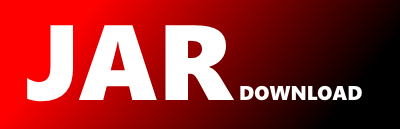
com.day.cq.commons.ConsoleUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.commons;
import static com.day.cq.commons.jcr.JcrConstants.JCR_DESCRIPTION;
import static com.day.cq.commons.jcr.JcrConstants.JCR_TITLE;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import javax.jcr.Node;
import javax.jcr.NodeIterator;
import javax.jcr.RepositoryException;
import javax.jcr.observation.EventIterator;
import javax.jcr.observation.EventListener;
import javax.jcr.observation.ObservationManager;
import javax.jcr.query.Query;
import javax.jcr.query.QueryManager;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Small tool to gather all consoles once. If consoles list need to be refreshed you need to create new
* instance of ConsoleUtil
*/
@Deprecated
public class ConsoleUtil implements EventListener {
private static final String NT_CONSOLE = "cq:Console";
private static final String PR_CONSOLE_TITLE = "consoleTitle";
private static final String PR_CONSOLE_DESCRIPTION = "consoleDescription";
private static final String PR_VANITY_PATH = "sling:vanityPath";
private static final String QUERY_BASE = "SELECT * FROM ";
private static final String QUERY_CONSOLE = QUERY_BASE + NT_CONSOLE;
private static final String CLAUSE_ORDER = " ORDER BY sling:vanityOrder DESC";
private final QueryManager queryManager;
private final String[] queries;
private Collection consoles;
private final static Logger log = LoggerFactory.getLogger(ConsoleUtil.class);
/**
* Construct a Tool with Query- and Observation-facility to gather list of cq:Console node types.
* If this object is not needed anymore {@link #dispose()} should be called.
* @param queryManager to search for consoles
* @param observationManger - this is not used anymore
* @param searchPath resolvers search path to limit search
*/
public ConsoleUtil(QueryManager queryManager, ObservationManager observationManger, String[] searchPath) {
this.queryManager = queryManager;
this.queries = new String[searchPath.length];
if (searchPath.length>0) {
for (int i=0;i getPaths() {
if (this.consoles==null) {
try {
this.consoles = collectConsoles();
} catch (RepositoryException e) {
log.error("Failed to build paths to Console: {}", e);
throw new SlingRepositoryException(e.getMessage(),e);
}
}
return Collections.unmodifiableCollection(this.consoles);
}
//------------------------------------------------< EventListener >---------
/**
* Does nothing now.
*/
public void onEvent(EventIterator eventIterator) {
}
/**
* @return search all nodes for specified console type using query-manager
* @throws RepositoryException in case of error accessing repository
*/
private Collection collectConsoles() throws RepositoryException {
Collection relativePaths = new ArrayList();
Collection consoles = new ArrayList();
for (String query : queries) {
Query q = queryManager.createQuery(query, Query.SQL);
NodeIterator itr = q.execute().getNodes();
while(itr.hasNext()) {
Node node = itr.nextNode();
String path = node.getPath();
String appName = null;
if (node.hasProperty(PR_CONSOLE_TITLE)) {
appName = node.getProperty(PR_CONSOLE_TITLE).getString();
}
if (appName == null && node.hasProperty(JCR_TITLE)) {
appName = node.getProperty(JCR_TITLE).getString();
}
if (appName == null) {
appName = node.getName();
}
String appDescription = null;
if (node.hasProperty(PR_CONSOLE_DESCRIPTION)) {
appDescription = node.getProperty(PR_CONSOLE_DESCRIPTION).getString();
}
if (appDescription == null && node.hasProperty(JCR_DESCRIPTION)) {
appDescription = node.getProperty(JCR_DESCRIPTION).getString();
}
if (appDescription == null) {
appDescription = "";
}
String iconClass = null;
if (node.hasProperty("iconClass")) {
iconClass = node.getProperty("iconClass").getString();
}
String vanityPath = null;
if (node.hasProperty(PR_VANITY_PATH)) {
vanityPath = node.getProperty(PR_VANITY_PATH).getString();
}
String relativePath = path.substring(1);
relativePath = relativePath.substring(relativePath.indexOf("/") + 1);
if (!relativePaths.contains(relativePath)) { // if contained, it has been overlayed
consoles.add(new Console(appName, appDescription, iconClass, path, vanityPath));
relativePaths.add(relativePath);
}
}
}
return consoles;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy