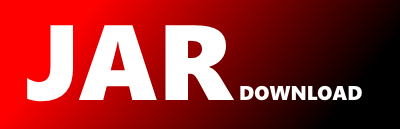
com.adobe.fontengine.math.F2Dot14 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: F2Dot14.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.math;
import com.adobe.agl.text.DecimalFormat;
/** Constants and operations on fixed 2.14 numbers.
*
* The value v is represented by the int
value
* 2^14 * v .
*/
public final class F2Dot14 {
public static final int /*2.14*/ ZERO = 0x00;
public static final int /*2.14*/ ONE = 0x4000;
public static final int /*2.14*/ ONE_SIXTEENTH = 0x400;
private static int clamp (long x) {
if (x > 32767) {
return 32767; }
else if (x < -32768) {
return -32768; }
else {
return (int) x; }
}
public static int /*2.14*/ multiply (int /*2.14*/ v1, int /*2.14*/ v2) {
return clamp (((((long) v1 * (long) v2) >> 13) + 1) >> 1);
}
public static int /*2.14*/ square (int /*2.14*/ v) {
return multiply (v, v);
}
public static int /*2.14*/ fromDouble (double v) {
return (int) (v * ONE);
}
public static double toDouble (int /*2.14*/ v) {
return ((double) v) / ONE;
}
private final static DecimalFormat df = new DecimalFormat ("0.###");
public static String toString (int /*2.14*/ v) {
return df.format (toDouble (v));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy