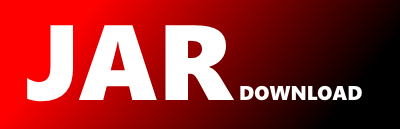
com.adobe.xfa.wsdl.WSDLBindingOperation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2008 Adobe Systems Incorporated
* All Rights Reserved
*
* Notice: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.wsdl;
import com.adobe.xfa.Element;
import com.adobe.xfa.ut.StringUtils;
/**
* @exclude from published api.
*/
public class WSDLBindingOperation extends WSDLNode {
/**
* @exclude from published api.
*/
public static final class SoapStyle {
SoapStyle (int outStyle, int outInputUse, int outOutputUse) {
mOutStyle = outStyle;
mOutInputUse = outInputUse;
mOutOutputUse = outOutputUse;
}
public final int mOutStyle;
public final int mOutInputUse;
public final int mOutOutputUse;
};
public static final int SOAP_STYLE_RPC = 0;
public static final int SOAP_STYLE_DOC = 1;
public static final int SOAP_USE_ENCODED = 0;
public static final int SOAP_USE_LITERAL = 1;
private WSDLOperation mpOperation; // the operation this node binds - keep it as an impl otherwise we run into a nasty memory leak
private WSDLExten moSoapOperation; // the extensibility element soap:operation - child of this node.
private WSDLExten moSoapBinding; // the extensibility element soap:binding - sibling of this node. (child of this node's parent)
private WSDLExten moInputSoapBody; // the extensibility element soap:body - child of this node's input child
private WSDLExten moOutputSoapBody; // the extensibility element soap:body - child of this node's output child
public WSDLBindingOperation (WSDLDocument poDocument, Element oSrc) {
super (poDocument, oSrc, WSDLNode.WSDL_BINDING_OPERATION);
}
/*
* Clone a WSDLBindingOperation.
* @param bDeep - if true then clone recursively.
*/
// Note: cloneNode() commented out. In C++, it is not used by XFA,
// Designer or Acrobat. There is currently no requirement for it in
// Java. The ported code below is untested. This code could be restored
// if a requirement arose.
// public WSDLBindingOperation cloneNode (boolean bDeep) {
// WSDLBindingOperation poNewNode = new WSDLBindingOperation (getWSDLOwnerDocument(), getDomNode());
// cloneNodeHelper (poNewNode, bDeep);
// poNewNode.mpOperation = mpOperation;
// return poNewNode;
// }
/**
* get the WSDLOperation this binding operation binds.
* @return the WSDLOperation node this binding operation binds.
*/
public WSDLOperation getOperation () {
return mpOperation;
}
void setOperation (WSDLOperation operation) {
mpOperation = operation;
}
/**
* Get the node .
* @return the WSDLNode that the soap:operation child of this node.
*/
public WSDLExten getSoapOperation () {
if (moSoapOperation == null) {
moSoapOperation = findChildByExtenType (WSDLExten.EX_TYPE_SOAP_OPERATION);
}
return moSoapOperation;
}
/**
* Get the soap:binding node that is a sibling of this node.
* @return the WSDLNode that is the soap:binding node associated with this node.
*/
public WSDLExten getSoapBinding () {
if (moSoapBinding == null) {
moSoapBinding = findChildByExtenType (getWSDLParentNode(), WSDLExten.EX_TYPE_SOAP_BINDING);
}
return moSoapBinding;
}
/**
* Get the SOAP style of this operation.
* @return SOAP style.
*/
public SoapStyle getSoapStyle () {
int outStyle = WSDLBindingOperation.SOAP_STYLE_DOC;
int outInputUse = WSDLBindingOperation.SOAP_USE_LITERAL;
int outOutputUse = WSDLBindingOperation.SOAP_USE_LITERAL;
String sStyle = null;
// check the soap:operation style attribute first
WSDLExten soapOperation = getSoapOperation();
if (soapOperation != null) {
sStyle = soapOperation.getExtenAttribute (WSDLExten.EX_ATTR_SOAP_STYLE);
}
if (StringUtils.isEmpty(sStyle)) {
// check the soap:binding style attribute next
WSDLExten soapBinding = getSoapBinding();
if (soapBinding != null) {
sStyle = soapBinding.getExtenAttribute (WSDLExten.EX_ATTR_SOAP_STYLE);
}
}
if (sStyle == WSDL.RPC) {
outStyle = WSDLBindingOperation.SOAP_STYLE_RPC;
} else if (StringUtils.isEmpty (sStyle) || StringUtils.equalsWithNull (sStyle, WSDL.DOCUMENT)) {
outStyle = WSDLBindingOperation.SOAP_STYLE_DOC; // the default is document
}
// check the ecoding in the soap:body use attribute for the Input and Output children nodes
String sInputUse = null;
WSDLExten inputSoapBody = getInputSoapBody();
if (inputSoapBody != null) {
sInputUse = inputSoapBody.getExtenAttribute (WSDLExten.EX_ATTR_SOAP_USE);
}
String sOutputUse = null;
WSDLExten outputSoapBody = getOutputSoapBody();
if (outputSoapBody != null) {
sOutputUse = outputSoapBody.getExtenAttribute (WSDLExten.EX_ATTR_SOAP_USE);
}
if (sInputUse == WSDL.ENCODED) {
outInputUse = WSDLBindingOperation.SOAP_USE_ENCODED;
} else if (StringUtils.isEmpty (sInputUse) || StringUtils.equalsWithNull (sInputUse, WSDL.LITERAL)) {
outInputUse = WSDLBindingOperation.SOAP_USE_LITERAL; // the default is literal
}
if (sOutputUse == WSDL.ENCODED) {
outOutputUse = WSDLBindingOperation.SOAP_USE_ENCODED;
} else if (StringUtils.isEmpty (sOutputUse) || StringUtils.equalsWithNull (sOutputUse, WSDL.LITERAL)) {
outOutputUse = WSDLBindingOperation.SOAP_USE_LITERAL; // the default is literal
}
return new SoapStyle (outStyle, outInputUse, outOutputUse);
}
/**
* returns whether or not this operation uses the httpTransport.
* @return a Bool indicating whether this operation uses the httpTransport.
*/
public boolean usesHTTPTransport () {
String sTransport = null;
WSDLExten soapBinding = getSoapBinding();
if (soapBinding != null) {
sTransport = soapBinding.getExtenAttribute (WSDLExten.EX_ATTR_SOAP_TRANSPORT);
}
return sTransport == WSDL.SOAP_HTTP_TRANSPORT;
}
/**
* Get the element for the input.
*
* @return The element for the input.
*/
public WSDLExten getInputSoapBody () {
if (moInputSoapBody == null) {
moInputSoapBody = getSoapBody (WSDLNode.WSDL_INPUT);
}
return moInputSoapBody;
}
/**
* Get the element for the output.
*
* @return The element for the output.
*/
public WSDLExten getOutputSoapBody () {
if (moOutputSoapBody == null) {
moOutputSoapBody = getSoapBody (WSDLNode.WSDL_OUTPUT);
}
return moOutputSoapBody;
}
private WSDLExten getSoapBody (int inNodeType) {
// get the child node should be either WSDL_INPUT OR WSDL_OUTPUT
WSDLNode childNode = getFirstWSDLNode (inNodeType);
if (childNode == null) {
return null;
}
return findChildByExtenType (childNode, WSDLExten.EX_TYPE_SOAP_BODY);
}
private final WSDLExten findChildByExtenType (int type) {
return findChildByExtenType (this, type);
}
private static final WSDLExten findChildByExtenType (WSDLNode parent, int type) {
WSDLNode node = parent.getFirstWSDLNode (WSDL_EXTEN);
while (node != null) {
if (node instanceof WSDLExten) { // it should be
WSDLExten exten = (WSDLExten) node;
if (exten.getExtenType() == type) {
return exten;
}
}
node = node.getNextWSDLNode (WSDL_EXTEN);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy