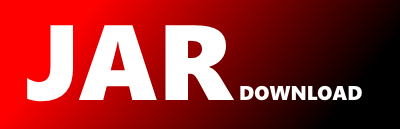
com.day.cq.xss.XSSProtectionService Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*##############################################################################
# ADOBE CONFIDENTIAL
# ___________________
#
# Copyright 2020 Adobe
# All Rights Reserved.
#
# NOTICE: All information contained herein is, and remains
# the property of Adobe and its suppliers, if any. The intellectual
# and technical concepts contained herein are proprietary to Adobe
# and its suppliers and are protected by all applicable intellectual
# property laws, including trade secret and copyright laws.
# Dissemination of this information or reproduction of this material
# is strictly forbidden unless prior written permission is obtained
# from Adobe.
#############################################################################*/
package com.day.cq.xss;
/**
* This interface must be implemented by all services that can be used for preventing
* XSS attacks.
* @deprecated Use the {@link com.adobe.granite.xss.XSSFilter} instead.
*/
@Deprecated
public interface XSSProtectionService {
/**
* Invalidates the given policy.
*
* This is used to declare cached policies as invalid and enforce reloading when
* protectFromXss()
is called the next time.
*
* Invalidating policies manually is not necessary anymore, as changes get detetcted
* automatically since CQ 5.4.
*
* @param policyPath policy path (as used for protectFromXSS()
) to
* invalidate
*/
@Deprecated
void invalidatePolicy(String policyPath);
/**
* Prevents the given source string from containing XSS stuff.
*
* The default policy is used for checking.
*
* @param src source string
* @return string that does not contain XSS stuff
* @throws XSSProtectionException if loading the default policy or scanning the source
* string didn't succeed.
*/
String protectFromXSS(String src) throws XSSProtectionException;
/**
* Protects the given source string from containing XSS stuff.
*
* The default policy is used for checking.
*
* @param src source string
* @param policyPath path to policy configuration node; the default configuration will
* be taken if this parameter is set to null
* @return string that does not contain XSS stuff
* @throws XSSProtectionException if loading the given policy or scanning the source
* string didn't succeed.
*/
String protectFromXSS(String src, String policyPath) throws XSSProtectionException;
/**
* Protected the given source string from containing XSS stuff, considering the
* specified protection context.
*
* For more information about protection contexts, see {@link ProtectionContext} and
* http://www.owasp.org/index.php/XSS_%28Cross_Site_Scripting%29_Prevention_Cheat_Sheet
*
* @param context The protection context
* @param src The string to protect
* @param policyPath The path to a configuration node that contains the policy to be
* used (dependant on the protection context); null
to
* use the default policy.
* @return The protected String
* @throws XSSProtectionException if loading the policy or scanning the source does not
* succeed
* @since 5.4
*/
String protectForContext(ProtectionContext context, String src, String policyPath)
throws XSSProtectionException;
/**
* Protected the given source string from containing XSS stuff, considering the
* specified protection context.
*
* For more information about protection contexts, see {@link ProtectionContext} and
* http://www.owasp.org/index.php/XSS_%28Cross_Site_Scripting%29_Prevention_Cheat_Sheet
*
* @param context The protection context
* @param src The string to protect
* @return The protected String
* @throws XSSProtectionException if loading the policy or scanning the source does not
* succeed
* @since 5.4
*/
String protectForContext(ProtectionContext context, String src)
throws XSSProtectionException;
}