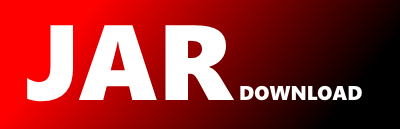
com.adobe.internal.io.CountingInputStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/**
*
*/
package com.adobe.internal.io;
import java.io.FilterInputStream;
import java.io.IOException;
import java.io.InputStream;
/**
* A FilterInputStream
wrapper that counts the number of bytes read from the stream or skipped over
* since the creation of this wrapper.
*
*/
public class CountingInputStream extends FilterInputStream
{
private long offset;
/**
* @param is
*/
public CountingInputStream(InputStream is)
{
super(is);
}
/**
* Reads the next byte of data from this input stream. The value byte is returned as an
* int in the range 0 to 255. If no byte is available because the end of the stream has
* been reached, the value -1 is returned. This method blocks until input data is available,
* the end of the stream is detected, or an exception is thrown.
* @return the next byte of data or -1
if the end of stream is reached
* @throws IOException if an I/O error occurs
*/
public int read() throws IOException
{
int b = super.read();
if (b != -1)
{
offset++;
}
return b;
}
/**
* Reads up to len bytes of data from this input stream into an array of bytes. This method
* blocks until some input is available.
* @param b the buffer into which the data is read
* @param off the start offset in the buffer to write the data
* @param len the maximum number of bytes to read
* @return the total number of bytes read into the buffer, or -1
if there
* is no more data because the end of the stream has been reached.
* @exception IOException if an I/O error occurs
*/
public int read(byte[] b, int off, int len) throws IOException
{
int bytesRead = super.read(b, off, len);
if (bytesRead > 0)
{
offset += bytesRead;
}
return bytesRead;
}
/**
* Reads up to byte.length bytes of data from this input stream into an array of bytes.
* This method blocks until some input is available.
* @return the total number of bytes read into the buffer, or -1
if there
* is no more data because the end of the stream has been reached.
* @exception IOException if an I/O error occurs
*/
public int read(byte[] b) throws IOException
{
int bytesRead = super.read(b);
if (bytesRead > 0)
{
offset += bytesRead;
}
return bytesRead;
}
/**
* Skips over and discards n bytes of data from the input stream. The skip method may, for a
* variety of reasons, end up skipping over some smaller number of bytes, possibly 0. The
* actual number of bytes skipped is returned.
* @param n the number of bytes to be skipped
* @return the actual number of bytes skipped
* @exception IOException if an I/O error occurs
*/
public long skip(long n) throws IOException
{
long bytesSkipped = super.skip(n);
if (bytesSkipped > 0)
{
offset += bytesSkipped;
}
return bytesSkipped;
}
/**
* Get the offset in the wrapped stream since the creation of this wrapper.
* @return the offset in bytes
*/
public long getOffset()
{
return this.offset;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy