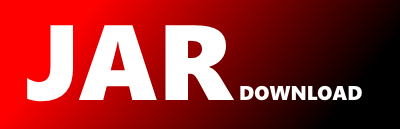
com.adobe.cq.wcm.launches.utils.LaunchUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
/**
* AdobePatentID="3435US01"
*/
package com.adobe.cq.wcm.launches.utils;
import com.adobe.cq.launches.api.Launch;
import com.adobe.cq.launches.api.LaunchException;
import com.adobe.cq.launches.api.LaunchSource;
import com.adobe.cq.wcm.launches.impl.LaunchConstants;
import com.adobe.cq.wcm.launches.impl.LaunchManagerImpl;
import com.day.cq.commons.jcr.JcrConstants;
import com.day.cq.wcm.api.Page;
import com.day.text.Text;
import org.apache.commons.lang.StringUtils;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ValueMap;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.LinkedList;
import java.util.List;
import java.util.ListIterator;
import static com.adobe.cq.wcm.launches.impl.LaunchConstants.LAUNCHES_ROOT_PATH;
/**
* Utilities for WCM launches.
*/
public class LaunchUtils {
private static final Logger log = LoggerFactory.getLogger(LaunchUtils.class);
/**
* Content tree level where to find the launch (starts at 0)
*/
private static final int LAUNCH_NAME_LEVEL = 2;
/**
* Returns the resource of the launch the provided resource belongs to
*
* @param resource Resource to check
* @return Resource of the launch the provided resource belongs to
*/
public static Resource getLaunchResource(Resource resource) {
if (resource == null) {
throw new IllegalArgumentException("Resource cannot be null");
}
while (resource != null) {
final Resource contentRes = resource.getChild(JcrConstants.JCR_CONTENT);
if (contentRes != null && contentRes.isResourceType(LaunchConstants.RT_LAUNCH)) {
return resource;
}
resource = resource.getParent();
}
return null;
}
/**
* Given a launch-based resource, this method returns the corresponding resource in the source of the
* launch. For nested launches, the returned resource is launch-based. For first-level launches, the
* returned resource is the production version.
*
* @deprecated Use {@link #getTargetResource} instead
* @param resource A launch-based resource
* @return the source version of the resource
*/
public static Resource getProductionResource(final Resource resource) {
if (!isLaunchResourcePath(resource.getPath())) {
return resource;
}
final Resource launchResource = getLaunchResource(resource);
return resource.getResourceResolver().getResource(resource.getPath().substring(launchResource.getPath().length()));
}
/**
* Given a launch-based resource, this method returns the corresponding resource in the specified target launch.
* The target launch must be a parent launch of the launch that resource
is part of. If
* target
is null
, the production version of the resource is returned.
*
* @param resource a launch-based resource
* @param target a parent launch or null
to get the production version
* @return the resource at the specified target
* @throws LaunchException if the specified target is not a parent launch
*/
public static Resource getTargetResource(Resource resource, Launch target)
throws LaunchException {
String path = getTargetResourcePath(resource, target);
return resource.getResourceResolver().getResource(path);
}
/**
* Constructs the target path of a launch-based resource according to {@link #getTargetResource(Resource, Launch)}.
* The returned path might not resolve (if the target resource does not exist).
*
* @param resource a launch-based resource
* @param target a parent launch or null
to get the production version
* @return the path of the resource at the specified target
* @throws LaunchException if the specified target is not a parent launch
*/
public static String getTargetResourcePath(Resource resource, Launch target)
throws LaunchException {
// check if the resource is launch-based
if (!isLaunchResourcePath(resource.getPath())) {
return target == null ? resource.getPath() : null;
}
// get the launch of the resource
Launch currentLaunch = getLaunchResource(resource).adaptTo(Launch.class);
// start with the path of the launch-based resource
String path = resource.getPath();
// go up the launch hierarchy until hitting the target launch (or the production version)
while (target == null || currentLaunch.compareTo(target) != 0) {
// strip prefix due to current launch
path = path.substring(currentLaunch.getResource().getPath().length());
// get parent launch
Resource launchResource = getLaunchResource(currentLaunch.getSourceRootResource());
if (launchResource == null) {
// there is no parent launch, we have hit production
if (target != null) {
throw new LaunchException("The specified target launch is not a parent launch " +
"of the resource's launch");
}
break;
}
currentLaunch = launchResource.adaptTo(Launch.class);
}
return path;
}
/**
* Given a resource, which can be production or launch-based, returns the corresponding resource in
* the specified (and possibly nested) launch.
*
* @param launch a (possibly nested) launch
* @param resource a (source) resource
* @return the corresponding resource in the launch
* @throws LaunchException if the resource is launch-based and the specified launch is not a child launch
*/
public static Resource getLaunchResource(final Launch launch, Resource resource)
throws LaunchException {
if (launch == null || resource == null) {
return null;
}
String path = getLaunchResourcePath(launch, resource);
return resource.getResourceResolver().getResource(path);
}
/**
* Constructs the path of a resource in the specified launch according to {@link #getLaunchResource(Launch,Resource)}.
* The returned path might not resolve (if the resource does not exist in the launch).
*
* @param launch a (possibly nested) launch
* @param resource a (source) resource
* @return the path of the resource in the launch
* @throws LaunchException if the resource is launch-based and the specified launch is not a child launch, or if the
* specified launch has an invalid parent launch in the launch hierarchy
*/
public static String getLaunchResourcePath(Launch launch, Resource resource)
throws LaunchException {
if (launch == null || resource == null) {
return null;
}
// get the launch of the resource (if any)
Resource res = getLaunchResource(resource);
Launch resourceLaunch = res == null ? null : res.adaptTo(Launch.class);
// list storing parent launches
List parents = new LinkedList();
Launch currentLaunch = launch;
// collect parent launches of 'launch' until we either hit the launch that
// 'resource' is part of, or hit production
while (resourceLaunch == null || currentLaunch.compareTo(resourceLaunch) != 0) {
parents.add(currentLaunch);
// get parent launch
Resource launchResource = getLaunchResource(currentLaunch.getSourceRootResource());
if (launchResource == null) {
// there is no more parent launch, we have hit production
if (resourceLaunch != null) {
throw new LaunchException("The specified launch is not a child launch " +
"of the resource's launch");
}
break;
}
currentLaunch = launchResource.adaptTo(Launch.class);
if (currentLaunch == null) {
throw new LaunchException("Invalid parent launch " + launchResource.getPath());
}
}
// build path by concatenating the paths of the parent launches' root resources
StringBuilder path = new StringBuilder(resource.getPath());
ListIterator reverseIterator = parents.listIterator(parents.size());
while (reverseIterator.hasPrevious()) {
path.insert(0, reverseIterator.previous().getResource().getPath());
}
return path.toString();
}
/**
* Returns true
if the provided path is a launch-based path
*
* @param path The path to check
* @return true
if the provided path is a launch-based path
*/
public static boolean isLaunchBasedPath(String path) {
return Text.isDescendantOrEqual(LAUNCHES_ROOT_PATH, path);
}
/**
* Returns true
if the provided path is a launch-based path
* within the provided launch path
*
* @param path The path to check
* @param launchPath The launch path
* @return true
if the provided path is a launch-based path
* within the provided launch path
*/
static boolean isLaunchBasedPath(String path, String launchPath) {
// TODO: Any reason why this method is not public?
return Text.isDescendantOrEqual(launchPath, path);
}
/**
* Returns true
if the provided path is a launch resource path
*
* @param path Path to check
* @return true
if the provided path is a launch resource path
*/
public static boolean isLaunchResourcePath(String path) {
return isLaunchBasedPath(path) && StringUtils.isNotEmpty(Text.getAbsoluteParent(path, LAUNCH_NAME_LEVEL + 1));
}
/**
* Warning: this method is deprecated. It will not work with the new launches structure.
*
* Returns the launch resource path corresponding to the provided launch-based path
*
* @param path The launch-based path
* @return Launch resource path corresponding to the provided launch-based path
* or null
if provided path does not belong to a launch
* @deprecated since 6.0.0 - use {@link #getLaunchResource(Resource)} instead.
*/
public static String getLaunchPath(String path) {
log.warn("LaunchUtils.getLaunchPath is deprecated and potentially broken. Please remove usages of this method.");
if (isLaunchBasedPath(path)) {
String launchPath = Text.getAbsoluteParent(path, LAUNCH_NAME_LEVEL);
return StringUtils.isNotEmpty(launchPath) ? launchPath : null;
}
return null;
}
/**
* Warning: this method is deprecated. It will not work with the new launches structure.
*
* Returns the launch resource path from the provided launch name
*
* @param launchName The launch name
* @return Launch resource path from the provided launch name
*
* @deprecated since 6.0.0
*/
public static String getLaunchPathFromName(String launchName) {
return Text.makeCanonicalPath(LAUNCHES_ROOT_PATH + "/" + launchName);
}
/**
* Returns the production resource path corresponding to the provided launch-based resource path
*
* @param path The launch-based resource path
* @return Production resource path corresponding to the provided launch-based resource path
* or the provided path if it isn't a launch-based resource path
* @deprecated since 6.0.0
*/
public static String getProductionResourcePath(String path) {
return isLaunchResourcePath(path) ? StringUtils.substringAfter(path, getLaunchPath(path)) : path;
}
/**
* Returns the launch resource path corresponding to a provided path and a launch name
*
* @param path The path to build the launch resource path from
* @param launchName Launch name
* @return Returns the resource path within the provided launch or null
if the provided path is already
* a launch-based resource path from a different launch than the provided one
* @deprecated since 6.0.0
*/
public static String buildLaunchResourcePath(String path, String launchName) {
log.warn("LaunchUtils#buildLaunchResourcePath is deprecated and potentially broken. Please remove usages of this method.");
if (isLaunchResourcePath(path)) {
// Provided path is already a launch-based path, check if it belongs to the current one
return isLaunchBasedPath(path, getLaunchPathFromName(launchName)) ? path : null;
}
// Build launch resource path
return !isLaunchBasedPath(path) ? Text.makeCanonicalPath(LAUNCHES_ROOT_PATH + "/" + launchName + "/" + path) : null;
}
/**
* returns true if the given launch resource is approved
*
* @param resource launch based resource
* @return returns the boolean
*/
public static boolean isApprovedLaunchResource(Resource resource) {
if (resource != null && isLaunchBasedPath(resource.getPath())) {
Page resPage = resource.adaptTo(Page.class);
if (resPage != null) {
ValueMap resVM = resPage.getProperties();
String status = resVM.get(LaunchManagerImpl.STATUS, String.class);
return LaunchManagerImpl.APPROVED.equals(status);
}
}
return false;
}
public static LaunchSource findNearestLaunchSource(Resource inputResource, List launchSourceList) {
LaunchSource startResourceSource = null;
for (LaunchSource source : launchSourceList) {
Resource sourceResource = source.getSourceRootResource();
if (inputResource.getPath().indexOf(sourceResource.getPath()) != -1) {
if (startResourceSource == null) {
startResourceSource = source;
} else {
// check is deeper, or path length greater
if (sourceResource.getPath().length() > startResourceSource.getSourceRootResource().getPath()
.length()) {
startResourceSource = source;
}
}
}
}
return startResourceSource;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy