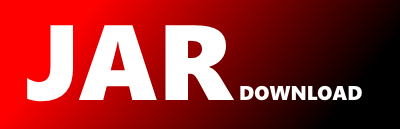
com.day.cq.wcm.api.components.Toolbar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.api.components;
import java.util.LinkedList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
import java.util.Collections;
import java.util.Arrays;
import org.apache.sling.commons.json.JSONException;
import org.apache.sling.commons.json.JSONObject;
import org.apache.sling.commons.json.io.JSONWriter;
/**
* Toolbar
...
*/
public class Toolbar implements List {
private final List- items;
/**
* Creates an empty toolbar
*/
public Toolbar() {
items = new LinkedList
- ();
}
/**
* Creates a new toolbar and adds the given items to it.
* @param items items to add
*/
public Toolbar(Item ... items) {
this.items = new LinkedList
- ();
this.items.addAll(Arrays.asList(items));
}
/**
* Creates a new toolbar and adds the given items to it.
* @param items items to add
*/
public Toolbar(Collection extends Item> items) {
this.items = new LinkedList
- ();
this.items.addAll(items);
}
/**
* Creates an unmodifiable copy of the given toolbar.
* @param toolbar base toolbar
*/
public Toolbar(Toolbar toolbar) {
items = toolbar == null
? Collections.
- emptyList()
: Collections.unmodifiableList(toolbar);
}
public void write(JSONWriter writer, String key) throws JSONException {
if (key != null) {
writer.key(key);
}
writer.array();
for (Item item: items) {
item.write(writer);
}
writer.endArray();
}
public interface Item {
/**
* Serialize to JSON writer.
* @param writer the writer
* @throws JSONException if a JSON error occurs.
*/
void write(JSONWriter writer) throws JSONException;
}
public static class Custom extends JSONObject implements Item {
public void write(JSONWriter writer) throws JSONException {
writer.value(this);
}
}
/**
* Represents a toolbar button
* @since 5.3
*/
public static class Button extends JSONObject implements Item {
public Button(String text, String handler) {
this(text, handler, false, null);
}
public Button(String text, String handler, boolean disabled, String tooltip) {
try {
put("xtype", "button");
put("text", text);
put("handler", handler);
if (tooltip != null) {
put("tooltip", tooltip);
}
if (disabled) {
put("disabled", true);
}
} catch (JSONException e) {
// ignore
}
}
public Button setText(String text) {
try {
put("text", text);
} catch (JSONException e) {
// ignore
}
return this;
}
public Button setHandler(String handler) {
try {
put("handler", handler);
} catch (JSONException e) {
// ignore
}
return this;
}
public Button setTooltip(String tooltip) {
try {
put("tooltip", tooltip);
} catch (JSONException e) {
// ignore
}
return this;
}
public Button setDisabled(boolean disabled) {
try {
put("disabled", disabled);
} catch (JSONException e) {
// ignore
}
return this;
}
public void write(JSONWriter writer) throws JSONException {
writer.value(this);
}
}
public static class Label implements Item {
private final String text;
public Label(String text) {
this.text = text;
}
public void write(JSONWriter writer) throws JSONException {
writer.object();
writer.key("xtype").value("tbtext");
writer.key("text").value(text);
writer.endObject();
}
}
public static class Separator implements Item {
public void write(JSONWriter writer) throws JSONException {
writer.object();
writer.key("xtype").value("tbseparator");
writer.endObject();
}
}
public int size() {
return items.size();
}
public boolean isEmpty() {
return items.isEmpty();
}
public boolean contains(Object o) {
return items.contains(o);
}
public Iterator
- iterator() {
return items.iterator();
}
public Object[] toArray() {
return items.toArray();
}
public
T[] toArray(T[] a) {
return items.toArray(a);
}
public boolean add(Item o) {
return items.add(o);
}
public boolean remove(Object o) {
return items.remove(o);
}
public boolean containsAll(Collection> c) {
return items.containsAll(c);
}
public boolean addAll(Collection extends Item> c) {
return items.addAll(c);
}
public boolean addAll(int index, Collection extends Item> c) {
return items.addAll(index, c);
}
public boolean removeAll(Collection> c) {
return items.removeAll(c);
}
public boolean retainAll(Collection> c) {
return items.retainAll(c);
}
public void clear() {
items.clear();
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Toolbar toolbar = (Toolbar) o;
return items == null
? toolbar.items == null
: items.equals(toolbar.items);
}
public int hashCode() {
return items.hashCode();
}
public Item get(int index) {
return items.get(index);
}
public Item set(int index, Item element) {
return items.set(index, element);
}
public void add(int index, Item element) {
items.add(index, element);
}
public Item remove(int index) {
return items.remove(index);
}
public int indexOf(Object o) {
return items.indexOf(o);
}
public int lastIndexOf(Object o) {
return items.lastIndexOf(o);
}
public ListIterator- listIterator() {
return items.listIterator();
}
public ListIterator
- listIterator(int index) {
return items.listIterator(index);
}
public List
- subList(int fromIndex, int toIndex) {
return items.subList(fromIndex, toIndex);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy