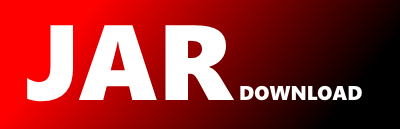
com.adobe.fontengine.fontmanagement.URLFont Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: URLFont.java
*
* ****************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.fontmanagement;
import java.io.File;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.lang.ref.SoftReference;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import com.adobe.fontengine.font.FontData;
import com.adobe.fontengine.font.FontImpl;
import com.adobe.fontengine.font.FontLoadingException;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.UnsupportedFontException;
import com.adobe.fontengine.font.type1.MetricFile;
import com.adobe.fontengine.font.type1.Type1Font;
/**
* A Font that can revive a FontData from a set of URLs
*/
class URLFont extends FontImpl {
static final long serialVersionUID = 1;
protected final URL outlineFileURL;
protected URL metricFileURL;
protected final int index;
protected String canonicalPath;
protected long length;
protected long lastModified;
protected ConcurrentHashMap descCache = new ConcurrentHashMap(16, 0.75f, 1);
transient protected SoftReference fontRef;
URLFont(URL outlineFileURL, int index)
{
this.outlineFileURL = outlineFileURL;
this.index = index;
this.fontRef = new SoftReference(null);
initCacheTags();
}
URLFont(URL outlineFileURL, int index, FontData font)
throws UnsupportedFontException
{
this.outlineFileURL = outlineFileURL;
this.index = index;
this.fontRef = new SoftReference(font);
initCacheTags();
}
void setMetricURL(URL metricFileURL, MetricFile f)
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
FontData data = retrieveFontData();
if (!(data instanceof Type1Font))
return;
else if (((Type1Font)data).setMetricFile(f))
this.metricFileURL = metricFileURL;
}
protected synchronized FontData retrieveFontData()
throws InvalidFontException, UnsupportedFontException, FontLoadingException
{
FontData font = null;
if ((font = (FontData) fontRef.get()) == null)
{
FontData[] arr;
try
{
arr = FontLoader.fromURL(outlineFileURL, metricFileURL);
} catch (IOException e)
{
throw new FontLoadingException(e);
}
font = arr[index];
fontRef = new SoftReference(font);
}
return font;
}
public int hashCode()
{
int hash = index;
hash ^= outlineFileURL.hashCode();
if (metricFileURL != null)
hash ^= metricFileURL.hashCode();
return hash;
}
public boolean equals(Object obj)
{
if (obj != null)
{
if (this == obj)
{
return true;
}
else if (obj instanceof URLFont)
{
URLFont otherURLFont = (URLFont) obj;
if (this.index == otherURLFont.index)
{
try {
if (!this.outlineFileURL.toURI().equals(otherURLFont.outlineFileURL.toURI()))
{
return false;
}
} catch (URISyntaxException e) {
return false;
}
if (this.metricFileURL == null && otherURLFont.metricFileURL == null)
{
return true;
}
try {
if ((this.metricFileURL == null) ||
otherURLFont.metricFileURL == null ||
!this.metricFileURL.toURI().equals(otherURLFont.metricFileURL.toURI()))
{
return false;
}
} catch (URISyntaxException e) {
return false;
}
return true;
}
}
}
return false;
}
public String toString()
{
return this.outlineFileURL.toString();
}
private void readObject(ObjectInputStream s) throws IOException, ClassNotFoundException
{
s.defaultReadObject();
this.fontRef = new SoftReference(null);
}
/* These are the cache support methods. ResourceFonts ARE supported for the
* cache so they should provide the necessary support to connect a URLFont
* to its underlying file and cached FontDescription objects.
*/
private void initCacheTags()
{
try {
try {
canonicalPath = outlineFileURL.toURI().getPath();
if (canonicalPath == null) {
throw new IOException("Cannot resolve path to file");
}
} catch (URISyntaxException e) {
throw new IOException(e.toString());
}
File file = new File(canonicalPath);
canonicalPath = file.getCanonicalPath();
length = file.length();
lastModified = file.lastModified();
} catch (IOException e) {
canonicalPath = null;
length = 0;
lastModified = 0;
}
}
public String getCanonicalPath()
{
return canonicalPath;
}
public long getLength()
{
return length;
}
public long getLastModified()
{
return lastModified;
}
public Object getCachedFontDescription(String key)
{
return descCache.get(key);
}
public Map getCachedFontDescriptionMap()
{
return descCache;
}
public void setCachedFontDescription(String key, Object value)
{
descCache.put(key, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy