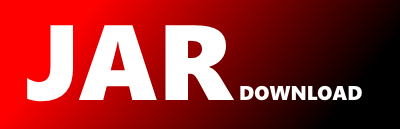
com.adobe.internal.util.ByteStringBuffer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: ByteStringBuffer.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.internal.util;
import java.io.Serializable;
/**
* @author sgill
*
*/
public class ByteStringBuffer implements ByteSequence, Comparable, Serializable
{
private byte[] value;
private int length;
/**
*
*/
private static final long serialVersionUID = -5059151845327279132L;
/**
*
*/
public ByteStringBuffer()
{
this(16);
}
/**
* @param length
*/
public ByteStringBuffer(int length)
{
this.value = new byte[length];
}
/* (non-Javadoc)
* @see com.adobe.internal.util.ByteSequence#byteAt(int)
*/
public byte byteAt(int index)
{
if ((index < 0) || (index >= this.length))
{
throw new StringIndexOutOfBoundsException(index);
}
return value[index];
}
/* (non-Javadoc)
* @see com.adobe.internal.util.ByteSequence#length()
*/
public int length()
{
return this.length;
}
/* (non-Javadoc)
* @see com.adobe.internal.util.ByteSequence#subSequence(int, int)
*/
public ByteSequence subSequence(int start, int end)
{
// TODO Auto-generated method stub
return null;
}
/**
* @param begin the first byte index to include in the substring
* @param end the last byte index to include in the substring
* @return substring of this byte string from begin
to end
*/
public ByteString substring(int begin, int end)
{
if (begin < 0) {
throw new StringIndexOutOfBoundsException(begin);
}
if (end > this.length) {
throw new StringIndexOutOfBoundsException(end);
}
if (begin > end) {
throw new StringIndexOutOfBoundsException(end - begin);
}
return new ByteString(this.value, begin, end - begin);
}
public void getBytes(int srcBegin, int srcEnd, byte dst[], int dstBegin)
{
try
{
System.arraycopy(this.value, srcBegin, dst, dstBegin, srcEnd - srcBegin);
} catch (IndexOutOfBoundsException e) {
StringIndexOutOfBoundsException newException = new StringIndexOutOfBoundsException();
newException.initCause(e);
throw newException;
}
}
/* (non-Javadoc)
* @see com.adobe.internal.util.ByteSequence#getBytes()
*/
public byte[] getBytes()
{
byte[] array = new byte[length];
System.arraycopy(this.value, 0, array, 0, this.length);
return array;
}
public void setByteAt(int index, byte b)
{
if ((index < 0) || (index >= this.length)) {
throw new StringIndexOutOfBoundsException(index);
}
this.value[index] = b;
}
public ByteStringBuffer append(byte b)
{
int newLength = this.length + 1;
if (newLength > this.value.length)
{
expandCapacity(newLength);
}
this.value[this.length++] = b;
return this;
}
public ByteStringBuffer append(byte[] b)
{
return this.append(b, 0, b.length);
}
public ByteStringBuffer append(byte[] b, int offset, int length)
{
if ((offset < 0) || (length < 0) || ((offset + length) > b.length))
{
throw new IndexOutOfBoundsException("Byte array index out of bounds.");
}
int newLength = this.length + length;
if (newLength > this.value.length)
{
expandCapacity(newLength);
}
System.arraycopy(this.value, this.length, b, offset, length);
this.length += length;
return this;
}
public void ensureCapacity(int minimumCapacity)
{
if (minimumCapacity > this.value.length)
{
this.expandCapacity(minimumCapacity);
}
}
private void expandCapacity(int minimumCapacity)
{
int newCapacity = (value.length + 1) * 2;
if (newCapacity < 0)
{
newCapacity = Integer.MAX_VALUE;
}
else if (minimumCapacity > newCapacity)
{
newCapacity = minimumCapacity;
}
byte newValue[] = new byte[newCapacity];
System.arraycopy(value, 0, newValue, 0, this.length);
value = newValue;
}
/* (non-Javadoc)
* @see java.lang.Comparable#compareTo(java.lang.Object)
*/
public int compareTo(Object otherObject)
{
return compareTo((ByteStringBuffer) otherObject);
}
/**
* This comparision is byte by byte and one byte string will be "lesser" or "greater" than
* the other based on the byte values at the first position in which they differ. If both
* byte strings are identical in all positions but one is longer than the other then longer
* string is "greater". Only if both byte strings are of the same length and have
* identical byte values in all positions will they be identical.
*
* @param otherByteString
* @return 0 if the argument byte string is equal to this byte string; a value less than 0 if
* this byte string is "less" than the argument byte string; and a value greater than 0 if this
* byte string is "greater" than the argument byte string
*/
public int compareTo(ByteStringBuffer otherByteString)
{
int count = Math.min(this.length, otherByteString.length);
for (int i = 0; i < count; i++)
{
byte b1 = this.value[i];
byte b2 = otherByteString.value[i];
if (b1 != b2)
{
return b1 - b2;
}
}
return this.length - otherByteString.length;
}
/* (non-Javadoc)
* @see java.lang.Object#equals(java.lang.Object)
*/
public boolean equals(Object otherObject)
{
if (this == otherObject)
{
return true;
}
if (otherObject instanceof ByteStringBuffer)
{
return (this.compareTo(otherObject) == 0);
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy