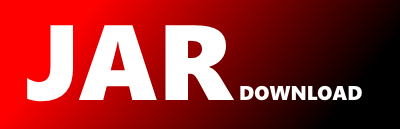
com.adobe.xfa.service.renderer.FormUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2009 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.service.renderer;
import java.util.StringTokenizer;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.Element;
import com.adobe.xfa.EnumAttr;
import com.adobe.xfa.Model;
import com.adobe.xfa.Node;
import com.adobe.xfa.STRS;
import com.adobe.xfa.XFA;
import com.adobe.xfa.XMLMultiSelectNode;
import com.adobe.xfa.content.ExDataValue;
import com.adobe.xfa.form.FormField;
import com.adobe.xfa.layout.Layout;
import com.adobe.xfa.layout.LayoutNode;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.ResId;
import com.adobe.xfa.ut.StringHolder;
import com.adobe.xfa.ut.StringUtils;
/**
* @exclude from published api.
*/
public class FormUtils {
/**
* @exclude from published api.
*/
public enum LayerType {ePrintAndView, ePrintOnly, eViewOnly, eNotSet};
public static LayerType getLayer(Element oFormNode) {
if (oFormNode != null) {
LayerType eLayer = getExplicitLayer(oFormNode);
if (eLayer != LayerType.ePrintAndView)
return eLayer;
Element oParent = oFormNode.getXFAParent();
if (oParent == null)
return LayerType.ePrintAndView;
return getLayer(oParent);
}
return LayerType.ePrintAndView;
}
public static LayerType getLayer(Layout oLayout, Element oFormNode) {
LayoutNode oLayoutNode= findRootXFALayoutNodeForFormNode(oLayout, oFormNode);
Element oFNode = oLayoutNode.getFormNode();
return getLayer(oFNode);
}
static LayerType getExplicitLayer(Element oFormNode) {
if (oFormNode != null) {
// Get the form node.
if (!oFormNode.isPropertySpecified(XFA.RELEVANTTAG, true, 0))
return LayerType.ePrintAndView;
Attribute oRelevantAttr = oFormNode.getAttribute(XFA.RELEVANTTAG);
String sRelevant = oRelevantAttr.getAttrValue();
StringTokenizer sToker = new StringTokenizer(sRelevant);
while (sToker.hasMoreTokens()) {
String sLayer = sToker.nextToken();
// visible but don't print
if (sLayer.equals("-print"))
return LayerType.eViewOnly;
// invisible but print
else if (sLayer.equals("print") || sLayer.equals("+print")) {
Model oModel = oFormNode.getModel();
AppModel oAppModel = (AppModel) oModel.getXFAParent();
if (oAppModel != null && oAppModel.getLegacySetting(AppModel.XFA_LEGACY_PLUSPRINT))
return LayerType.ePrintAndView;
return LayerType.ePrintOnly;
}
}
}
return LayerType.ePrintAndView;
}
public static LayoutNode findRootXFALayoutNodeForFormNode(Layout oLayout, Element oFormField) {
// JavaPort: Should never not be called at this time.
throw new ExFull(ResId.UNSUPPORTED_OPERATION, "getToolTipText");
// JavaPort: TODO.
// LayoutNode oRetNode;
// int nNumPages = oLayout.getPageCount();
// for(int i=0; i< nNumPages; i++) {
// LayoutNode oRootLayoutNode = oLayout.getPageNode(i);
// if(oRootLayoutNode != null) {
// oRetNode = FindXFALayoutNodeForFormNode(oRootLayoutNode, oFormNode);
// if (oRetNode != null)
// return oRetNode;
// }
// }
// return oRetNode;
}
public static String getToolTipText(LayoutNode layoutNode) {
// JavaPort: Should never not be called at this time.
throw new ExFull(ResId.UNSUPPORTED_OPERATION, "getToolTipText");
}
static int getDisplayCode(LayoutNode oLayoutNode, FormField oFormField) {
// Vis ""
// Vis (Screen only) relevant="-print"
// Vis (Print only) relevant="+print"
// Invis presence="invisible"
// Hidden presence="hidden"
LayerType elayer = getLayer(oLayoutNode.getFormNode());
int oPresence = oFormField.getEnum(XFA.PRESENCETAG);
int nDisplay;
if (oPresence == EnumAttr.PRESENCE_INVISIBLE)
nDisplay = 1; /* Hidden. */
else if (elayer == LayerType.eViewOnly)
nDisplay = 2; /* Visible but not printed. */
else if (elayer == LayerType.ePrintOnly)
nDisplay = 3; /* Hidden but printed. */
else
nDisplay = 0; /* Visible and printable */
return nDisplay;
}
public static int getDisplayCode(Layout oLayout, FormField oFormField) {
LayoutNode oLayoutNode= findRootXFALayoutNodeForFormNode(oLayout, oFormField);
return getDisplayCode(oLayoutNode, oFormField);
}
public static void setDisplayCode(FormField oFormField, int nDisplay) {
// Vis ""
// Vis (Screen only) relevant="-print"
// Vis (Print only) relevant="+print"
// Invis presence="invisible"
// Hidden presence="hidden"
if (nDisplay == 0) { /* Visible and printable */
oFormField.setAttribute(EnumAttr.PRESENCE_VISIBLE, XFA.PRESENCETAG);
oFormField.setProperty("", XFA.RELEVANTTAG);
}
else if (nDisplay == 1) { /* Hidden. */
oFormField.setAttribute(EnumAttr.PRESENCE_INVISIBLE, XFA.PRESENCETAG);
oFormField.setProperty("", XFA.RELEVANTTAG);
}
else if (nDisplay == 2) { /* Visible but not printed. */
oFormField.setAttribute(EnumAttr.PRESENCE_VISIBLE, XFA.PRESENCETAG);
oFormField.setProperty("-print", XFA.RELEVANTTAG);
}
else { // if (nDisplay == 3) /* Hidden but printed. */
oFormField.setAttribute(EnumAttr.PRESENCE_INVISIBLE, XFA.PRESENCETAG);
oFormField.setProperty("+print", XFA.RELEVANTTAG);
}
}
public static boolean getRichTextField(FormField oField, StringHolder sMarkup) {
if (oField != null) {
Element oValueNode = oField.getElement(XFA.VALUETAG, 0);
if (oValueNode != null) {
Node oContentNode = oValueNode.getOneOfChild();
if (oContentNode == null)
return false;
if (oContentNode instanceof ExDataValue) {
ExDataValue oExData = (ExDataValue) oContentNode;
Node aNode = oExData.getOneOfChild();
if (oExData.getAttribute(XFA.CONTENTTYPETAG).toString().equals(STRS.TEXTPLAIN)) {
// contentType = text/plain
return false;
}
if (aNode instanceof XMLMultiSelectNode) {
// contentType = text/xml
return false;
}
//Access the xhtml markup string
if (sMarkup != null) {
String sTemp = ((ExDataValue) oContentNode).getValue(true, false, false);
// Remove LF characters...
if (sTemp.indexOf('\n') >= 0)
sTemp = sTemp.replace("\n", "");
// Replace CR characters with entity reference...
sMarkup.value = StringUtils.toXML(sTemp, StringUtils.ToXMLType.XMLTEXT, "", '\r', '\r', "<&>'\"");
}
return true;
}
}
}
return false;
}
/*
* Disallow any instance of this class.
*/
private FormUtils() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy