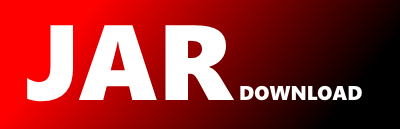
com.adobe.fontengine.font.postscript.Token Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: Token.java
*
* ****************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font.postscript;
/**
* A postscript token. Generally, this is what is a parsed unit according to the Tokenizer class.
*
* Synchronization
*
* This class is NOT threadsafe. Multiple instances can safely
* coexist without threadsafety issues, but each must only be accessed
* from one thread (or must be guarded by the client).
*/
final public class Token {
public TokenType tokenType;
public byte[] buff;
public int tokenLength;
Token()
{
tokenType = TokenType.kOPERATOR;
buff = new byte[256];
tokenLength = 0;
}
public boolean matches(byte[] tokenToFind)
{
if (tokenLength == tokenToFind.length
&& SubArrays.arrayCompare(buff, 0, tokenToFind, 0, tokenToFind.length))
return true;
return false;
}
public boolean isEOL()
{
return (tokenLength == 1 && buff[0] == -1);
}
public String stringTokenToString(int startPos, int endPos)
{
char[] chars = new char [endPos - startPos];
for (int i = startPos; i < endPos; i++) {
chars [i-startPos] = (char) buff[i]; }
return new String (chars);
}
/**
* convert an integer held in this token and return it.
* @param pos the position in this token to begin parsing.
* @return the parsed integer
*/
public int convertInteger(int pos)
{
int base = 10;
int value = 0;
boolean neg = buff[pos] == '-';
if (Tokenizer.isSign(buff[pos]))
pos++; /* Skip leading sign */
do
if (buff[pos] == '#')
{
/* It's a radix number so change base */
base = value;
value = 0;
}
else
value = value*base + Tokenizer.digit[(buff[pos])];
while (++pos < tokenLength &&
(Tokenizer.digit[(buff[pos])] != 99 || (buff[pos] == '#')));
return neg? -value: value;
}
/*
// this is intended for debugging purposes only since
// the conversion from bytes to a String is going to
// give you random unicode if we are looking at binary data.
void print()
{
String buffData;
for (int i = tokenLength; i < buff.length; i++)
buff[i] = 0;
buffData = new String(buff);
System.out.print(tokenType.toString());
System.out.print(" ");
System.out.println(buffData);
}
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy