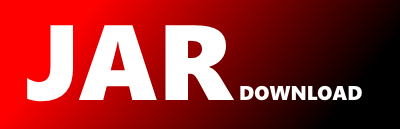
com.day.cq.wcm.api.components.EditConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.api.components;
import java.util.Map;
import com.day.cq.commons.JSONItem;
/**
* Defines the edit control configuration. A JSON serialized form needs to be
* written to the response when drawing the 'edit control'.
* Note that modifications to the config are never persisted and
* applied to the final, merged config. Be aware when modifying a parent
* config that the changes can propagate to the successive children.
*/
public interface EditConfig extends ComponentEditConfig {
/**
* Name of the edit listener that is invoked after
* a new component was created.
* @see #getListeners()
*/
final String LISTENER_AFTERCREATE = "aftercreate";
/**
* Name of the edit listener that is invoked after
* a component was edited (and modified).
* @see #getListeners()
*/
final String LISTENER_AFTEREDIT = "afteredit";
/**
* Name of the edit listener that is invoked after
* a component was deleted.
* @see #getListeners()
*/
final String LISTENER_AFTERDELETE = "afterdelete";
/**
* Name of the edit listener that is invoked after
* a component was inserted in this container component.
* @see #getListeners()
*/
final String LISTENER_AFTERINSERT = "afterinsert";
/**
* Name of the edit listener that is invoked after
* a component was removed from this container component.
* @see #getListeners()
*/
final String LISTENER_AFTERREMOVE = "afterremove";
/**
* Name of the edit listener that is invoked after
* a component was moved within this container component.
* @see #getListeners()
*/
final String LISTENER_AFTERMOVE = "aftermove";
/**
* Predefined key for the 'CQ.wcm.EditBase.refreshPage' listener.
* @see #getListeners()
*/
final String REFRESH_PAGE = "REFRESH_PAGE";
/**
* Predefined key for the 'CQ.wcm.EditBase.refreshSelf' listener.
* @see #getListeners()
*/
final String REFRESH_SELF = "REFRESH_SELF";
/**
* Predefined key for the 'CQ.wcm.EditBase.refreshParent' listener.
* @see #getListeners()
*/
final String REFRESH_PARENT = "REFRESH_PARENT";
/**
* Sets the edit layout for the edit control widget.
* @param layout edit layout
*/
void setLayout(EditLayout layout);
/**
* Returns amap of configurations of drop targets. the keys are the ids of
* the configs.
*
* Note that the map is modifiable and can be used to extend the drop
* targets dynamically.
*
* @return map of drop target configurations.
*/
Map getDropTargets();
/**
* Sets the dialog mode
* @param mode the mode
*/
void setDialogMode(DialogMode mode);
/**
* Sets the inplace editing configuration.
* @param inplaceEditingConfig The inplace editing configuration
* @since 5.3
*/
void setInplaceEditingConfig(InplaceEditingConfig inplaceEditingConfig);
/**
* Sets the insert behavior
* @param behavior the behavior
*/
void setInsertBehavior(String behavior);
/**
* Sets the empty
flag. If true
this indicates
* that the component has not visual content and a placeholder text should
* be rendered.
*
* @param empty true
if a placeholder text should be rendered.
*/
void setEmpty(boolean empty);
/**
* Sets the text to be displayed when {@link #isEmpty()} is true
.
* If text
is null
then the default text is displayed.
*
* @param text the text or null
*/
void setEmptyText(String text);
/**
* Sets if the edited component is orderable.
*
* @param orderable true
to enable ordering or null
* if the behavior is defined by the widgets.
*/
void setOrderable(Boolean orderable);
/**
* Returns the (modifiable) toolbar for the editbar/menu.
* @return toolbar
*/
Toolbar getToolbar();
/**
* Returns a modifiable map for additional form parameters to be included
* in the dialog.
* @return map of additional form parameters
*/
Map getFormParameters();
/**
* Returns the additional form parameters to be included in the dialog.
* This method allows to use multi value properties for form parameters.
* @return map of additional form parameters
*/
Map getFormParameterMap();
/**
* Returns a modifiable map of edit listeners
* @return map of edit listeners
*/
Map getListeners();
/**
* Set the computed live relationship of the component
* TODO seems like we are only considering this as an additional JSONItem
* to render - if that's confirmed we might rename this method.
* @param liveRelationship the relationship to set
*/
void setLiveRelationship(JSONItem liveRelationship);
/**
* Sets if cancelling inheritance of the component sets the cq:isCancelledForChildren flag or not.
*
* @param deepCancel true
if cancel sets the flag or null
* if cancel does not set the flag.
*/
void setDeepCancel(Boolean deepCancel);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy