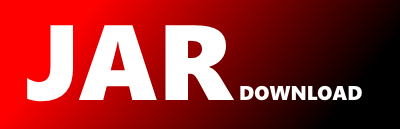
com.adobe.xfa.HostPseudoModelScript Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.ResId;
import java.util.ArrayList;
import java.util.List;
import java.util.StringTokenizer;
/**
* This class contains all the script functionality associated with the
* HostPseudoModel class. Broken out into a separate class for easier maintainability.
*
* @exclude from published api.
*/
public class HostPseudoModelScript extends PseudoModelScript {
protected static final ScriptTable moScriptTable = new ScriptTable(
PseudoModelScript.moScriptTable,
"hostPseudoModel",
new ScriptPropObj[] {
new ScriptPropObj(HostPseudoModelScript.class, "appType", "getAppType", null, Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_APPTYPE_DESC, XFA_IS_HOST_APPTYPE_PARAMDESC*/, 0),
new ScriptPropObj(HostPseudoModelScript.class, "currentPage", "getCurrentPage", "setCurrentPage", Arg.INTEGER, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_CURRENTPAGE_DESC, 0*/, 0),
new ScriptPropObj(HostPseudoModelScript.class, "language", "getLanguage", null, Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_LANGUAGE_DESC, XFA_IS_HOST_LANGUAGE_PARAMDESC*/, 0),
new ScriptPropObj(HostPseudoModelScript.class, "name", "getName", null, Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_NAME_DESC, 0*/, 0),
new ScriptPropObj(HostPseudoModelScript.class, "numPages", "getNumPages", null, Arg.INTEGER, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_NUMPAGES_DESC, 0*/, 0),
new ScriptPropObj(HostPseudoModelScript.class, "platform", "getPlatform", null, Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_PLATFORM_DESC, XFA_IS_HOST_PLATFORM_PARAMDESC*/, 0),
new ScriptPropObj(HostPseudoModelScript.class, "title", "getTitle", "setTitle", Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_TITLE_DESC, 0*/, 0),
new ScriptPropObj(HostPseudoModelScript.class, "variation", "getVariation", null, Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_VARIATION_DESC, XFA_IS_HOST_VARIATION_PARAMDESC*/, 0),
new ScriptPropObj(HostPseudoModelScript.class, "version", "getVersion", null, Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_VERSION_DESC, 0*/, 0),
new ScriptPropObj(HostPseudoModelScript.class, "calculationsEnabled", "getCalculationsEnabled", "setCalculationsEnabled", Arg.BOOL, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_CALCULATIONSENABLED_DESC, 0*/, 0),
new ScriptPropObj(HostPseudoModelScript.class, "validationsEnabled", "getValidationsEnabled", "setValidationsEnabled", Arg.BOOL, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_VALIDATIONSENABLED_DESC, 0*/, 0)
},
new ScriptFuncObj[] {
new ScriptFuncObj(HostPseudoModelScript.class, "beep", "beep", Arg.EMPTY,
new int[] { Arg.INTEGER/*, XFA_IS_HOST_BEEP_PARAM1 */ }, 0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_BEEP_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "currentDateTime", "getCurrentDateTime", Arg.STRING,
new int[] { }, 0, Schema.XFAVERSION_28, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_CURRENTDATETIME_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "documentInBatch", "getDocumentInBatch", Arg.INTEGER,
new int[] { }, 0, Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_DOCUMENTINBATCH_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "documentCountInBatch", "getDocumentCountInBatch", Arg.INTEGER,
new int[] { }, 0, Schema.XFAVERSION_25, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_DOCUMENTCOUNTINBATCH_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "exportData", "exportData", Arg.EMPTY,
new int[] { Arg.STRING, Arg.BOOL/*, XFA_IS_HOST_EXPORTDATA_PARAM1, XFA_IS_HOST_EXPORTDATA_PARAM2 */ },
0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_EXPORTDATA_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "gotoURL", "gotoURL", Arg.EMPTY,
new int[] { Arg.STRING, Arg.BOOL/*, XFA_IS_HOST_GOTOURL_PARAM1, XFA_IS_HOST_GOTOURL_PARAM2*/ },
1, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_GOTOURL_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "importData", "importData", Arg.EMPTY,
new int[] { Arg.STRING/*, XFA_IS_HOST_IMPORTDATA_PARAM1 */ },
0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_IMPORTDATA_DESC, 0,*/, "importDataPermsCheck", 0),
new ScriptFuncObj(HostPseudoModelScript.class, "messageBox", "messageBox", Arg.INTEGER,
new int[] { Arg.STRING, Arg.STRING, Arg.INTEGER, Arg.INTEGER/*, XFA_IS_HOST_MESSAGEBOX_PARAM1, XFA_IS_HOST_MESSAGEBOX_PARAM2, XFA_IS_HOST_MESSAGEBOX_PARAM3, XFA_IS_HOST_MESSAGEBOX_PARAM4 */ },
1, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_MESSAGEBOX_DESC, XFA_IS_HOST_MESSAGEBOX_RET, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "pageDown", "pageDown", Arg.EMPTY,
new int[] { }, 0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_PAGEDOWN_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "pageUp", "pageUp", Arg.EMPTY,
new int[] { }, 0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_PAGEUP_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "print", "print", Arg.EMPTY,
new int[] { Arg.BOOL, Arg.STRING, Arg.STRING, Arg.BOOL, Arg.BOOL, Arg.BOOL, Arg.BOOL, Arg.BOOL/*, XFA_IS_HOST_PRINT_PARAM1, XFA_IS_HOST_PRINT_PARAM2, XFA_IS_HOST_PRINT_PARAM3, XFA_IS_HOST_PRINT_PARAM4, XFA_IS_HOST_PRINT_PARAM5, XFA_IS_HOST_PRINT_PARAM6, XFA_IS_HOST_PRINT_PARAM7, XFA_IS_HOST_PRINT_PARAM8*/ }, 8, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_PRINT_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "response", "response", Arg.INVALID,
new int[] { Arg.STRING, Arg.STRING, Arg.STRING, Arg.BOOL/*, XFA_IS_HOST_RESPONSE_PARAM1, XFA_IS_HOST_RESPONSE_PARAM2, XFA_IS_HOST_RESPONSE_PARAM3, XFA_IS_HOST_RESPONSE_PARAM4*/ },
1, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_RESPONSE_DESC, XFA_IS_HOST_RESPONSE_RET, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "setFocus", "setFocus", Arg.EMPTY,
new int[] { Arg.INVALID /*, XFA_IS_HOST_SETFOCUS_PARAM1 */ },
0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_SETFOCUS_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "resetData", "resetData", Arg.EMPTY,
new int[] { Arg.STRING/*, XFA_IS_HOST_RESETDATA_PARAM1 */ },
0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_RESETDATA_DESC, 0*/, "resetDataPermsCheck", 0),
new ScriptFuncObj(HostPseudoModelScript.class, "openList", "openList", Arg.EMPTY,
new int[] { Arg.INVALID/*, XFA_IS_HOST_OPENLIST_PARAM1 */ },
1, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_HOST_OPENLIST_DESC, 0, null*/, 0),
new ScriptFuncObj(HostPseudoModelScript.class, "getFocus", "getFocus", Arg.OBJECT,
new int[] { }, 0, Schema.XFAVERSION_26, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_HOST_GETFOCUS_DESC, 0, null*/, 0)
}
);
public static ScriptTable getScriptTable() {
return moScriptTable;
}
public static void beep(Obj pObj, Arg oRetVal, Arg[] pArgs) {
if (pArgs.length == 1 )
((HostPseudoModel) pObj).beep(pArgs[0].getInteger().intValue());
else
((HostPseudoModel) pObj).beep(0);
}
public static void exportData(Obj pObj, Arg oRetVal, Arg[] pArgs) {
if (pArgs.length == 2)
((HostPseudoModel) pObj).exportData(pArgs[0].getString(), pArgs[1].getAsBool(false).booleanValue());
else if (pArgs.length == 1)
((HostPseudoModel) pObj).exportData(pArgs[0].getString(), true);
else
((HostPseudoModel) pObj).exportData("", true);
}
public static void getAppType(Obj pObj, Arg oRetVal) {
oRetVal.setString(((HostPseudoModel) pObj).getAppType());
}
public static void getCalculationsEnabled(Obj pObj, Arg oRetVal) {
oRetVal.setBool(Boolean.valueOf(((HostPseudoModel) pObj).getCalculationsEnabled()));
}
public static void getCurrentDateTime(Obj pObj, Arg oRetVal, Arg[] pArgs) {
oRetVal.setString(((HostPseudoModel) pObj).getCurrentDateTime());
}
public static void getCurrentPage(Obj pObj, Arg oRetVal) {
oRetVal.setInteger(Integer.valueOf(((HostPseudoModel) pObj).getCurrentPage()));
}
public static void getDocumentInBatch(Obj pObj, Arg oRetVal, Arg pArgs[]) {
oRetVal.setInteger(Integer.valueOf(((HostPseudoModel) pObj).getDocumentInBatch()));
}
public static void getDocumentCountInBatch(Obj pObj, Arg oRetVal, Arg[] pArgs) {
oRetVal.setInteger(Integer.valueOf(((HostPseudoModel) pObj).getDocumentCountInBatch()));
}
public static void getFocus(Obj pObj,Arg oRetVal, Arg[] pArgs) {
oRetVal.setObject(((HostPseudoModel) pObj).getFocus());
}
public static void getLanguage(Obj pObj, Arg oRetVal) {
oRetVal.setString(((HostPseudoModel) pObj).getLanguage());
}
public static void getName(Obj pObj, Arg oRetVal) {
oRetVal.setString(((HostPseudoModel) pObj).getName());
}
public static void getNumPages(Obj pObj, Arg oRetVal) {
oRetVal.setInteger(Integer.valueOf(((HostPseudoModel) pObj).getNumPages()));
}
public static void getPlatform(Obj pObj, Arg oRetVal) {
oRetVal.setString(((HostPseudoModel) pObj).getPlatform());
}
public static void getTitle(Obj pObj, Arg oRetVal) {
oRetVal.setString(((HostPseudoModel) pObj).getTitle());
}
public static void getValidationsEnabled(Obj pObj, Arg oRetVal) {
oRetVal.setBool(Boolean.valueOf(((HostPseudoModel) pObj).getValidationsEnabled()));
}
public static void getVariation(Obj pObj, Arg oRetVal) {
oRetVal.setString(((HostPseudoModel) pObj).getVariation());
}
public static void getVersion(Obj pObj, Arg oRetVal) {
oRetVal.setString(((HostPseudoModel) pObj).getVersion());
}
public static void gotoURL(Obj pObj, Arg oRetVal, Arg[] pArgs) {
if (pArgs.length == 2)
((HostPseudoModel) pObj).gotoURL(pArgs[0].getString(), pArgs[1].getAsBool(false).booleanValue());
else
((HostPseudoModel) pObj).gotoURL(pArgs[0].getString(), false);
}
public static void importData(Obj pObj, Arg oRetVal, Arg[] pArgs) {
if (pArgs.length == 1)
((HostPseudoModel) pObj).importData(pArgs[0].getString());
else
((HostPseudoModel) pObj).importData("");
}
public static boolean importDataPermsCheck(Obj obj, Arg[] args) {
return ((HostPseudoModel) obj).importDataPermsCheck();
}
public static void messageBox(Obj pObj, Arg oRetVal, Arg[] pArgs) {
if (pArgs.length == 4)
oRetVal.setInteger(Integer.valueOf(((HostPseudoModel) pObj).messageBox(pArgs[0].getString(),
pArgs[1].getString(),
pArgs[2].getInteger().intValue(),
pArgs[3].getInteger().intValue())));
else if (pArgs.length == 3)
oRetVal.setInteger(Integer.valueOf(((HostPseudoModel) pObj).messageBox(pArgs[0].getString(),
pArgs[1].getString(),
pArgs[2].getInteger().intValue(),
0)));
else if (pArgs.length == 2)
oRetVal.setInteger(Integer.valueOf(((HostPseudoModel) pObj).messageBox(pArgs[0].getString(),
pArgs[1].getString(),
0,
0)));
else
oRetVal.setInteger(Integer.valueOf(((HostPseudoModel) pObj).messageBox(pArgs[0].getString(),
"",
0,
0)));
}
public static void openList(Obj pObj, Arg oRetVal, Arg[] pArgs) {
if (pArgs[0].getArgType() == Arg.OBJECT) {
Obj xfaobj = pArgs[0].getObject();
if (! (xfaobj instanceof Node))
throw new ExFull(ResId.ArgumentMismatchException);
Node xfanode = (Node) xfaobj;
((HostPseudoModel) pObj).openList(xfanode);
}
else {
String sNode = pArgs[0].getString();
((HostPseudoModel) pObj).openList(sNode);
}
}
public static void pageDown(Obj pObj, Arg oRetVal, Arg[] pArgs) {
((HostPseudoModel) pObj).pageDown();
}
public static void pageUp(Obj pObj,Arg oRetVal, Arg[] pArgs) {
((HostPseudoModel) pObj).pageUp();
}
public static void print(Obj pObj, Arg oRetVal, Arg[] pArgs) {
((HostPseudoModel) pObj).print(pArgs[0].getAsBool(false).booleanValue(),
pArgs[1].getInteger().intValue(),
pArgs[2].getInteger().intValue(),
pArgs[3].getAsBool(false).booleanValue(),
pArgs[4].getAsBool(false).booleanValue(),
pArgs[5].getAsBool(false).booleanValue(),
pArgs[6].getAsBool(false).booleanValue(),
pArgs[7].getAsBool(false).booleanValue());
}
public static void resetData(Obj pObj, Arg oRetVal, Arg[] pArgs) {
List oNodes = new ArrayList();
if (pArgs.length == 1) {
String sNodes = pArgs[0].getString();
StringTokenizer sToker = new StringTokenizer(sNodes, STRS.COMMA);
//
// get the fields to reset
//
while (sToker.hasMoreTokens())
oNodes.add(sToker.nextToken());
}
((HostPseudoModel) pObj).resetData(oNodes);
}
public static boolean resetDataPermsCheck(Obj obj, Arg[] args) {
if (obj instanceof PseudoModel) {
List oNodes = new ArrayList();
if (args.length == 1) {
String sNodes = args[0].getString();
StringTokenizer sToker = new StringTokenizer(sNodes, STRS.COMMA);
//
// get the fields to reset
//
while (sToker.hasMoreTokens())
oNodes.add(sToker.nextToken());
}
return ((HostPseudoModel) obj).resetDataPermsCheck(oNodes);
}
return true;
}
public static void response(Obj pObj, Arg oRetVal, Arg[] pArgs) {
String sRetVal;
if (pArgs.length == 4)
sRetVal = ((HostPseudoModel) pObj).response(pArgs[0].getString(), pArgs[1].getString(), pArgs[2].getString(), pArgs[3].getAsBool(false).booleanValue());
else if (pArgs.length == 3)
sRetVal = ((HostPseudoModel) pObj).response(pArgs[0].getString(), pArgs[1].getString(), pArgs[2].getString(), false);
else if (pArgs.length == 2)
sRetVal = ((HostPseudoModel) pObj).response(pArgs[0].getString(), pArgs[1].getString(), "", false);
else
sRetVal = ((HostPseudoModel) pObj).response(pArgs[0].getString(), "", "", false);
// Watson 1660669: return value should be NULL if user hits cancel.
if (sRetVal == null)
oRetVal.setNull();
else
oRetVal.setString(sRetVal);
}
public static void setCurrentPage(Obj pObj, Arg propertyValue) {
((HostPseudoModel) pObj).setCurrentPage(propertyValue.getInteger().intValue());
}
public static void setFocus(Obj pObj, Arg oRetVal, Arg[] pArgs) {
boolean isClearFocus = false;
if (pArgs.length >= 1) {
if (pArgs[0].getArgType() == Arg.OBJECT) {
Obj xfaobj = pArgs[0].getObject();
if (! (xfaobj instanceof Node))
throw new ExFull(ResId.ArgumentMismatchException);
Node xfanode = (Node) xfaobj;
((HostPseudoModel) pObj).setFocus(xfanode);
}
else if (pArgs[0].getArgType() == Arg.EMPTY) {
isClearFocus = true;
}
else if (pArgs[0].getArgType() == Arg.NULL) {
isClearFocus = true;
}
else {
String sNode = pArgs[0].getString();
((HostPseudoModel) pObj).setFocus(sNode);
}
}
else
isClearFocus = true;
if (isClearFocus) {
Node xfanode = null; // A call with a null node implies a clear focus
((HostPseudoModel) pObj).setFocus(xfanode);
}
}
public static void setTitle(Obj pObj, Arg propertyValue) {
((HostPseudoModel) pObj).setTitle(propertyValue.getString());
}
public static void setValidationsEnabled(Obj pObj, Arg propertyValue) {
((HostPseudoModel) pObj).setValidationsEnabled(propertyValue.getAsBool(false).booleanValue());
}
public static void setCalculationsEnabled(Obj pObj, Arg propertyValue) {
((HostPseudoModel) pObj).setCalculationsEnabled(propertyValue.getAsBool(false).booleanValue());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy