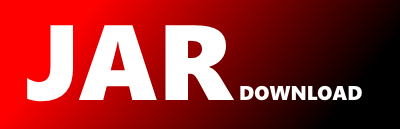
com.adobe.xfa.formcalc.ScriptHost Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.formcalc;
import com.adobe.xfa.Obj;
/**
* Class ScriptHost defines the interface by which the
* {@link CalcParser} (FormCalc scripting engine)
* can access a script host whenever it
* needs to resolve scripting object model (SOM) references.
*
* FormCalc applications need to inform the FormCalc script engine
* that they are capable of supporting the ScriptHost
* interface by invoking the
* {@link CalcParser#setScriptHost(ScriptHost oScriptHost)}
* method.
*
* @author Paul Imeson
*
* @exclude from published api.
*/
public interface ScriptHost {
/**
* Abstract method to get the handle of a SOM object.
*
*
A SOM reference provides access to objects, object properties
* and methods, as well as access to the values of objects,
* properties and methods. A SOM reference may be qualified,
* as in, for example, Field2.Color[2].Value, Field3[-1] -- some
* of which may be relative to the current object.
* A SOM reference may also contain any number of object literals
* of the form #N where N is a non-negative number
* corresponding to the 0-based index of an array of ObjImpl *.
* As an example of SOM references with object literals consider:
* #0.Parent, and #0.SetColor(#1.Border[1].Value)
*
*
The return object is an object handle -- an ObjImpl *.
* If the given SOM reference refers to several SOM objects, this
* method should throw an exception.
*
* @param sItem a given SOM reference.
*
* @param oObj an array of objects. If
* non-null, this is array whose size corresponds
* to the maximal object literal occurence in the given SOM reference.
*
* @return a returned object upon success.
*
* @throws {@link CalcException}
* upon error.
*/
Obj getItem(String sItem, Obj[] oObj);
/**
* Virtual method to get the value(s) of a SOM reference.
*
*
A SOM reference provides access to objects, object properties
* and methods, as well as access to the values of objects,
* properties and methods. A SOM reference may be qualified,
* as in, for example, Field2.Color[2].Value, Field3[-1] -- some
* of which may be relative to the current object.
* A SOM reference may also contain any number of object literals
* of the form #N where N is a non-negative number
* corresponding to the 0-based index of an array of Obj.
* As an example of SOM references with object literals consider:
* #0.Parent, and #0.SetColor(#1.Border[1].Value)
*
*
The return values are objects of type
* {@link CalcSymbol}
* which are no more than typed-values.
References to:
*
* - null-valued objects should be returned as
* {@link CalcSymbol}s of type TypeNull.
*
- string-valued objects should be returned as
* {@link CalcSymbol}s of type TypeString.
*
- numeric-valued objects should be preferably returned
* as string-valued
* {@link CalcSymbol}s of type TypeString
* to avoid loss of precision, although it is possible for them to
* be returned as numeric-valued
* {@link CalcSymbol}s of type TypeDouble.
*
- errors should be returned as error-valued
* {@link CalcSymbol}s of type TypeError.
*
*
*
* To illustrate all the above, here's a degenerate implementation that,
* irrespective of the SOM reference given, always returns a single value
* of type error, accompanied with an "unknown accessor" message text.
*
*
* import com.adobe.xfa.formcalc.ScriptHost;
* import com.adobe.xfa.formcalc.CalcSymbol;
* import com.adobe.xfa.Obj;
* import com.adobe.xfa.ut.MsgFormat;
* import com.adobe.xfa.ut.ResId;
*
* class FormCalcUser implements ScriptHost {
* CalcSymbol[] getItemValue(String sItem, Obj[] oObj) {
* MsgFormat sFmt = new MsgFormat(ResId.FC_ERR_ACCESSOR);
* sFmt.format(sItem);
* CalcSymbol[] oRetValues = new CalcSymbol[1];
* oRetValues[0] = new CalcSymbol(sFmt, true);
* return oRetValues;
* }
*
* ...
* };
*
*
* @param sItem a SOM reference.
*
* @param oObj an array of objects. If
* non-null, this is an array whose size corresponds
* to the maximal object literal occurence in the given SOM reference.
*
* @return the resulting array of values upon success.
*
* @throws {@link CalcException}
* upon error or catastrophic failure. As noted above, errors can
* returned as
* {@link CalcSymbol} of type TypeError.
*/
CalcSymbol[] getItemValue(String sItem, Obj[] oObj);
/**
* Virtual method to set the value of an SOM reference.
*
*
The given value is an object of type
* {@link CalcSymbol}
* which again, is no more than a typed-value.
* Only CalcSymbols of one of these types will ever be involved:
*
* - null-valued objects of type CalcTypeNull,
*
- string-valued objects of type CalcTypeString, and possibly,
*
- string-valued objects of type CalcTypeVariable.
*
* For the latter two types, use the
* {@link CalcSymbol#getStringValue()} method to get the CalcSymbols's
* value.
*
* @param oObj an array of objects. If
* non-null, this is an array whose size corresponds
* to the maximal object literal occurence in the given SOM reference.
*
* @param oValue the given object value.
*
* @return 0 upon success.
*
* @throws {@link CalcException}
* upon error or catatrophic failure.
*/
int putItem(Obj[] oObj, CalcSymbol oValue);
/**
* Virtual method to set the value of a SOM reference.
*
* A SOM reference provides access to objects, object properties
* and methods, as well as access to the values of objects,
* properties and methods. A SOM reference may be qualified,
* as in, for example, Field2.Color[2].Value, Field3[-1] -- some
* of which may be relative to the current object.
* A SOM reference may also contain any number of object literals
* of the form #N where N is a non-negative number
* corresponding to the 0-based index of an array of ObjImpl *.
* As an example of SOM references with object literals consider:
* #0.Parent, and #0.SetColor(#1.Border[1].Value)
*
*
The given SOM reference may refer to more than one scripting
* object, in which case this methods sets the value of several
* SOM objects.
*
*
The given value is an object of type
* {@link CalcSymbol}
* which again, is no more than a typed-value.
* Only CalcSymbols of one of these types will ever be involved:
*
* - null-valued objects of type CalcTypeNull,
*
- string-valued objects of type CalcTypeString, and possibly,
*
- string-valued objects of type CalcTypeVariable.
*
* For the latter two types, use the
* {@link CalcSymbol#getStringValue()} method to get the CalcSymbols's
* value.
*
* To illustrate all the above, here's a degenerate implementation that,
* stores the given object into a hash table indexed by the given SOM
* reference.
*
* import com.adobe.xfa.formcalc.ScriptHost;
* import com.adobe.xfa.formcalc.CalcSymbol;
* import com.adobe.xfa.ut.Obj;
* import java.util.HashMap;
*
* class FormCalcUser implements ScriptHost {
* HashMap moMap;
*
* int PutItemValue(String sItem, Obj[] oObj, CalcSymbol oValue) {
* moMap.remove(sItem);
* moMap.put(sItem, oValue);
* return 0;
* }
*
* ...
* };
*
*
* @param sItem the given SOM reference.
*
* @param oObj an array of objects. If
* non-null, this is an array whose size corresponds
* to the maximal object literal occurence in the given SOM reference.
*
* @param oValue the given object value.
*
* @return 0 upon success.
*
* @throws {@link CalcException}
* upon error or catatrophic failure.
*/
int putItemValue(String sItem, Obj[] oObj, CalcSymbol oValue);
/**
* Method that is called periodically during script execution.
* The method returns true if user has pressed Esc Key during the execution of FormCalc.
*/
boolean cancelActionOccured();
}