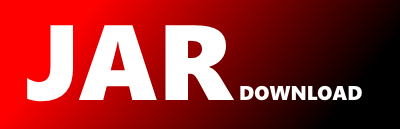
com.adobe.granite.ui.components.Options Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2016 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.ui.components;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
/**
* The class representing the options during Sling include.
*/
public class Options {
private Tag tag;
private boolean rootField = true;
/**
* Returns the tag.
*
* @return the tag
*/
@CheckForNull
public Tag tag() {
return tag;
}
/**
* Sets the tag.
*
* @param tag
* the tag
* @return the options
*/
@Nonnull
public Options tag(@CheckForNull Tag tag) {
this.tag = tag;
return this;
}
/**
* Returns {@code true} if the renderer (the field) should render itself as root
* field. See {@link #rootField(boolean)} for details.
*
* @return {@code true} if the renderer (the field) should render itself as root
* field.
*/
public boolean rootField() {
return rootField;
}
/**
* Sets {@code true} to make the renderer (the field) should render itself as
* root field; {@code false} otherwise.
*
* A root field is a field that acts in its own context, instead of as part of a
* composite field. For example, sizing field consists of weight and height
* fields. So sizing field is a composite field and wants to leverage the
* existing number field for width and height. In this case when sizing field is
* including (
* {@link ComponentHelper#include(org.apache.sling.api.resource.Resource, ComponentHelper.Options)})
* the number field, it should set this option as {@code false}.
*
* The field implementation is free to interpret the exact behaviour of
* root/non-root field. Most likely scenario, the root field will handle it own
* sizing state (e.g. inline-block/block state), while non root field will not,
* where the parent composite field is managing it.
*
* @param flag
* the flag
* @return this instance
*/
@Nonnull
public Options rootField(boolean flag) {
rootField = flag;
return this;
}
}