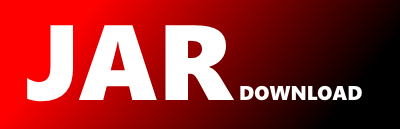
com.adobe.fontengine.CharUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: CharUtil.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import com.adobe.agl.lang.UCharacter;
import com.adobe.agl.lang.UCharacterCategory;
final public class CharUtil {
private static final CombiningSequence emptySequence;
static {
try {
InputStream is = CombiningSequence.class.getResourceAsStream ("composer");
ObjectInputStream ois = new ObjectInputStream (is);
emptySequence = (CombiningSequence) ois.readObject ();
ois.close (); }
catch (IOException e) {
throw new RuntimeException ("cannot load AFE Composer resources", e); }
catch (ClassNotFoundException e) {
throw new RuntimeException ("cannot load AFE Composer resources", e); }}
/** Return the entry to lookup in a cmap for a combining sequence.
*
* @param usvs contains the sequence
* @param start the index of the first character
* @param limit the index following the last character
* @return the entry to lookup, -1 if none
*/
public static int compose (int[] usvs, int start, int limit) {
return emptySequence.compose (usvs, start, limit);
}
/** Return the entries to populate in a cmap for a glyph that
* represents a given string.
*
* This method accounts for all canonical equivalences except
* CJK compatibility characters.
*
* @param usvs contains the string
* @param start the index in usvs of the first character
* @param limit the index in usvs following the last character
* @return the cmap entries to populate; if none, an empty array
*/
public static int[] mapStringFrom (int[] usvs, int start, int limit) {
int c = composeHangulSyllable (usvs, start, limit);
if (c != -1) {
return new int [] {c}; }
else {
return emptySequence.map (usvs, start, limit); }
}
public static int composeHangulSyllable (int[] usvs, int start, int limit) {
if ( start + 2 <= limit
&& 0x1100 <= usvs [start] && usvs [start] <= 0x1100 + 19
&& 0x1161 <= usvs [start+1] && usvs [start+1] <= 0x1161 + 21
&& ( ( limit == start + 3
&& 0x11a7 <= usvs [start+2] && usvs [start+2] <= 0x11a7 + 28)
|| limit == start + 2)) {
int s = ((usvs [start] - 0x1100) * 21 + (usvs [start+1] - 0x1161)) * 28 + 0xac00;
if (limit == start + 3) {
s += usvs [start+2] - 0x11a7; }
return s; }
else {
return -1; }
}
public static boolean isBase (int ch) {
return UCharacter.isBaseForm (ch);
}
public static boolean isCombining (int ch) {
int gc = UCharacter.getType (ch);
return ( gc == UCharacterCategory.COMBINING_SPACING_MARK
|| gc == UCharacterCategory.NON_SPACING_MARK
|| gc == UCharacterCategory.ENCLOSING_MARK);
}
public static boolean isControl (int ch) {
int gc = UCharacter.getType (ch);
return ( gc == UCharacterCategory.FORMAT
|| gc == UCharacterCategory.CONTROL);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy