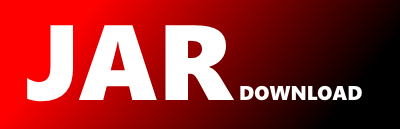
com.adobe.fontengine.font.mac.sfntResourceHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.fontengine.font.mac;
import java.io.InputStream;
import java.util.HashMap;
import java.util.Map;
import com.adobe.fontengine.font.Font;
import com.adobe.fontengine.font.FontLoadingException;
import com.adobe.fontengine.fontmanagement.FontLoader;
import com.adobe.internal.mac.resource.BasicResourceHandler;
import com.adobe.internal.mac.resource.ResourceParser.ResourceEntry;
public class sfntResourceHandler extends BasicResourceHandler
{
public static class SfntResource
{
private String name;
private int script;
private long size;
private int id;
private Font font;
private Exception exception;
private SfntResource(int id, String name, int script, long size)
{
this.size = size;
this.id = id;
this.name = name;
this.script = script;
}
public long getSize()
{
return this.size;
}
public int getID()
{
return this.id;
}
public String getName()
{
return this.name;
}
public int getScriptCode()
{
return this.script;
}
void setFont(Font font)
{
this.font = font;
}
public Font getFont()
{
return this.font;
}
void setException(Exception e)
{
this.exception = e;
}
public Exception getException()
{
return this.exception;
}
public String toString()
{
StringBuffer sb = new StringBuffer();
sb.append("fontID = " + this.id);
sb.append(", name = " + this.name);
sb.append(", size = " + this.size);
sb.append(", font = " + this.font);
return sb.toString();
}
}
private static final byte[] sfnt = {'s', 'f', 'n', 't'};
FontLoader loader = new FontLoader();
Map resources = new HashMap();
int idToCreate = -1;
boolean createAllFonts = true;
public sfntResourceHandler()
{
super(sfnt);
}
public sfntResourceHandler(int idToCreate)
{
this();
this.idToCreate = idToCreate;
this.createAllFonts = false;
}
public void handleResource(ResourceEntry entry, long length, InputStream stream)
{
// System.out.println("@@@ Resource Handler Plugin for type " + new String(handlerType));
// System.out.println("\ttype = " + new String(type));
// System.out.println("\tattributes = " + attributes);
// System.out.println("\tname = " + name);
// System.out.println("\tlength = " + length);
if (this.createAllFonts || this.idToCreate == entry.getID())
{
SfntResource resource = new SfntResource(entry.getID(), entry.getName(), entry.getScriptCode(), length);
Font font = null;
try {
font = loader.load(stream, (int) length, false);
} catch (FontLoadingException e) {
resource.setException(e);
}
resource.setFont(font);
resources.put(new Integer(entry.getID()), resource);
}
}
public Map getResources()
{
return this.resources;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy