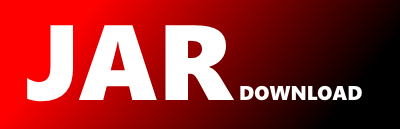
com.adobe.fontengine.inlineformatting.infontformatting.GlyphFormatter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: GlyphFormatter.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.inlineformatting.infontformatting;
import com.adobe.agl.util.ULocale;
import com.adobe.fontengine.font.FontData;
import com.adobe.fontengine.font.FontLoadingException;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.UnsupportedFontException;
import com.adobe.fontengine.font.opentype.OTSelectors;
import com.adobe.fontengine.font.opentype.OpenTypeFont;
import com.adobe.fontengine.font.opentype.Tag;
import com.adobe.fontengine.font.type1.Type1Font;
import com.adobe.fontengine.inlineformatting.AttributedRun;
import com.adobe.fontengine.inlineformatting.ElementAttribute;
final public class GlyphFormatter extends BaseFormatter {
//---------------------------------------------------------- OT formatting ---
protected boolean canFormatOT() {
return true;
}
protected int formatOT (OpenTypeFont otFont, AttributedRun run, int start, int limit, boolean shouldKern)
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
// Position the glyphs by advance
posFromAdvanceWidth (run, otFont, start, limit);
// Adjust the positions by GPOS
if (otFont.gpos != null) {
int scriptTag = getOTScriptTag ((Integer) run.getElementStyle (start, InFontFormatter.scriptAttribute));
int langTag = getOTLanguageTag ((ULocale) run.getElementStyle (start, ElementAttribute.locale));
int[][] gposLookups = LookupsCache.resolveFeatureTag (otFont.gpos, scriptTag, langTag, gposFeatures);
limit = otFont.gpos.applyLookups (gposLookups [0], run, start, limit, OTSelectors.everywhere, otFont.gdef); // mark
limit = otFont.gpos.applyLookups (gposLookups [1], run, start, limit, OTSelectors.everywhere, otFont.gdef); } // mkmk
else if (shouldKern && otFont.kern != null) {
applyKernTable(otFont, run, start, limit);
}
return limit;
}
private static final int[] gposFeatures = {
Tag.feature_mark, // 0
Tag.feature_mkmk, // 1
};
//---------------------------------------------------------- TT formatting ---
protected boolean canFormatTT() {
return true;
}
protected int formatTT (OpenTypeFont otFont, AttributedRun run, int start, int limit, boolean shouldKern)
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
posFromAdvanceWidth (run, otFont, start, limit);
if (shouldKern && otFont.kern != null) {
applyKernTable(otFont, run, start, limit);
}
return limit;
}
//---------------------------------------------------------- T1 formatting ---
protected boolean canFormatT1() {
return true;
}
protected int formatT1 (Type1Font t1Font, AttributedRun run, int start, int limit, boolean shouldKern)
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
posFromAdvanceWidth (run, t1Font, start, limit);
if (shouldKern)
{
for (int i = start; i < limit - 1; i++) {
double kernValue = t1Font.getKernValue (run.elementAt (i), run.elementAt (i+1));
run.adjustPlacementAndAdvance (i, 0, 0, kernValue, 0); }
}
return limit;
}
//----------------------------------------------------- Generic formatting ---
protected boolean canFormatGeneric() {
return true;
}
protected int formatGeneric (FontData fontData, AttributedRun run, int start, int limit, boolean shouldKern)
throws InvalidFontException, UnsupportedFontException, FontLoadingException {
posFromAdvanceWidth (run, fontData, start, limit);
return limit;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy