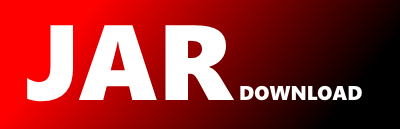
com.adobe.fontengine.font.FontImpl Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
*
* File: FontImpl.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font;
import java.util.Map;
import com.adobe.agl.util.ULocale;
import com.adobe.fontengine.fontmanagement.CacheSupportInfo;
import com.adobe.fontengine.fontmanagement.Platform;
import com.adobe.fontengine.fontmanagement.fxg.FXGFontDescription;
import com.adobe.fontengine.fontmanagement.platform.PlatformFontDescription;
import com.adobe.fontengine.fontmanagement.postscript.PostscriptFontDescription;
import com.adobe.fontengine.inlineformatting.css20.CSS20FontDescription;
/**
* The base class for all Font objects.
*
* This class provides the basic functionality common to all Fonts.
*
* Synchronization
*
* These objects are immutable.
*/
abstract public class FontImpl implements Font {
static final long serialVersionUID = 1;
public double getUnitsPerEmX ()
throws UnsupportedFontException, InvalidFontException, FontLoadingException
{
try {
return this.getFontData ().getUnitsPerEmX (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public double getUnitsPerEmY ()
throws UnsupportedFontException, InvalidFontException, FontLoadingException
{
try {
return this.getFontData ().getUnitsPerEmY (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
/** Return the line metrics for this font.
*
* The metrics are expressed in the design space of the font,
* i.e. they need to be converted through the metrics matrix.
*
*
Some font formats do not support the notion of line metrics,
* and in those cases, this method returns null.
*
*
See also the {@link #getCoolTypeLineMetrics()} method.
*
* @throws UnsupportedFontException
* @throws InvalidFontException
* @throws FontLoadingException
*/
public LineMetrics getLineMetrics ()
throws UnsupportedFontException, InvalidFontException, FontLoadingException {
try {
return this.getFontData ().getLineMetrics (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public Rect getCoolTypeGlyphBBox(int glyphID)
throws UnsupportedFontException, InvalidFontException, FontLoadingException {
try {
return this.getFontData ().getCoolTypeGlyphBBox (glyphID); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
/** Emulates the CoolType API CTFontDict:GetHorizontalMetrics.
*
*
The metrics are expressed in the metric space of the font.
*
*
See also the {@link #getLineMetrics()} method.
*
* @throws UnsupportedFontException
* @throws InvalidFontException
* @throws FontLoadingException
*/
public LineMetrics getCoolTypeLineMetrics ()
throws UnsupportedFontException, InvalidFontException, FontLoadingException {
try {
return this.getFontData ().getCoolTypeLineMetrics (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public CoolTypeScript getCoolTypeScript() throws FontLoadingException, InvalidFontException, UnsupportedFontException
{
try {
return this.getFontData ().getCoolTypeScript(); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
/** Emulates the CoolType API CTFontDict:GetUnderlineInfo.
*
*
The metrics are expressed in the metric space of the font.
*
* @throws UnsupportedFontException
* @throws InvalidFontException
* @throws FontLoadingException
*/
public UnderlineMetrics getCoolTypeUnderlineMetrics ()
throws UnsupportedFontException, InvalidFontException, FontLoadingException{
try {
return this.getFontData ().getCoolTypeUnderlineMetrics (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
/** Emulates the CoolType API CTFontDict:GetIdeoEmBox.
*
*
The metrics are expressed in the metric space of the font.
*
* @throws UnsupportedFontException
* @throws InvalidFontException
* @throws FontLoadingException
*/
public Rect getCoolTypeIdeoEmBox ()
throws UnsupportedFontException, InvalidFontException, FontLoadingException {
try {
return this.getFontData ().getCoolTypeIdeoEmBox (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
/**
* Emulates the CoolType API CTFontDict::hasPropRoman.
* @return true if the font contains proportional roman glyphs.
* @throws UnsupportedFontException
* @throws InvalidFontException
* @throws FontLoadingException
*/
public boolean hasCoolTypeProportionalRoman()
throws UnsupportedFontException, InvalidFontException, FontLoadingException
{
try {
return this.getFontData ().hasCoolTypeProportionalRoman (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
/** Emulates the CoolType API CTFontDict:GetICFBox.
*
*
The metrics are expressed in the metric space of the font.
*
* @throws UnsupportedFontException
* @throws InvalidFontException
* @throws FontLoadingException
*/
public Rect getCoolTypeIcfBox ()
throws UnsupportedFontException, InvalidFontException, FontLoadingException {
try {
return this.getFontData ().getCoolTypeIcfBox (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
/** Tell whether the font is symbolic.
* @throws UnsupportedFontException
* @throws InvalidFontException
* @throws FontLoadingException
*/
public boolean isSymbolic ()
throws UnsupportedFontException, InvalidFontException, FontLoadingException {
try {
return getFontData ().isSymbolic (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
//------------------------------------------------------- pdf generation ---
public boolean canEmbedForEditting()
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
try {
Permission thePerm = this.getFontData ().getEmbeddingPermission(wasEmbedded());
return thePerm != Permission.RESTRICTED && thePerm != Permission.PREVIEW_AND_PRINT; }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public boolean canEmbedForPrintAndPreview()
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
try {
return this.getFontData ().getEmbeddingPermission(wasEmbedded()) != Permission.RESTRICTED; }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
/** Create a subset for this font.
*/
public Subset createSubset ()
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
try {
return this.getFontData ().createSubset (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
//--------------------------------------------------------------------------
public FontData getFontData ()
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
try {
return this.retrieveFontData (); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public SWFFont4Description getSWFFont4Description ()
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
try {
return retrieveFontData ().getSWFFont4Description(wasEmbedded()); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public SWFFontDescription getSWFFontDescription ()
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
try {
return retrieveFontData ().getSWFFontDescription(wasEmbedded()); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public PDFFontDescription getPDFFontDescription ()
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
try {
return retrieveFontData ().getPDFFontDescription (this); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public XDCFontDescription getXDCFontDescription ()
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
try {
return retrieveFontData ().getXDCFontDescription (this); }
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public PostscriptFontDescription[] getPostscriptFontDescription ()
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
try {
return (PostscriptFontDescription[])getFontDescription(PostscriptFontDescription[].class.getSimpleName());
}
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public CSS20FontDescription[] getCSS20FontDescription ()
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
try {
return (CSS20FontDescription[])getFontDescription(CSS20FontDescription[].class.getSimpleName());
}
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public CSS20FontDescription getPreferredCSS20FontDescription()
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
try {
return (CSS20FontDescription)getFontDescription(CSS20FontDescription.class.getSimpleName());
}
catch (InvalidFontException e) {
e.initFont (this);
throw e; }
catch (UnsupportedFontException e) {
e.initFont (this);
throw e; }
}
public FXGFontDescription[] getFXGFontDescription()
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
return getFXGFontDescription(null, null);
}
public FXGFontDescription[] getFXGFontDescription(Platform platform)
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
return getFXGFontDescription(platform, null);
}
public FXGFontDescription[] getFXGFontDescription(Platform platform, ULocale locale)
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
try {
FXGFontDescription[] fxgDesc = (FXGFontDescription[])getFontDescription(FXGFontDescription[].class.getSimpleName());
if (platform == null && locale == null)
return fxgDesc;
int itemCount = 0;
for (int i = 0; i < fxgDesc.length; i++) {
if ((platform == null || platform == fxgDesc[i].getPlatform()) && (locale == null || locale == fxgDesc[i].getLocale()))
itemCount++;
}
FXGFontDescription[] fxgCopy = new FXGFontDescription[itemCount];
itemCount = 0;
for (int i = 0; i < fxgDesc.length; i++) {
if ((platform == null || platform == fxgDesc[i].getPlatform()) && (locale == null || locale == fxgDesc[i].getLocale()))
fxgCopy[itemCount++] = fxgDesc[i];
}
return fxgCopy;
} catch (InvalidFontException e) {
e.initFont (this);
throw e;
} catch (UnsupportedFontException e) {
e.initFont (this);
throw e;
}
}
public PlatformFontDescription[] getPlatformFontDescription()
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
return getPlatformFontDescription(null, null);
}
public PlatformFontDescription[] getPlatformFontDescription(Platform platform)
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
return getPlatformFontDescription(platform, null);
}
public PlatformFontDescription[] getPlatformFontDescription(Platform platform, ULocale locale)
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
try {
PlatformFontDescription[] platDesc = (PlatformFontDescription[])getFontDescription(PlatformFontDescription[].class.getSimpleName());
if (platform == null && locale == null)
return platDesc;
int itemCount = 0;
for (int i = 0; i < platDesc.length; i++) {
if ((platform == null || platform == platDesc[i].getPlatform()) && (locale == null || locale == platDesc[i].getLocale()))
itemCount++;
}
PlatformFontDescription[] platCopy = new PlatformFontDescription[itemCount];
itemCount = 0;
for (int i = 0; i < platDesc.length; i++) {
if ((platform == null || platform == platDesc[i].getPlatform()) && (locale == null || locale == platDesc[i].getLocale()))
platCopy[itemCount++] = platDesc[i];
}
return platCopy;
} catch (InvalidFontException e) {
e.initFont (this);
throw e;
} catch (UnsupportedFontException e) {
e.initFont (this);
throw e;
}
}
/**
* The new font cache support code starts here, though some code above here has been reworked also.
* The cached CacheSupportInfo of a font is used to allow the IntelligentResolver to operate entirely
* from cached data without have to re-parse the font.
*/
public CacheSupportInfo getCacheSupportInfo()
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
try {
return (CacheSupportInfo)getFontDescription(CacheSupportInfo.class.getSimpleName());
} catch (InvalidFontException e) {
e.initFont(this);
throw e;
} catch (UnsupportedFontException e) {
e.initFont(this);
throw e;
}
}
/**
* This is the hook for fetching or generating known description types and then storing
* them in the cache for future reference.
*/
private Object getFontDescription(String type)
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
Object cacheDes = getCachedFontDescription(type);
if (cacheDes == null) {
if (type.equals("CacheSupportInfo"))
cacheDes = retrieveFontData().getCacheSupportInfo();
else if (type.equals("CSS20FontDescription"))
cacheDes = retrieveFontData().getPreferredCSS20FontDescription();
else if (type.equals("CSS20FontDescription[]"))
cacheDes = retrieveFontData().getCSS20FontDescription();
else if (type.equals("FXGFontDescription[]"))
cacheDes = retrieveFontData().getFXGFontDescription(null, null);
else if (type.equals("PlatformFontDescription[]"))
cacheDes = retrieveFontData().getPlatformFontDescription(null, null);
else if (type.equals("PostscriptFontDescription[]"))
cacheDes = retrieveFontData().getPostscriptFontDescription();
else
throw new RuntimeException("Undefined font description class");
if (cacheDes != null)
setCachedFontDescription(type, cacheDes);
}
return cacheDes;
}
public abstract String getCanonicalPath();
public abstract long getLength();
public abstract long getLastModified();
public abstract Object getCachedFontDescription(String key);
public abstract Map getCachedFontDescriptionMap();
public abstract void setCachedFontDescription(String key, Object value);
/**
* Tells whether this font originally was embedded in a document.
* @return true iff the font was embedded according to our client.
*/
public boolean wasEmbedded() { return false; }
public abstract int hashCode();
public abstract boolean equals(Object obj);
public abstract String toString();
protected abstract FontData retrieveFontData ()
throws InvalidFontException, UnsupportedFontException, FontLoadingException;
}