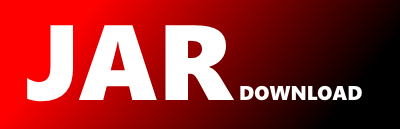
com.adobe.xfa.ScriptHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa;
import java.util.List;
import com.adobe.xfa.scripthandler.formcalc.FormCalcScriptHandler;
import com.adobe.xfa.scripthandler.rhino.RhinoScriptHandler;
import com.adobe.xfa.ut.MsgFormatPos;
import com.adobe.xfa.ut.ResId;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.MsgFormat;
/**
*
* A base class to handle scripting engines.
* A script handler is used by an XFA application model to enable scripting support.
* A script handler provides support for one particular language.
*/
public abstract class ScriptHandler {
/**
* The unspecified script reason.
*/
public static final int UNSPECIFIED = 0;
/**
* The calculate script reason.
*/
public static final int CALCULATE = 1;
/**
* The validate script reason.
*/
public static final int VALIDATE = 2;
/**
* An activity initialize script reason.
*/
public static final int ACTIVITY_INITIALIZE = 3;
/**
* An activity pre-save script reason.
*/
public static final int ACTIVITY_PRESAVE = 4;
/**
* The activity post-save reason.
*/
public static final int ACTIVITY_POSTSAVE = 5;
/**
* The activity pre-print reason.
*/
public static final int ACTIVITY_PREPRINT = 6;
/**
* The activity post-print reason.
*/
public static final int ACTIVITY_POSTPRINT = 7;
/**
* The activity ready reason.
*/
public static final int ACTIVITY_READY = 8;
/**
* The activity doc ready reason.
*/
public static final int ACTIVITY_DOCREADY = 9;
/**
* The activity do close reason.
*/
public static final int ACTIVITY_DOCCLOSE = 10;
/**
* The activity pre-submit reason.
*/
public static final int ACTIVITY_PRESUBMIT = 11;
/**
* The activity pre-execute reason.
*/
public static final int ACTIVITY_PREEXECUTE = 12;
/**
* The activity post-execute reason.
*/
public static final int ACTIVITY_POSTEXECUTE = 13;
/**
* The activity pre-open reason.
*/
public static final int ACTIVITY_PREOPEN = 14;
/**
* The activity index change reason.
*/
public static final int ACTIVITY_INDEXCHANGE = 15;
/**
* The predicate (when executing a predicate in a SOM expression) reason.
*/
public static final int PREDICATE = 16;
/**
* The activity pre-sign reason.
*/
public static final int ACTIVITY_PRESIGN = 17;
/**
* The activity post-sign reason.
*/
public static final int ACTIVITY_POSTSIGN = 18;
/**
* The activity post-submit reason.
*/
public static final int ACTIVITY_POSTSUBMIT = 19;
/**
* The activity post-open reason.
*/
public static final int ACTIVITY_POSTOPEN = 20;
/**
* The activity validation state reason.
*/
public static final int ACTIVITY_VALIDATIONSTATE = 21;
/**
* @exclude from published api.
*/
public static final int ObjectInteraction_Start = 22; // (not used) All events below are user interaction events with objects
/**
* The activity enter reason.
*/
public static final int ACTIVITY_ENTER = 23;
/**
* The activity exit reason.
*/
public static final int ACTIVITY_EXIT = 24;
/**
* The activity mouse enter reason.
*/
public static final int ACTIVITY_MOUSEENTER = 25;
/**
* The activity mouse exit reason.
*/
public static final int ACTIVITY_MOUSEEXIT = 26;
/**
* The activity change reason.
*/
public static final int ACTIVITY_CHANGE = 27;
/**
* The activity click reason.
*/
public static final int ACTIVITY_CLICK = 28;
/**
* The activity full reason.
*/
public static final int ACTIVITY_FULL = 29;
/**
* The activity mouse up reason.
*/
public static final int ACTIVITY_MOUSEUP = 30;
/**
* The activity mouse down reason.
*/
public static final int ACTIVITY_MOUSEDOWN = 31;
/**
* @exclude from published api.
*/
public static final int ObjectInteraction_End = 32; // (not used) All events above are user interaction events with objects
/**
* @exclude from published api.
*/
public static final int executeReason_LAST = 33; // only used to mark the end.
/**
* @exclude from published api.
*/
public static final int ERR_OK = 0; // No error, success.
// Javaport: ExtendScript isn't used in Java, and Rhino doesn't have such things.
// // ExtendScript errors
// public static final int ERR_General = 1; // %1 (Error)
// public static final int ERR_Undefined = 2; // %1 is undefined (ReferenceError)
// public static final int ERR_NoLvalue = 3; // Cannot assign value (ReferenceError)
// public static final int ERR_OpenString = 4; // Unterminated string constant (SyntaxError)
// public static final int ERR_OpenComment = 5; // Unterminated comment (SyntaxError)
// public static final int ERR_BadDigit = 6; // Bad digit in number (SyntaxError)
// public static final int ERR_Unsupported = 7; // Language feature %1 is not supported (SyntaxError)
/**
* @exclude from published api.
*/
public static final int ERR_Syntax = 8; // Syntax error (SyntaxError)
// public static final int ERR_Keyword = 9; // Illegal use of reserved word '%1' (SyntaxError)
/**
* @exclude from published api.
*/
public static final int ERR_BadBreakContinue = 10; // Break or Continue outside a loop (SyntaxError)
// public static final int ERR_BadLabel = 11; // Label not found (SyntaxError)
// public static final int ERR_ExpressionNotConst = 12; // Expression is not constant (SyntaxError)
// public static final int ERR_ClosedBlock = 13; // Too many closing braces (SyntaxError)
// public static final int ERR_OpenBlock = 14; // No matching closing brace found (SyntaxError)
// public static final int ERR_NoCatch = 15; // try without catch/finally (SyntaxError)
// public static final int ERR_NoTry = 16; // catch/finally without try (SyntaxError)
// public static final int ERR_VarExpected = 17; // Variable expected (TypeError)
// public static final int ERR_ScalarExpected = 18; // Variable or value expected, but found %1 (TypeError)
// public static final int ERR_BadArgument = 19; // Bad argument %1 (TypeError)
// public static final int ERR_BadArgumentList = 20; // Bad argument list (TypeError)
// public static final int ERR_ObjectExpected = 21; // %1 is not an object (TypeError)
// public static final int ERR_NoCtor = 22; // %1 does not have a constructor (ReferenceError)
// public static final int ERR_NoValue = 23; // %1 does not have a value (ReferenceError)
// public static final int ERR_NoFunction = 24; // %1 is not a function (ReferenceError)
// public static final int ERR_Expected = 25; // Expected: %1 (SyntaxError)
// public static final int ERR_WrongClass = 26; // %1 cannot work with this class (Error)
// public static final int ERR_BadReturn = 30; // Illegal 'return' outside of a function body (SyntaxError)
// public static final int ERR_CharConversion = 37; // Conversion error (SyntaxError)
// public static final int ERR_CharPartial = 38; // Partial multibyte character found (SyntaxError)
// public static final int ERR_DuplicateDefault = 39; // More than one switch default
// public static final int ERR_Redeclared = 40; // %1 redeclared (TypeError)
// public static final int ERR_Range = 41; // %1 is out of range (RangeError)
// public static final int ERR_CatchAfterCatch = 42; // Catch after unconditional catch (SyntaxError)
/*
* Following are FC-specific errors
*/
/**
* @exclude from published api.
*/
public static final int ERR_FuncBuiltIn = 100; // function '%1' on line %2, column %3 is built in.
/**
* @exclude from published api.
*/
public static final int ERR_FuncUnknown = 101; // function '%1' on line %2, column %3 is unknown.
/**
* @exclude from published api.
*/
public static class ScriptException extends ExFull {
static final long serialVersionUID = 132047461644242605L;
public ScriptException(MsgFormat oFormat, int nLineNumber, int nErrorCode) {
super(oFormat);
mnLineNumber = nLineNumber;
mnErrorCode = nErrorCode;
}
public int getLineNumber() {
return mnLineNumber;
}
public int getErrorCode() {
return mnErrorCode;
}
private final int mnLineNumber;
private final int mnErrorCode;
}
/**
* Instantiates a script handler.
*/
public ScriptHandler() {
this(null);
}
/**
* Instantiates a script handler using the given debugger.
* @param oDebugger a script debugger.
*
* @exclude from published api.
*/
public ScriptHandler(ScriptDebugger oDebugger) {
moDebugger = oDebugger;
if (moDebugger != null)
moDebugger.private_attach(this);
}
/**
* Create a new ScriptHandler that can handle a given contentType.
*
* @param contentType the contentType of the script.
* @param appModel the AppModel that contains the context for the script.
* @param debugger the ScriptDebugger for debugging the script, or null if debugging is not required.
* @return a new ScriptHandler.
* @throws ExFull if the contentType is not recognized.
* @deprecated This won't work in client environments.
*
* @exclude from published api.
*/
public static ScriptHandler createScriptHandler(String contentType, AppModel appModel, ScriptDebugger debugger) {
/*
* Handle the same content types as Element.evaluate
*/
if (contentType.length() == 0)
contentType = "formcalc";
else if (contentType.startsWith("application/x-"))
contentType = contentType.substring("application/x-".length());
if (contentType.equals("formcalc"))
return new FormCalcScriptHandler(appModel, debugger);
else if (contentType.equals("javascript"))
return new RhinoScriptHandler(appModel, debugger);
else {
MsgFormatPos msg = new MsgFormatPos(ResId.UnknownScriptLanguageException);
msg.format(contentType); // script type eg. formcalc
msg.format(""); // context
throw new ExFull(msg);
}
}
/**
* Set the debugger for this script handler. Can also be specified in the
* constructor.
*
* @exclude from published api.
*/
public void setDebugger(ScriptDebugger oDebugger) {
if (moDebugger != null) {
moDebugger.private_detach(this);
assert(moDebugger == null); // private_detach should clear this
}
moDebugger = oDebugger;
if (moDebugger != null)
moDebugger.private_attach(this);
}
/**
* Executes or checks the syntax of the given script.
*
* @param script
* the script to execute.
* @param oReturnValue
* the return value of the script.
* @param eReason
* the reason for the execution of the script.
* @param bSyntaxCheckOnly
* the flag to only check syntax when set.
* @throws ExFull
* whenever errors are reported.
* @exclude from published api.
*/
abstract public void executeOrSyntaxCheck(String script, Arg oReturnValue, int eReason, boolean bSyntaxCheckOnly);
/**
* Executes the given script.
*
* @param script
* the script to execute.
* @param locale
* the operating locale.
* @param returnValue
* the return value of the script.
* @param eReason
* the reason for the execution of the script.
* @throws ExFull
* whenever errors are reported.
*/
public void execute(String script, String locale, Arg returnValue, int eReason /* = UNSPECIFIED */) {
executeOrSyntaxCheck(script, returnValue, eReason, false);
}
/**
* Checks the given script for syntax errors. Errors are reported in the
* form of a scriptException exception (scriptException derives from
* ExFull).
*
* @param script
* the script to execute.
* @throws ScriptException
* upon syntax error. ScriptException derives from ExFull.
* @exclude from published api.
*/
public void syntaxCheck(String script) {
executeOrSyntaxCheck(script, new Arg(), UNSPECIFIED, true);
}
/**
* Deletes any accumulated script execution contexts.
*
* @exclude from published api.
*/
protected void clearExecutionContexts() {
};
/**
* Gets the name of the scripting language supported by this script handler.
*
* @return the name of the scripting language.
*/
abstract public String languageName();
/**
* Sets the value of the given option. If the option is unknown, the
* call is ignored.
*
* @param sOptionName
* the name of the option to set.
* @param sOptionValue
* the value to set.
* @exclude from published api.
*/
public void setOption(String sOptionName, String sOptionValue) {
}
/**
* Gets the value of the given option. If the option is unknown, the
* empty string is returned.
*
* @param sOptionName
* the name of the option to get.
* @return
* the value of the specified option as a string.
* @exclude from published api.
*/
public String getOption(String sOptionName) {
return "";
}
/**
* Determines the severity of an error is returned from execute().
*
* @exclude from published api.
*/
public boolean wasFatalError() {
return true;
}
/**
* Clones this script handler.
*
* @return a copy of this script handler.
*/
public abstract ScriptHandler clone();
// Javaport: not yet needed.
// /**
// * Compile a given script object.
// *
// * @param sScript
// * the script to object compile.
// * @return the unique identifier of the object.
// * @exception JF_SH_COMPILE_ERROR
// * if there is an error in the script
// */
// abstract long compileObject(String sScript);
/**
* Converts a string into an executeReason.
*
* @return the executeReason representing the string (UNSPECIFIED if not recognized).
*
* @exclude from public api.
*/
public static int stringToExecuteReason(String sReason) {
// special (non-enum) cases:
if (sReason.equals(XFA.CALCULATE))
return CALCULATE;
if (sReason.equals(XFA.VALIDATE))
return VALIDATE;
if (sReason.equals("predicate"))
return PREDICATE;
EnumAttr oEnum = EnumAttr.getEnum(EnumType.ACTIVITY_TYPE, sReason.intern());
switch (oEnum.getInt()) {
case EnumAttr.ACTIVITY_INITIALIZE:
return ACTIVITY_INITIALIZE;
case EnumAttr.ACTIVITY_ENTER:
return ACTIVITY_ENTER;
case EnumAttr.ACTIVITY_EXIT:
return ACTIVITY_EXIT;
case EnumAttr.ACTIVITY_MOUSEENTER:
return ACTIVITY_MOUSEENTER;
case EnumAttr.ACTIVITY_MOUSEEXIT:
return ACTIVITY_MOUSEEXIT;
case EnumAttr.ACTIVITY_CHANGE:
return ACTIVITY_CHANGE;
case EnumAttr.ACTIVITY_CLICK:
return ACTIVITY_CLICK;
case EnumAttr.ACTIVITY_PRESAVE:
return ACTIVITY_PRESAVE;
case EnumAttr.ACTIVITY_POSTSAVE:
return ACTIVITY_POSTSAVE;
case EnumAttr.ACTIVITY_PREPRINT:
return ACTIVITY_PREPRINT;
case EnumAttr.ACTIVITY_POSTPRINT:
return ACTIVITY_POSTPRINT;
case EnumAttr.ACTIVITY_READY:
return ACTIVITY_READY;
case EnumAttr.ACTIVITY_FULL:
return ACTIVITY_FULL;
case EnumAttr.ACTIVITY_MOUSEUP:
return ACTIVITY_MOUSEUP;
case EnumAttr.ACTIVITY_MOUSEDOWN:
return ACTIVITY_MOUSEDOWN;
case EnumAttr.ACTIVITY_DOCREADY:
return ACTIVITY_DOCREADY;
case EnumAttr.ACTIVITY_DOCCLOSE:
return ACTIVITY_DOCCLOSE;
case EnumAttr.ACTIVITY_PRESUBMIT:
return ACTIVITY_PRESUBMIT;
case EnumAttr.ACTIVITY_PREEXECUTE:
return ACTIVITY_PREEXECUTE;
case EnumAttr.ACTIVITY_POSTEXECUTE:
return ACTIVITY_POSTEXECUTE;
case EnumAttr.ACTIVITY_PREOPEN:
return ACTIVITY_PREOPEN;
case EnumAttr.ACTIVITY_INDEXCHANGE:
return ACTIVITY_INDEXCHANGE;
case EnumAttr.ACTIVITY_PRESIGN:
return ACTIVITY_PRESIGN;
case EnumAttr.ACTIVITY_POSTSIGN:
return ACTIVITY_POSTSIGN;
case EnumAttr.ACTIVITY_POSTSUBMIT:
return ACTIVITY_POSTSUBMIT;
case EnumAttr.ACTIVITY_POSTOPEN:
return ACTIVITY_POSTOPEN;
case EnumAttr.ACTIVITY_VALIDATIONSTATE:
return ACTIVITY_VALIDATIONSTATE;
default:
return UNSPECIFIED;
}
}
/**
* Converts an executeReason into a string.
*
* @return the string representing the executeReason ("other" if not recognized).
*
* @exclude from published api.
*/
public static String executeReasonToString(int eReason) {
// special (non-enum) cases:
if (eReason == ScriptHandler.CALCULATE)
return XFA.CALCULATE;
if (eReason == ScriptHandler.VALIDATE)
return XFA.VALIDATE;
if (eReason == ScriptHandler.PREDICATE)
return "predicate";
int oEnum = executeReasonToXFAEnum(eReason);
if (oEnum == UNSPECIFIED)
return "other"; // yeah, it's English!
return EnumAttr.getString(oEnum);
}
/**
* Converts an executeReason into an XFAEnum.
*
* @return the XFAEnum corresponding to the executeReason (NULL if not
* recognized). Note in particular that UNSPECIFIED, CALCULATE, and
* VALIDATE have no XFAEnum equivalent.
*
* @exclude from published api.
*/
public static int executeReasonToXFAEnum(int eReason) {
switch (eReason) {
case ACTIVITY_INITIALIZE:
return EnumAttr.ACTIVITY_INITIALIZE;
case ACTIVITY_ENTER:
return EnumAttr.ACTIVITY_ENTER;
case ACTIVITY_EXIT:
return EnumAttr.ACTIVITY_EXIT;
case ACTIVITY_MOUSEENTER:
return EnumAttr.ACTIVITY_MOUSEENTER;
case ACTIVITY_MOUSEEXIT:
return EnumAttr.ACTIVITY_MOUSEEXIT;
case ACTIVITY_CHANGE:
return EnumAttr.ACTIVITY_CHANGE;
case ACTIVITY_CLICK:
return EnumAttr.ACTIVITY_CLICK;
case ACTIVITY_PRESAVE:
return EnumAttr.ACTIVITY_PRESAVE;
case ACTIVITY_POSTSAVE:
return EnumAttr.ACTIVITY_POSTSAVE;
case ACTIVITY_PREPRINT:
return EnumAttr.ACTIVITY_PREPRINT;
case ACTIVITY_POSTPRINT:
return EnumAttr.ACTIVITY_POSTPRINT;
case ACTIVITY_READY:
return EnumAttr.ACTIVITY_READY;
case ACTIVITY_MOUSEUP:
return EnumAttr.ACTIVITY_MOUSEUP;
case ACTIVITY_MOUSEDOWN:
return EnumAttr.ACTIVITY_MOUSEDOWN;
case ACTIVITY_DOCREADY:
return EnumAttr.ACTIVITY_DOCREADY;
case ACTIVITY_DOCCLOSE:
return EnumAttr.ACTIVITY_DOCCLOSE;
case ACTIVITY_FULL:
return EnumAttr.ACTIVITY_FULL;
case ACTIVITY_PRESUBMIT:
return EnumAttr.ACTIVITY_PRESUBMIT;
case ACTIVITY_PREEXECUTE:
return EnumAttr.ACTIVITY_PREEXECUTE;
case ACTIVITY_POSTEXECUTE:
return EnumAttr.ACTIVITY_POSTEXECUTE;
case ACTIVITY_PREOPEN:
return EnumAttr.ACTIVITY_PREOPEN;
case ACTIVITY_INDEXCHANGE:
return EnumAttr.ACTIVITY_INDEXCHANGE;
case ACTIVITY_PRESIGN:
return EnumAttr.ACTIVITY_PRESIGN;
case ACTIVITY_POSTSIGN:
return EnumAttr.ACTIVITY_POSTSIGN;
case ACTIVITY_POSTSUBMIT:
return EnumAttr.ACTIVITY_POSTSUBMIT;
case ACTIVITY_POSTOPEN:
return EnumAttr.ACTIVITY_POSTOPEN;
case ACTIVITY_VALIDATIONSTATE:
return EnumAttr.ACTIVITY_VALIDATIONSTATE;
default:
return UNSPECIFIED;
}
}
// Debugging routines
/**
* Get the debugger for this script handler.
* @exclude from published api.
*/
public ScriptDebugger getDebugger() {
return moDebugger;
}
/**
* enum debugCommandCode
*/
/**
* @exclude from published api.
*/
public static final int STEP_OVER = 0;
/**
* @exclude from published api.
*/
public static final int STEP_INTO = 1;
/**
* @exclude from published api.
*/
public static final int STEP_OUT = 2;
/**
* @exclude from published api.
*/
public static final int BREAK = 3;
/**
* @exclude from published api.
*/
public static final int HALT = 4;
/**
* Set an internal flag in the script handler indicating that, when control
* is returned to the script handler, it should perform a step-over,
* step-into or step-out function (calling the stopped callback on the
* associated debugger).
*
* @exclude from published api.
*/
public boolean debugCommand(int eCmd) {
return false;
}
/**
* enum debugBreakPointCode
*/
/**
* @exclude from published api.
*/
public static final int BP_SET = 0;
/**
* @exclude from published api.
*/
public static final int BP_CLEAR = 1;
/**
* Set or clear a break-point.
*
* @exclude from published api.
*/
public boolean debugBreakPoint(int nScriptID, int nLine, int eSetType) {
return false;
}
/**
* Get the current stack frame.
*
* @exclude from published api.
*/
public boolean debugGetStack(List oStack) {
return false;
}
/**
* Retrieve parallel lists of variable names and their values (lists have
* the same size). Note that this will only give meaningful results when
* stopped at a break-point.
*
* @exclude from published api.
*/
public boolean debugGetVariables(List oVarNames, List oVarValues) {
return false;
}
/*
* enum debugFeaturesCode
* @exclude from published api.
*/
/**
* @exclude from published api.
*/
public static final int FEATURE_NONE = 0;
/**
* @exclude from published api.
*/
public static final int FEATURE_IS_REMOTE = 1;
/**
* @exclude from published api.
*/
public static final int FEATURE_CAN_SET_BREAKPOINT = 2;
/**
* @exclude from published api.
*/
public static final int FEATURE_CAN_STEP_OVER = 4;
/**
* @exclude from published api.
*/
public static final int FEATURE_CAN_STEP_INTO = 8;
/**
* @exclude from published api.
*/
public static final int FEATURE_CAN_STEP_OUT = 16;
/**
* @exclude from published api.
*/
public static final int FEATURE_CAN_BREAK = 32;
/**
* @exclude from published api.
*/
public static final int FEATURE_CAN_HALT = 64;
/**
* Retrieves the capabilities of this script handler.
*
* @exclude from published api.
*/
protected int debugFeatures() {
return FEATURE_NONE;
}
/**
* Removes any script references to the node and the descendants
*
* @exclude from public api.
*/
public void removeReference(Node oNode) {
// JavaPort: implement this?
}
ScriptDebugger moDebugger;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy