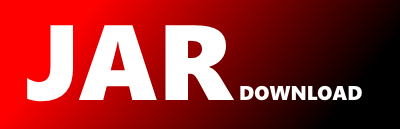
com.adobe.granite.haf.api.ApiResponseBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2016 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.haf.api;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.servlets.post.Modification;
import org.osgi.annotation.versioning.ProviderType;
/**
* An interface for creating ApiResponse instances. To create an ApiResponseBuilder instance use
* ApiResponseBuilderFactory.createBuilder()
*/
@ProviderType
public interface ApiResponseBuilder {
/**
* Set the HTTP status
* @param code The status code
* @param message The status message
* @return This ApiResponseBuilder instance
*/
ApiResponseBuilder setStatus(Integer code, String message);
/**
* Set an HTTP header to be used in the response. If a header with this key already exists it will be replaced
* with the value specified in this method.
* @param key The HTTP header name
* @param value The HTTP header value
* @return This ApiResponseBuilder instance
*/
ApiResponseBuilder withHeader(String key, String value);
/**
* Set an HTTP header with multiple values. If a header with this key already exists it will be replaced with
* the values specified in this method.
* @param key The HTTP header name
* @param values The HTTP header values
* @return This ApiResponseBuilder instance
*/
ApiResponseBuilder withHeader(String key, String[] values);
/**
* Set an entity to be used as the response body. The entity must be a Resource that can be interpreted by the
* HTTP API Framework
* @param entity A resource to be used as the entity
* @return This ApiResponseBuilder instance
*/
ApiResponseBuilder setEntity(Resource entity);
/**
* Set the body to be returned in the response. The body must be an instance of CharSequence or a byte[]
* @param body An instance of CharSequence or a byte[] to be used in the body of the response.
* @return This ApiResponseBuilder instance
*/
ApiResponseBuilder setBody(Object body);
/**
* Set the content type of the body.
* @param contentType The content type.
* @return This ApiResponseBuilder instance.
*/
ApiResponseBuilder setContentType(String contentType);
/**
* Add a Modification to be returned in the changes section of the response.
* @param modification The Modification to be added.
* @return This ApiResponseBuilder
*/
ApiResponseBuilder withChange(Modification modification);
/**
* Build the ApiResponse instance with the values specified in this ApiRespoonseBuilder.
* @return The ApiResponse instance
*/
ApiResponse build();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy