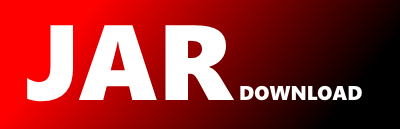
com.adobe.fontengine.font.cff.Index Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: Index.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font.cff;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.UnsupportedFontException;
import com.adobe.fontengine.font.cff.CFFByteArray.CFFByteArrayBuilder;
/** Represents an INDEX structure.
*/
class Index {
/* Our strategy is to entirely parse an Index at construction time. We
* still need to retain some access to the bits to deliver them (this
* is particularly true for superclasses of this one, such as NameIndex). */
/** The container for our bytes. */
public final CFFByteArray data;
/** The offset of our bytes in data
. */
public final int offset;
/** The size of the INDEX structure, in bytes. */
protected final int size;
/** The number of entries in the index. */
protected final int entryCount;
/** The offsets of the entries, relative to the beginning of the
* data
array. If there are no entries, entryOffsets
* is undefined. Otherwise, this array has entryCount + 1
* elements, with the last entry being the offset of the byte following
* the last byte of the last entry.
*/
protected final int[] entryOffsets;
/** Construct a Index
from a CFFByteArray
.
* @param data the CFFByteArray to get data from
* @param offset the offset of the first byte in data
*/
Index (CFFByteArray data, int offset)
throws InvalidFontException, UnsupportedFontException {
this.data = data;
this.offset = offset;
entryCount = data.getcard16 (offset + 0);
entryOffsets = new int [entryCount + 1];
if (entryCount == 0) {
size = 2;
return; }
int offSize = data.getOffSize (offset + 2);
int firstDataByteOffset = offset + 3 + offSize * (entryCount + 1);
for (int i = 0; i < entryCount + 1; i++) {
entryOffsets [i] = firstDataByteOffset
+ data.getOffset (offset + 3 + offSize * i, offSize,
"INDEX offset too big")
- 1; }
size = entryOffsets [entryCount] - offset;
}
/** The size, in bytes, of this INDEX in the data.*/
public int size () {
return size;
}
/** The number of entries in the INDEX.
*/
public int getCount () {
return entryCount;
}
/** The offset, in data, of entry
.
*/
public int offsetOf (int entry) {
return entryOffsets [entry];
}
/** The offset, in data, of the first byte following entry
.
*/
public int offsetFollowing (int entry) {
return entryOffsets [entry + 1];
}
/** The size, in bytes, of entry
.
*/
public int sizeOf (int entry) {
return entryOffsets [entry + 1] - entryOffsets [entry];
}
public void stream (CFFByteArrayBuilder bb) throws InvalidFontException {
bb.addBytes (data, offset, size);
}
static public class Cursor {
int base;
int offset; }
static public Cursor startIndex (CFFByteArrayBuilder bb, int n) {
if (n == 0) {
bb.addCard16 (0);
return null; }
bb.addCard16 (n);
bb.addCard8 (4);
bb.addCard32 (1);
Cursor cursor = new Cursor ();
cursor.offset = bb.getSize();
for (int i = 0; i < n; i++) {
bb.addCard32 (1); }
cursor.base = bb.getSize() - 1;
return cursor;
}
static public Cursor elementEntered (CFFByteArrayBuilder bb, Cursor cursor) {
bb.setCard32 (cursor.offset, bb.getSize() - cursor.base);
cursor.offset += 4;
return cursor;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy