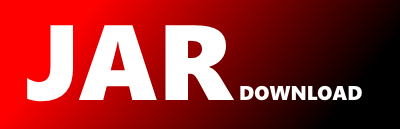
com.adobe.fontengine.fontmanagement.fxg.FXGFontResolver Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
*
* File: PSNameResolver.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.fontmanagement.fxg;
import java.io.Serializable;
import com.adobe.fontengine.font.Font;
import com.adobe.fontengine.font.FontLoadingException;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.UnsupportedFontException;
import com.adobe.fontengine.fontmanagement.FontResolutionPriority;
/**
* This interface provides access to the methods that a client can use to build a font set
* that is used
* to resolve FXG font names into actual {@link com.adobe.fontengine.font.Font} objects.
*
* Concurrency
*
* All classes that implement this interface are in general not guaranteed to be threadsafe. Specific
* implementations may offer tighter guarantees.
*/
public interface FXGFontResolver extends Serializable
{
/**
* Set the resolution mechanism that the FXGNameResolver should use to decide which
* font should be chosen when two fonts have the same FXG description.
* {@link com.adobe.fontengine.fontmanagement.FontResolutionPriority#INTELLIGENT_FIRST
* FontResolutionPriority.INTELLIGENT_FIRST} specifies
* that an "intelligent" determination is made about the fonts and the "better" of the two font is chosen.
* If the "intelligent" resolver can make no distinction between the fonts then the first font to have
* been added first is chosen.
*
{@link com.adobe.fontengine.fontmanagement.FontResolutionPriority#INTELLIGENT_LAST
* FontResolutionPriority.INTELLIGENT_LAST} specifies
* that an "intelligent" determination is made about the fonts and the "better" of the two font is chosen.
* If the "intelligent" resolver can make no distinction between the fonts then the first font to have
* been added last is chosen.
*
{@link com.adobe.fontengine.fontmanagement.FontResolutionPriority#FIRST
* FontResolutionPriority.FIRST} specifies
* the first font added to the font set that matches the FXG attributes is chosen.
*
{@link com.adobe.fontengine.fontmanagement.FontResolutionPriority#LAST
* FontResolutionPriority.LAST} specifies
* the last font added to the font set that matches the FXG attributes is chosen.
* @param priority The resolution mechanism to use
* @return The old resolution priority setting.
*/
public abstract FontResolutionPriority setResolutionPriority(FontResolutionPriority priority);
/**
* Adds a font to the set of fonts that are examined for resolving of FXG descriptions.
* If this font is indistinguishable from another font already in the font set then which
* of the two that is kept is determined by the settings of
* {@link com.adobe.fontengine.fontmanagement.FontResolutionPriority FontResolutionPriority}.
* @param font the font to add to the fonts used for FXG resolution
* @throws UnsupportedFontException
* @throws FontLoadingException
* @throws InvalidFontException
*/
public abstract void addFont(Font font)
throws UnsupportedFontException, InvalidFontException, FontLoadingException;
/**
* Adds a font to the set of fonts that are examined for resolving of FXG names.
* If this font's PlatformFontDescription is indistinguishable from another font already in
* the font set then which of the two that is kept is determined by the settings of
* {@link com.adobe.fontengine.fontmanagement.FontResolutionPriority FontResolutionPriority}.
*
Note that the PlatformFontDescription is NOT pushed down into the Font. It is only an
* alias in the database. Thus, calling
* {@link com.adobe.fontengine.font.Font#getFXGFontDescription() font.getFXGFontDescription()}
* will not return the description
* passed in through this method but will instead return the original FXG description
* of the font.
* @param FXGDesc the FXG description of the font
* @param font the font to add to the fonts used for FXG resolution
* @throws UnsupportedFontException
* @throws FontLoadingException
* @throws InvalidFontException
*/
public abstract void addFont(FXGFontDescription FXGDesc, Font font)
throws UnsupportedFontException, InvalidFontException, FontLoadingException;
/**
* @return true
if the font set is empty (has no fonts); false
otherwise
*/
public abstract boolean isEmpty();
/**
* Find a Font that matches the provided description. No analysis (e.g.,
* removal of a CMap extension) is done by this function.
* @param searchAttributes the FXG font attributes to search for
* @return the Font.
*/
public abstract Font findFont(FXGFontSearchAttributes searchAttributes);
}