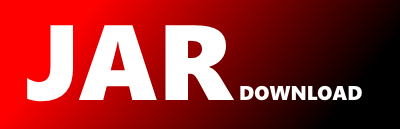
javax.jcr.retention.RetentionManager Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* Copyright 2008 Day Management AG, Switzerland. All rights reserved.
*/
package javax.jcr.retention;
import javax.jcr.AccessDeniedException;
import javax.jcr.PathNotFoundException;
import javax.jcr.RepositoryException;
import javax.jcr.lock.LockException;
import javax.jcr.version.VersionException;
/**
* The RetentionManager
object is accessed via {@link
* javax.jcr.Session#getRetentionManager()}.
*
* @since JCR 2.0
*/
public interface RetentionManager {
/**
* Returns all hold objects that have been added through this API to the
* existing node at absPath
. If no hold has been set before,
* this method returns an empty array.
*
* @param absPath an absolute path.
* @return All hold objects that have been added to the existing node at
* absPath
through this API or an empty array if no
* hold has been set.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficent access to retrieve the node.
* @throws AccessDeniedException if the current session does not have
* sufficient access to retrieve the holds.
* @throws RepositoryException if another error occurs.
*/
public Hold[] getHolds(String absPath) throws PathNotFoundException,
AccessDeniedException, RepositoryException;
/**
* Places a hold on the existing node at absPath
. If
* isDeep
is true
) the hold applies to this node
* and its subgraph. The hold does not take effect until a save
* is performed. A node may have more than one hold.
*
* The format and interpretation of the name
are not specified.
* They are application-dependent.
*
* A VersionException
will be thrown either immediately, on
* dispatch or on persists, if the node at absPath
is read-only
* due to a checked-in node. Implementations may differ on when this
* validation is performed.
*
* A LockException
will be thrown either immediately, on
* dispatch or on persists, if a lock prevents the operation.
* Implementations may differ on when this validation is performed.
*
* @param absPath an absolute path.
* @param name an application-dependent string.
* @param isDeep a boolean indicating if the hold applies to the subgraph.
* @return The Hold
applied.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve the node.
* @throws AccessDeniedException if the current session does not have
* sufficient access to perform the operation.
* @throws LockException if a lock applies at the node at
* absPath
and this implementation performs this validation
* immediately.
* @throws VersionException if the node at absPath
is read-only
* due to a checked-in node. and this implementation performs this
* validation immediately.
* @throws RepositoryException if another error occurs.
*/
public Hold addHold(String absPath, String name, boolean isDeep)
throws PathNotFoundException, AccessDeniedException,
LockException, VersionException, RepositoryException;
/**
* Removes the specified hold
from the node at
* absPath
. The removal does not take effect until a
* save
is performed.
*
* A VersionException
will be thrown either immediately, on
* dispatch or on persists, if the node at absPath
is read-only
* due to a checked-in node. Implementations may differ on when this
* validation is performed.
*
* A LockException
will be thrown either immediately, on
* dispatch or on persists, if a lock prevents the operation.
* Implementations may differ on when this validation is performed.
*
* @param absPath an absolute path.
* @param hold the hold to be removed.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve the node.
* @throws AccessDeniedException if the current session does not have
* sufficient access to perform the operation.
* @throws LockException if a lock applies at the node at
* absPath
and this implementation performs this validation
* immediately.
* @throws VersionException if the node at absPath
is read-only
* due to a checked-in node and this implementation performs this validation
* immediately.
* @throws RepositoryException if another error occurs.
*/
public void removeHold(String absPath, Hold hold)
throws PathNotFoundException, AccessDeniedException, LockException,
VersionException, RepositoryException;
/**
* Returns the retention policy that has been set using {@link
* #setRetentionPolicy} on the node at absPath
or
* null
if no policy has been set.
*
* @param absPath an absolute path to an existing node.
* @return The retention policy that applies to the existing node at
* absPath
or null
if no policy applies.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficent access to retrieve the node.
* @throws AccessDeniedException if the current session does not have
* sufficient access to retrieve the policy.
* @throws RepositoryException if another error occurs.
*/
public RetentionPolicy getRetentionPolicy(String absPath)
throws PathNotFoundException, AccessDeniedException, RepositoryException;
/**
* Sets the retention policy of the node at absPath
to that
* defined in the specified policy node. Interpretation and enforcement of
* this policy is an implementation issue. In any case the policy does does
* not take effect until a save
is performed.
*
* A VersionException
will be thrown either immediately, on
* dispatch or on persists, if the node at absPath
is read-only
* due to a checked-in node. Implementations may differ on when this
* validation is performed.
*
* A LockException
will be thrown either immediately, on
* dispatch or on persists, if a lock prevents the operation.
* Implementations may differ on when this validation is performed.
*
* @param absPath an absolute path to an existing node.
* @param retentionPolicy a retention policy.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve the node.
* @throws AccessDeniedException if the current session does not have
* sufficient access to perform the operation.
* @throws LockException if a lock applies at the node at
* absPath
and this implementation performs this validation
* immediately.
* @throws VersionException if the node at absPath
is read-only
* due to a checked-in node and this implementation performs this validation
* immediately.
* @throws RepositoryException if another error occurs.
*/
public void setRetentionPolicy(String absPath, RetentionPolicy retentionPolicy)
throws PathNotFoundException, AccessDeniedException, LockException,
VersionException, RepositoryException;
/**
* Causes the current retention policy on the node at absPath
* to no longer apply. The removal does not take effect until a
* save
is performed.
*
* A VersionException
will be thrown either immediately, on
* dispatch or on persists, if the node at absPath
is read-only
* due to a checked-in node. Implementations may differ on when this
* validation is performed.
*
* A LockException
will be thrown either immediately, on
* dispatch or on persists, if a lock prevents the operation.
* Implementations may differ on when this validation is performed.
*
* @param absPath an absolute path to an existing node.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve the node.
* @throws AccessDeniedException if the current session does not have
* sufficient access to perform the operation.
* @throws LockException if a lock applies at the node at
* absPath
and this implementation performs this validation
* immediately.
* @throws VersionException if the node at absPath
is read-only
* due to a checked-in node and this implementation performs this validation
* immediately.
* @throws RepositoryException if another error occurs.
*/
public void removeRetentionPolicy(String absPath)
throws PathNotFoundException, AccessDeniedException,
LockException, VersionException, RepositoryException;
}