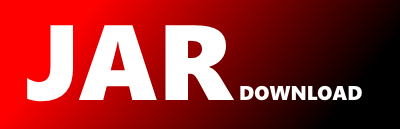
com.adobe.cq.projects.api.ProjectManager Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2013 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.cq.projects.api;
import aQute.bnd.annotation.ProviderType;
import com.adobe.granite.workflow.model.WorkflowModel;
import org.apache.sling.api.resource.Resource;
import java.util.Iterator;
import java.util.List;
/**
* The ProjectManager
provides the ability to retrieve projects,
* delete projects, and create projects.
*
* ProjectManager
instances are adapted from ResourceResolver
.
*
* Note that Projects can also be adapted from a Resource
if that resource represents a Project.
*
* Note that all changes are transient and need to be committed by the caller.
* For example:
*
* ResourceResolver resourceResolver = ...
* ProjectManager pm = resourceResolver.adaptTo(ProjectManager.class);
* Project project = pm.createProject("Test 1");
* project.setDescription("This is a test project");
* resourceResolver.commit();
*
*
*
* @see org.apache.sling.api.resource.ResourceResolver
* @see org.apache.sling.api.resource.Resource
*/
@ProviderType
public interface ProjectManager {
/**
* Retrieves an iterator over projects that are accessible by the current user.
* @param filter used to filter the list of returned projects
* @param startIndex the index of the first project to retrieve
* @param limit the maximum number of projects to retrieve
* @return an iterator over Projects.
* @throws ProjectException if the loading of the projects fails.
*/
Iterator getProjects(ProjectFilter filter, int startIndex, int limit);
/**
* Retrieves an iterator over project and folder resources in the specified folder.
*
* The returned iterator also hides the legacy bucket structure of projects by returning any projects in
* a bucket under the current path as part its parent folder.
*
* E.g. iterating over /content/projects, where the structure is:
* /content/projects/bucket/project1
* /content/projects/bucket/project2
* /content/projects/project3
* /content/projects/somefolder/project4
* this will in the iteration return:
* - 'project1' and 'project2' (because the bucket structure is hidden by this iterator)
* - 'project3' (since it's part of the current parent of /content/projects)
* - 'somefolder' (since it's also part of the current parent)
* however it will not include 'project4' since it is under a regular folder 'somefolder'
*
* @param folderPath root path where to start iterating from
* @param filter used to filter the list of returned projects
* @param startIndex the index of the first project to retrieve. A negative value behaves the same as a value
* of 0.
* @param limit the maximum number of projects to retrieve. A negative value means no limit.
* @return an iterator over Projects.
* @throws ProjectException if the loading of the projects fails.
*/
Iterator getChildren(String folderPath, ProjectFilter filter, int startIndex, int limit);
/**
* Deletes the specified project.
* @param project the project to delete.
* @throws ProjectException if the project can not be deleted, for example due to access restrictions.
*/
void deleteProject(Project project);
/**
* Creates a new project with the specified title.
* @param title the title of the new project
* @param templatePath the path of the template to create
* the project with.
* @return a new project instance
* @throws ProjectException in case an error occurred.
*/
Project createProject(String title, String templatePath);
/**
* Creates a new project with the specified title.
* @param nodeName the name of the node that will be created. If a node
* exists with the current name a unique name will be generated
* with the nodeName as the prefix.
* @param title the title of the new project
* @param templatePath the path of the template to create
* the project with.
* @return a new project instance
* @throws ProjectException in case an error occurred.
*/
Project createProject(String nodeName, String title, String templatePath);
/**
* Creates a new project with the specified root folder, title and template.
* @param parentPath the parent path of where this project will be created.
* @param nodeName the name of the node that will be created. If a node
* exists with the current name a unique name will be generated
* with the nodeName as the prefix.
* @param title the title of the new project
* @param templatePath the path of the template to create
* the project with.
* @return a new project instance
* @throws ProjectException in case an error occurred.
*/
Project createProject(String parentPath, String nodeName, String title, String templatePath);
/**
* Creates a new project from Master Project with the specified root folder, title and template.
* @param parentPath the parent path of where this project will be created.
* @param nodeName the name of the node that will be created. If a node exists with the current
* name a unique name will be generated with the nodeName as the prefix.
* @param title the title of the new project
* @param templatePath the path of the template to create the project with.
* @param description the description of the new project
* @param masterProject the Master Project which would be used to create the new project
* @param useDefaultCoverImage whether to use default cover image or not
* @return a new project instance
*/
Project createProjectFromMasterProject(String parentPath, String nodeName, String title, String templatePath,
String description, Project masterProject, boolean useDefaultCoverImage);
/**
* Return the list of workflows that are available for the project.
*
* @param project the project which to retrieve the available
* WorkflowModel
s for.
* @return the collection of available WorkflowModel
s.
*/
List getWorkflows(Project project);
}