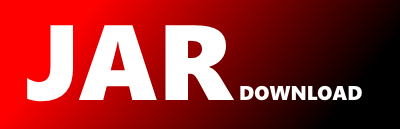
com.adobe.xfa.content.IntegerValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.content;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Element;
import com.adobe.xfa.LogMessage;
import com.adobe.xfa.Node;
import com.adobe.xfa.ScriptTable;
import com.adobe.xfa.XFA;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.MsgFormatPos;
import com.adobe.xfa.ut.ResId;
import com.adobe.xfa.ut.StringUtils;
/**
* An element that creates a unit of data content representing an
* integer value.
*
* integer-data is PCDATA that obeys the following rules:
* 1. an optional sign
* 2. no fractional digits
* 3. no exponent
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public final class IntegerValue extends Content {
public IntegerValue(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.INTEGER, XFA.INTEGER, null,
XFA.INTEGERTAG, XFA.INTEGER);
}
public boolean equals(Object object) {
if (this == object)
return true;
return super.equals(object) &&
getValue() == ((IntegerValue) object).getValue();
}
public int hashCode() {
return super.hashCode() ^ getValue();
}
public ScriptTable getScriptTable() {
return IntegerScript.getScriptTable();
}
/**
* Retrieve the value as an integer.
*
* @return the value as integer.
*/
public int getValue() {
final String sValue = getStrValue();
if (StringUtils.isEmpty(sValue))
return 0;
try {
return Integer.parseInt(sValue);
}
catch(NumberFormatException e) {
// Watson bug 1704232 since we can't update the value when ::setStrValue is called because that
// could accidentally change the value stored in the XFA Data Dom, we have to ensure we don't throw
// when the value of this object is queried.
// log a warning
final MsgFormatPos oMessage = new MsgFormatPos(ResId.FoundBadElementValueException);
oMessage.format(sValue);
oMessage.format(getSOMExpression());
oMessage.format("0");
getModel().addErrorList(new ExFull(oMessage), LogMessage.MSG_WARNING, this);
// like XFADoubleImpl and XFAFloatImpl return the default value of 0.
return 0;
}
}
/**
* Update the integer value
*
* @param newValue
* new value as integer
*/
public void setValue(int newValue) {
setStrValue(Integer.toString(newValue), true, false);
}
/**
* Update the integer value
*
* @param sValue
* new value as string. Use this method to set the value to null.
*/
public void setValue(String sValue, boolean bFromData /* = false */) {
if (StringUtils.isEmpty(sValue)) {
setStrValue("", true, false);
}
else {
boolean bTypeMismatch = false;
int nVal = 0;
try {
nVal = Integer.parseInt(sValue);
}
catch(NumberFormatException e) {
setStrValue("", true, false);
bTypeMismatch = true;
}
if (bTypeMismatch) {
if (!getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V30_SCRIPTING)) {
MsgFormatPos oMessage = new MsgFormatPos(ResId.TypeMismatch);
oMessage.format(getSOMExpression());
oMessage.format(sValue);
getModel().addErrorList(new ExFull(oMessage), LogMessage.MSG_WARNING, this);
}
if (getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V29_SCRIPTING)) {
setValue(nVal); // legacy behavior is to set to zero
}
else {
if (bFromData) // data is king, even if it's a type mismatch
setStrValue(sValue, true, false);
// else
// ; // otherwise leave existing value unchanged
}
return;
}
setValue(nVal);
}
}
public String toString() {
// Javaport: Returning "0" for a null value is a bit strange, but it is what C++ does
if (getIsNull())
return "0";
return getStrValue();
}
/**
* Returns true
if the current value does not legally parse
* into an integer.
* @exclude from published api.
*/
public boolean valueHasTypeMismatch() {
String sValue = getStrValue();
if (StringUtils.isEmpty(sValue))
return false;
try {
Integer.parseInt(sValue);
return false;
}
catch(NumberFormatException ex) {
return true;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy