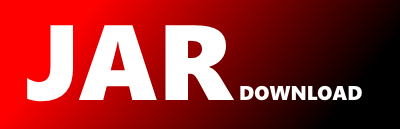
com.adobe.fontengine.font.HintedOutlineConsumer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: HintedOutlineConsumer.java
*
* ****************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font;
/**
* Receives information about a parsed hinted charstring. All coordinates are
* absolute in character space units. To get to a 1 ppem coordinate, compute
* [x y] * [font.getMetricsMatrix()]
*/
public interface HintedOutlineConsumer extends OutlineConsumer{
/**
* A stem command was seen.
* @param edge1 The bottom/left edge.
* @param edge2 The top/right edge. If isCounter is false and the stem is
* a topmost, rightmost, bottommost, or
* leftmost edge, the order of the edges is reversed (ie edge1 > edge2). If
* isCounter is true and the stem is the last counter in the current counter group,
* the order of the edges is reversed (ie edge1 > edge2).
* @param doHintSubstitution If isCounter is false, hint substitution must
* occur at this point iff doHintSubstitution is true.
* Else iff doHintSubstitution is true and isCounter is true, a new counter
* group must be started at this point.
* @param isVertical true iff the command was a vertical stem/counter.
* @param isCounter true iff the stem should be added to the current counter group.
*/
public void stem(double edge1, double edge2, boolean doHintSubstitution, boolean isVertical, boolean isCounter);
/**
* A hstem3 or vstem3 command was seen. The relevant edges are returned
*
* @param doHintSubstitution If true, hint substitution must occur at this point.
* @param isVertical true iff this command is a vstem3
*/
public void stem3(double edge1, double edge2,
double edge3, double edge4,
double edge5, double edge6,
boolean doHintSubstitution,
boolean isVertical);
/**
* A command was seen that says that global coloring applies to this glyph.
* One pass global coloring should be used if
* <@link HintedOutlineConsumer#noCounters()> is called or if a counter
* hint is associated with this glyph. Otherwise, two pass global coloring
* should be used.
*/
public void globalColorOn();
/**
* A command was seen that says that the glyph has no counters.
*/
public void noCounters();
/**
* A command was seen that says that no hints should be applied to the next part of the
* glyph.
*/
public void noHints();
/**
* The advance width of the glyph in the x direction
* @param wx the advance width
* @return true if charstring processing should continue. false otherwise.
*/
public boolean width(double wx);
/** A closepath command was seen. Note that closepath does NOT update the current point. */
public void closepath();
/** A flex command was seen */
public void flex(double depth,
double x1, double y1,
double x2, double y2,
double x3, double y3,
double x4, double y4,
double x5, double y5,
double x6, double y6);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy