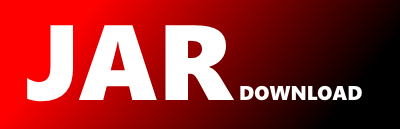
com.day.cq.wcm.designimporter.util.ImageMapExtractor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.wcm.designimporter.util;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.jcr.RepositoryException;
import org.apache.commons.lang.StringUtils;
import com.day.cq.wcm.designimporter.DesignImporterContext;
/**
* This is a utility class for extracting all map elements from the HTML
*/
public class ImageMapExtractor {
private static final String ATTRIBUTE_NAME = "attribute";
private static final String AREA_ATTRIBUTE_REGEX = "(?is)";
private static final String TITLE_ATTR = "title";
private static final String TARGET_ATTR = "target";
private static final String ALT_ATTR = "alt";
private static final String HREF_ATTR = "href";
private static final String COORDS_ATTR = "coords";
private static final String SHAPE_ATTR = "shape";
private static final String AREA_PATTERN_REGEX = "";
private static final String MAP_PATTERN_REGEX = "(?is)";
/**
* This method returns a Map containing the map element name and the stringified form of map element that is required for image
* component.
*
* @param input The html string
* @param designImporterContext
* @return a map containing key as map element name and value as stringified map element
*/
public static Map getImagemapsMap(String input, DesignImporterContext designImporterContext) {
Map imagemapsMap = new HashMap();
Pattern mapPattern = Pattern.compile(MAP_PATTERN_REGEX);
Matcher mapMatcher = mapPattern.matcher(input);
while (mapMatcher.find()) {
String mapElement = mapMatcher.group();
String mapName = mapMatcher.group(1);
Pattern areaPattern = Pattern.compile(AREA_PATTERN_REGEX);
Matcher areaMatcher = areaPattern.matcher(mapElement);
StringBuilder areasBuilder = new StringBuilder();
while (areaMatcher.find()) {
String areaElement = areaMatcher.group();
String translatedArea = translateAreaElement(areaElement, designImporterContext);
areasBuilder.append(translatedArea);
}
imagemapsMap.put(mapName, areasBuilder.toString());
}
return imagemapsMap;
}
public static String getFinalHtml(String input, List maps) {
StringBuffer strBuf = new StringBuffer();
Pattern mapPattern = Pattern.compile(MAP_PATTERN_REGEX);
Matcher mapMatcher = mapPattern.matcher(input);
int startIndex = 0;
int endIndex = 0;
while (mapMatcher.find()) {
mapMatcher.group();
String mapName = mapMatcher.group(1);
if (maps.contains(mapName)) {
endIndex = mapMatcher.start();
strBuf.append(input.substring(startIndex, endIndex));
startIndex = mapMatcher.end();
}
}
strBuf.append(input.substring(startIndex));
return strBuf.toString();
}
/**
* This method parses the area element from map.
*
* @param areaElement The area element
* @return string form of area.
*/
private static String translateAreaElement(String areaElement, DesignImporterContext designImporterContext) {
String shape = getAttributeValue(SHAPE_ATTR, areaElement);
String coords = getAttributeValue(COORDS_ATTR, areaElement);
String href = getAttributeValue(HREF_ATTR, areaElement);
String alt = getAttributeValue(ALT_ATTR, areaElement);
String target = getAttributeValue(TARGET_ATTR, areaElement);
if (StringUtils.isEmpty(alt)) {
alt = getAttributeValue(TITLE_ATTR, areaElement);
}
return getMapText(shape, coords, href, target, alt, designImporterContext);
}
private static String getMapText(String shape, String coords, String href, String target, String title,
DesignImporterContext designImporterContext) {
StringBuffer strBuf = new StringBuffer();
if (!StringUtils.isEmpty(shape)) {
strBuf.append('[').append(shape);
if (!StringUtils.isEmpty(coords)) {
strBuf.append('(').append(coords).append(')');
}
if (!StringUtils.isEmpty(href)) {
if (designImporterContext != null) {
strBuf.append('\"').append(getAbsolutePath(href, designImporterContext)).append('\"');
} else {
strBuf.append('\"').append(href).append('\"');
}
}
strBuf.append('|');
if (!StringUtils.isEmpty(target)) {
strBuf.append('\"').append(target).append('\"');
}
strBuf.append('|');
if (!StringUtils.isEmpty(title)) {
strBuf.append('\"').append(title).append('\"');
}
strBuf.append(']');
}
return strBuf.toString();
}
/**
* This method returns the attribute value for a particular area attribute.
*
* @param attribute The attribute name
* @param element area element
* @return
*/
private static String getAttributeValue(String attribute, String element) {
Pattern attribPattern = Pattern.compile(AREA_ATTRIBUTE_REGEX.replace(ATTRIBUTE_NAME, attribute));
Matcher m = attribPattern.matcher(element);
if (m.find()) {
return m.group(1);
}
return "";
}
private static boolean isAbsoluteUrl(String url) {
try {
new URL(url);
return true;
} catch (MalformedURLException e) {
return false;
}
}
private static String getAbsolutePath(String src, DesignImporterContext designImporterContext) {
if (isAbsoluteUrl(src)) {
return src;
}
try {
if (!designImporterContext.designNode.hasNode(src)) {
designImporterContext.importWarnings.add("Could not locate the referenced map href '" + src + "' in the design archive");
return src;
}
} catch (RepositoryException e) {
}
String designPath = null;
try {
designPath = designImporterContext.designNode.getPath();
} catch (RepositoryException e) {
designImporterContext.importWarnings.add("Could not locate the referenced map href '" + src + "' in the design archive");
return src;
}
// Check if the asset was existent in the archive. If not, log a warning.
return designPath + "/" + src;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy