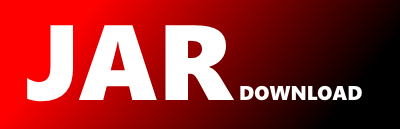
com.adobe.granite.auth.ims.IMSInstance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2017 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.auth.ims;
public final class IMSInstance {
private final String instanceId;
private final String owningEntity;
private final String serviceCode;
public IMSInstance(String instanceId, String owningEntity, String serviceCode) {
if (owningEntity == null || serviceCode == null) {
throw new IllegalArgumentException(String.format("null owningEntity or serviceCode are not allowed (instanceId: %s, owningEntity: %s, serviceCode: %s", instanceId, owningEntity, serviceCode));
}
this.instanceId = instanceId;
this.owningEntity = owningEntity;
this.serviceCode = serviceCode;
}
public String getInstanceId() {
return instanceId;
}
public String getOwningEntity() {
return owningEntity;
}
public String getServiceCode() {
return serviceCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
IMSInstance other = (IMSInstance) obj;
if (instanceId == null) {
if (other.instanceId != null)
return false;
} else if (!instanceId.equals(other.instanceId))
return false;
if (owningEntity == null) {
if (other.owningEntity != null)
return false;
} else if (!owningEntity.equals(other.owningEntity))
return false;
if (serviceCode == null) {
if (other.serviceCode != null)
return false;
} else if (!serviceCode.equals(other.serviceCode))
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((instanceId == null) ? 0 : instanceId.hashCode());
result = prime * result + ((owningEntity == null) ? 0 : owningEntity.hashCode());
result = prime * result + ((serviceCode == null) ? 0 : serviceCode.hashCode());
return result;
}
@Override
public String toString() {
return "IMSInstance{" +
"instanceId='" + instanceId + '\'' +
", owningEntity='" + owningEntity + '\'' +
", serviceCode='" + serviceCode + '\'' +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy