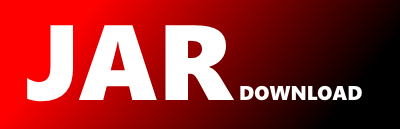
com.adobe.internal.util.ArrayMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/* ****************************************************************************
*
* File: ArrayMap.java
*
* ****************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
* ***************************************************************************/
package com.adobe.internal.util;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
/**
* @author itenenbo
*
* This class implements Map in a way that its iterators always return Map values
* in the same order.
* This class differs from TreeMap in that it does not require the keys to be
* Comparable. At the same time it requires twice as much memory as the TreeMap
* implementation requires. This is the price of getting this functionality.
*/
public class ArrayMap implements Map
{
public class Entry implements Map.Entry
{
int mMapPos;
Object mKey;
Object mValue;
Entry(int pos)
{
mMapPos = pos;
mKey = ArrayMap.this.mKeys.get(mMapPos);
mValue = ArrayMap.this.mValues.get(mMapPos);
}
public Object getKey()
{
return mKey;
}
public Object getValue()
{
return mValue;
}
public Object setValue(Object arg0)
{
Object oldValue = mValue;
mValue = arg0;
if (ArrayMap.this.containsKey(mKey))
{
ArrayMap.this.put(mKey, arg0);
}
return oldValue;
}
}
private class ArrayKeySet implements Set
{
ArrayKeySet()
{
}
public int size()
{
return ArrayMap.this.size();
}
public void clear()
{
throw new UnsupportedOperationException();
}
public boolean isEmpty()
{
return ArrayMap.this.isEmpty();
}
public Object[] toArray()
{
return ArrayMap.this.mKeys.toArray();
}
public boolean add(Object arg0)
{
throw new UnsupportedOperationException();
}
public boolean contains(Object arg0)
{
return ArrayMap.this.containsKey(arg0);
}
public boolean remove(Object arg0)
{
if (!ArrayMap.this.containsKey(arg0))
{
return false;
}
ArrayMap.this.remove(arg0);
return true;
}
public boolean addAll(Collection arg0)
{
throw new UnsupportedOperationException();
}
public boolean containsAll(Collection arg0)
{
return ArrayMap.this.mKeys.containsAll(arg0);
}
public boolean removeAll(Collection arg0)
{
throw new UnsupportedOperationException();
}
public boolean retainAll(Collection arg0)
{
throw new UnsupportedOperationException();
}
public Iterator iterator()
{
return new KeyIterator();
}
public Object[] toArray(Object[] arg0)
{
return ArrayMap.this.mKeys.toArray(arg0);
}
}
private class KeyIterator implements Iterator
{
int mPos;
KeyIterator()
{
mPos = 0;
}
public boolean hasNext()
{
return mPos < ArrayMap.this.size();
}
public Object next()
{
return ArrayMap.this.mKeys.get(mPos++);
}
public void remove()
{
if (mPos < 1)
{
throw new IllegalStateException();
}
Object key = ArrayMap.this.mKeys.get(--mPos);
ArrayMap.this.remove(key);
}
}
private class EntryIterator implements Iterator
{
int mPos;
EntryIterator()
{
mPos = 0;
}
public boolean hasNext()
{
return mPos < ArrayMap.this.size();
}
public Object next()
{
return new Entry(mPos++);
}
public void remove()
{
if (mPos < 1)
{
throw new IllegalStateException();
}
Entry curEntry = new Entry(--mPos);
Object key = curEntry.getKey();
ArrayMap.this.remove(key);
}
}
private class ArrayEntrySet implements Set
{
ArrayEntrySet()
{
}
public int size()
{
return ArrayMap.this.size();
}
public void clear()
{
throw new UnsupportedOperationException();
}
public boolean isEmpty()
{
return ArrayMap.this.isEmpty();
}
public Object[] toArray()
{
Object[] entries = new Object[ArrayMap.this.size()];
int posInd;
for (posInd = 0; posInd < ArrayMap.this.size(); posInd++)
{
entries[posInd] = new ArrayMap.Entry(posInd);
}
return entries;
}
public boolean add(Object arg0)
{
throw new UnsupportedOperationException();
}
public boolean contains(Object arg0)
{
if (!(arg0 instanceof Entry))
return false;
Object entryKey = ((Entry) arg0).getKey();
Object mapKey = ArrayMap.this.get(entryKey);
if (mapKey == null)
{
return false;
}
return ArrayMap.this.get(mapKey).equals(((Entry) arg0).getValue());
}
public boolean remove(Object arg0)
{
if (!(arg0 instanceof Entry))
{
return false;
}
Object key = ((Entry) arg0).getKey();
if (!ArrayMap.this.containsKey(key))
{
return false;
}
ArrayMap.this.remove(arg0);
return true;
}
public boolean addAll(Collection arg0)
{
throw new UnsupportedOperationException();
}
public boolean containsAll(Collection arg0)
{
if (arg0 == null)
{
return false;
}
Iterator entryIterator = arg0.iterator();
while (entryIterator.hasNext())
{
if (!contains(entryIterator.next()))
{
return false;
}
}
return true;
}
public boolean removeAll(Collection arg0)
{
throw new UnsupportedOperationException();
}
public boolean retainAll(Collection arg0)
{
throw new UnsupportedOperationException();
}
public Iterator iterator()
{
return new EntryIterator();
}
public Object[] toArray(Object[] arg0)
{
throw new UnsupportedOperationException();
}
}
private ArrayList mKeys;
private ArrayList mValues;
private HashMap mKeysMapping;
private int mCurIndex;
public ArrayMap(int size)
{
mKeys = new ArrayList(size);
mValues = new ArrayList(size);
mKeysMapping = new HashMap(size * 2);
mCurIndex = 0;
}
public ArrayMap()
{
this(100);
}
public int size()
{
return mCurIndex;
}
public void clear()
{
mCurIndex = 0;
mKeys.clear();
mValues.clear();
mKeysMapping.clear();
}
public boolean isEmpty()
{
return mCurIndex == 0;
}
public boolean containsKey(Object arg0)
{
return mKeysMapping.containsKey(arg0);
}
public boolean containsValue(Object arg0)
{
return mValues.contains(arg0);
}
public Collection values()
{
return mValues;
}
public void putAll(Map arg0)
{
Iterator mapIter = arg0.entrySet().iterator();
while (mapIter.hasNext())
{
Map.Entry curEntry = (Map.Entry) mapIter.next();
this.put(curEntry.getKey(), curEntry.getValue());
}
}
public Set entrySet()
{
return new ArrayEntrySet();
}
public Set keySet()
{
return new ArrayKeySet();
}
public Object get(Object arg0)
{
Integer pos = (Integer) mKeysMapping.get(arg0);
if (pos == null)
return null;
return mValues.get(pos.intValue());
}
public Object remove(Object arg0)
{
Integer pos = (Integer) mKeysMapping.get(arg0);
if (pos == null)
{
return null;
}
Object oldValue = mValues.get(pos.intValue());
mKeysMapping.remove(arg0);
mKeys.remove(pos.intValue());
mValues.remove(pos.intValue());
mCurIndex--;
int mapInd;
for (mapInd = pos.intValue(); mapInd < mCurIndex; mapInd++)
{
mKeysMapping.put(mKeys.get(mapInd), Integer.valueOf(mapInd));
}
return oldValue;
}
public Object put(Object arg0, Object arg1)
{
Integer pos = (Integer) mKeysMapping.get(arg0);
Object oldValue = null;
if (pos != null)
{
oldValue = mValues.get(pos.intValue());
mValues.set(pos.intValue(), arg1);
}
else
{
Integer key = Integer.valueOf(mCurIndex);
mKeysMapping.put(arg0, key);
mKeys.add(mCurIndex, arg0);
mValues.add(mCurIndex++, arg1);
}
return oldValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy