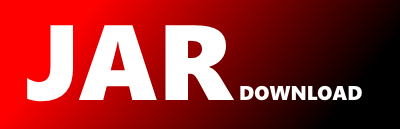
com.day.cq.workflow.job.ExternalProcessJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 2010 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.workflow.job;
import org.apache.sling.event.EventUtil;
import org.osgi.service.event.Event;
import java.io.Serializable;
import java.util.Dictionary;
import java.util.Hashtable;
import java.util.Map;
/**
* Helper class to identify and create external process job events, identified by job topic {@link #JOB_TOPIC}
*/
public class ExternalProcessJob implements Serializable {
private static final long serialVersionUID = 5718625605490930738L;
/**
* The job topic for adding an entry to the audit log.
*/
public static final String JOB_TOPIC = "com/day/cq/workflow/external/job";
public static final String JOB_TOPIC_WC = "com/day/cq/workflow/external/job/*";
/**
* The event property holding the {@link ExternalProcessJob}.
*/
public static final String WORKFLOW_JOB = "com.day.cq.workflow.job";
public static final String WORKFLOW_JOB_ID = "com.day.cq.workflow.jobid";
/**
* The serialized {@link com.day.cq.workflow.exec.WorkItem} {@link java.util.Map} of the job.
*/
protected Map serializedItem;
/**
* Creates a new WorkflowJob.
*
* @param serializedItem {@link Map} of the serialized Item
*/
public ExternalProcessJob(Map serializedItem) {
if (serializedItem == null) {
throw new IllegalArgumentException("Resource must not be null.");
}
this.serializedItem = serializedItem;
}
/**
* Returns the {@link com.day.cq.workflow.exec.WorkItem} {@link java.util.Map}of the job
*
* @return Map The {@link java.util.Map} of the job
*/
public Map getWorkItemMap() {
return serializedItem;
}
/**
* Convenience method to create a job event for the workflow job.
*
* @param retryCount how many times to retry
*
* @param jobId The jobId
*
* @return Event Returns {@link Event} created for the job
*/
public Event createJobEvent(Integer retryCount, String jobId) {
final Dictionary props = new Hashtable();
props.put(WORKFLOW_JOB, this);
props.put(EventUtil.PROPERTY_JOB_RETRIES, retryCount);
if (serializedItem.get("workflowModelId") != null) {
String workflowModelId = (String) serializedItem.get("workflowModelId");
props.put(EventUtil.PROPERTY_JOB_TOPIC, JOB_TOPIC + workflowModelId);
} else {
props.put(EventUtil.PROPERTY_JOB_TOPIC, JOB_TOPIC);
}
props.put(WORKFLOW_JOB_ID, jobId);
return new Event(EventUtil.TOPIC_JOB, props);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy