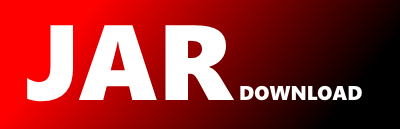
com.adobe.fontengine.font.cff.CFFScaler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: CFFScaler.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2006 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font.cff;
import com.adobe.fontengine.font.BitmapConsumer;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.Matrix;
import com.adobe.fontengine.font.OutlineConsumer;
import com.adobe.fontengine.font.OutlineConsumer2;
import com.adobe.fontengine.font.OutlineConsumerAdapter;
import com.adobe.fontengine.font.Scaler;
import com.adobe.fontengine.font.ScalerDebugger;
import com.adobe.fontengine.font.ScanConverter;
import com.adobe.fontengine.font.UnsupportedFontException;
/** A {@link Scaler} for {@link CFFFont}s.
*
* Currently does not interpret hints.
*/
public class CFFScaler implements Scaler {
protected final CFFFont font;
protected final ScanConverter scanConverter;
protected Matrix emToPixel;
public CFFScaler (CFFFont font, ScanConverter scanConverter) {
this.font = font;
this.scanConverter = scanConverter;
}
public void setScale (double pointSize, double ppemX, double ppemY,
double dX, double dY)
throws InvalidFontException, UnsupportedFontException {
emToPixel = new Matrix (ppemX, 0, 0, ppemY, dX, dY);
}
public void getOutline (int gid, OutlineConsumer outlineConsumer)
throws UnsupportedFontException, InvalidFontException {
font.getGlyphOutline (gid, outlineConsumer);
}
public void getBitmap (int gid, BitmapConsumer bitmapConsumer)
throws UnsupportedFontException, InvalidFontException {
if (ScalerDebugger.debugOn && debugger != null) {
OutlineConsumer2 x = debugger.getCFFOutlineConsumer ();
if (x != null) {
OutlineConsumerAdapter adapter = new OutlineConsumerAdapter (x);
adapter.setEmToPixelMatrix (emToPixel);
adapter.startOutline ();
font.getGlyphOutline (gid, adapter);
adapter.endOutline (); }}
OutlineConsumer2 consumer = scanConverter.getOutlineConsumer2 ();
consumer.startOutline ();
OutlineConsumerAdapter adapter = new OutlineConsumerAdapter (consumer);
adapter.setEmToPixelMatrix (emToPixel);
adapter.startOutline ();
font.getGlyphOutline (gid, adapter);
adapter.endOutline ();
scanConverter.getBitmap (bitmapConsumer);
}
//----------------------------------------------------------------------------
protected ScalerDebugger debugger;
public void setDebugger (ScalerDebugger debugger) {
this.debugger = debugger;
scanConverter.setDebugger (debugger);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy