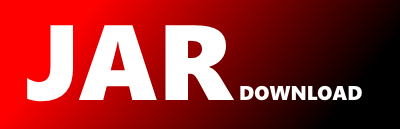
com.day.cq.wcm.api.msm.ActionManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2009 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.api.msm;
import java.util.Set;
import javax.jcr.Node;
import javax.jcr.RepositoryException;
import org.apache.sling.api.SlingHttpServletRequest;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import com.day.cq.wcm.api.WCMException;
/**
* Provides a service to manage live actions
*
* @deprecated since 5.3 use {@link com.day.cq.wcm.msm.api.ActionManager} instead
*/
@Deprecated
public interface ActionManager {
/**
* Registers a LiveAction in the ActionManager. This step is required to enable action is the rollout process.
* @param action action to regiter
*/
public void registerAcion(LiveAction action);
/**
* Unregisters a LiveAction from the ActionManager.
* @param action action to unregiter
*/
public void unregisterAcion(LiveAction action);
/**
* Execute actions found in relationship. Only registered actions will be executed.
* @see LiveAction#execute(org.apache.sling.api.resource.ResourceResolver, LiveRelationship, ActionConfig, boolean)
* @param resolver resource resolver
* @param relation live relationship
* @param autoSave Save modifications
* @throws WCMException occurs if one action is not registered or if action execution throws an exception
*/
public void executeActions(ResourceResolver resolver, LiveRelationship relation, boolean autoSave) throws WCMException;
/**
* Execute actions found in relationship. Only registered actions will be executed.
* @see LiveAction#execute(org.apache.sling.api.resource.ResourceResolver, LiveRelationship, ActionConfig, boolean)
* @param resolver resource resolver
* @param relation live relationship
* @param autoSave Save modifications
* @param isResetRollout True if rollout is run in reset mode
* @throws WCMException occurs if one action is not registered or if action execution throws an exception
*/
public void executeActions(ResourceResolver resolver, LiveRelationship relation, boolean autoSave, boolean isResetRollout) throws WCMException;
/**
* Execute action defined by config. Note that actions found in relationship are not executed.
* Only a registered action will be executed.
* @see LiveAction#execute(org.apache.sling.api.resource.ResourceResolver, LiveRelationship, ActionConfig, boolean)
* @param resolver resource resolver
* @param relation live relationship
* @param config defines actions to execute
* @param autoSave Save modifications
* @throws WCMException occurs if one action is not registered or if action execution throws an exception
*/
public void executeAction(ResourceResolver resolver, LiveRelationship relation, ActionConfig config, boolean autoSave) throws WCMException;
/**
* Execute action defined by config. Note that actions found in relationship are not executed.
* Only a registered action will be executed.
* @see LiveAction#execute(org.apache.sling.api.resource.ResourceResolver, LiveRelationship, ActionConfig, boolean)
* @param resolver resource resolver
* @param relation live relationship
* @param config defines actions to execute
* @param autoSave Save modifications
* @param isResetRollout True if rollout is run in reset mode
* @throws WCMException occurs if one action is not registered or if action execution throws an exception
*/
public void executeAction(ResourceResolver resolver, LiveRelationship relation, ActionConfig config, boolean autoSave, boolean isResetRollout) throws WCMException;
/**
* Returns an ActionConfig object from a resource.
* @param resource base to generate an action config
* @param inherited Mark action config as inherited
* @return constructed ActionConfig, null if construction is not possible
* @throws RepositoryException occurs while reading the repository
*/
public ActionConfig getActionConfig(Resource resource, boolean inherited) throws RepositoryException;
/**
* Returns an ActionConfig object from a resource node.
* @param node base to generate an action config
* @param inherited Mark action config as inherited
* @return constructed ActionConfig, null if construction is not possible
* @throws RepositoryException occurs while reading the repository
*/
public ActionConfig getActionConfig(Node node, boolean inherited) throws RepositoryException;
/**
* Returns all ActionConfig objects from a resource. Checks if resource is from resourceNodeType
* resource type and reads actions under actionNodeName
child node.
* @param resource base to generate an action config
* @param resourceNodeType node type to check on the resource
* @param actionNodeName name of node containing actions
* @param inherited Mark action config as inherited
* @return constructed ActionConfig, null if construction is not possible
* @throws RepositoryException occurs while reading the repository
*/
public Set getActionsConfig(Resource resource, String resourceNodeType, String actionNodeName, boolean inherited) throws RepositoryException;
/**
* Returns all ActionConfig objects from a node. Checks if node is from resourceNodeType
* resource type and reads actions under actionNodeName
child node.
* @param node base to generate an action config
* @param resourceNodeType node type to check on the resource
* @param actionNodeName name of node containing actions
* @param inherited Mark action config as inherited
* @return constructed ActionConfig, null if construction is not possible
* @throws RepositoryException occurs while reading the repository
*/
public Set getActionsConfig(Node node, String resourceNodeType, String actionNodeName, boolean inherited) throws RepositoryException;
/**
* Write config from request to a node. Reads action config in request and writes it in action node.
* @param request the request
* @param actionsNode the action node to write
* @throws WCMException if an error occurs
*/
public void writeConfigFromRequest(SlingHttpServletRequest request, Node actionsNode) throws WCMException;
/**
* Determines if at least one action config defined in relation has been updated in request.
* @param request the request
* @param relation live relationship
* @return true if updated, false otherwise
* @throws WCMException if an error occurs
*/
public boolean isActionUpdated(SlingHttpServletRequest request, LiveRelationship relation) throws WCMException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy