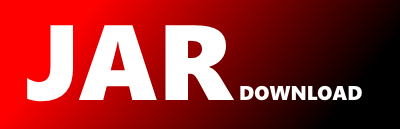
com.day.cq.commons.TidyJsonItemWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.commons;
import java.io.Writer;
import java.util.Set;
import javax.jcr.Node;
import javax.jcr.NodeIterator;
import javax.jcr.Property;
import javax.jcr.RepositoryException;
import javax.jcr.ValueFormatException;
import org.apache.sling.commons.json.JSONException;
import org.apache.sling.commons.json.io.JSONWriter;
import org.apache.sling.commons.json.jcr.JsonItemWriter;
/**
* Extension of the JsonItemWriter that uses a {@link TidyJSONWriter}.
*/
public class TidyJsonItemWriter extends JsonItemWriter {
/**
* flag indicating if output should be nicely formatted
*/
private boolean tidy;
/**
* Constructor
* @param propertyNamesToIgnore See javadoc of constructor of {@link JsonItemWriter}
*/
public TidyJsonItemWriter(Set propertyNamesToIgnore) {
super(propertyNamesToIgnore);
}
/**
* Checks if the output is nicely formatted.
* @return true
if nicely formatted
*/
public boolean isTidy() {
return tidy;
}
/**
* Controls if output should be nicely formatted.
* @param tidy true
to nicely format.
*/
public void setTidy(boolean tidy) {
this.tidy = tidy;
}
/**
* Dump given node in JSON, optionally recursing into its child nodes
* @param node node to dump
* @param w {@link JSONWriter}
* @param maxRecursionLevels max recursion level
* @throws RepositoryException if some error occurs
* @throws JSONException if some error occurs
*/
public void dump(Node node, JSONWriter w, int maxRecursionLevels)
throws RepositoryException, JSONException {
dump(node, w, 0, maxRecursionLevels);
}
/**
* {@inheritDoc}
*/
public void dump(Node node, Writer w, int maxRecursionLevels)
throws RepositoryException, JSONException {
TidyJSONWriter jw = new TidyJSONWriter(w);
jw.setTidy(tidy);
dump(node, jw, 0, maxRecursionLevels);
}
/**
* Dump all Nodes of given NodeIterator in JSON
* @param it {@link NodeIterator}
* @param w {@link JSONWriter}
* @throws RepositoryException if some error occurs
* @throws JSONException if some error occurs
*/
public void dump(NodeIterator it, JSONWriter w) throws RepositoryException, JSONException {
w.array();
while (it.hasNext()) {
dumpSingleNode(it.nextNode(), w, 1, 0);
}
w.endArray();
}
/**
* {@inheritDoc}
*/
public void dump(NodeIterator it, Writer out) throws RepositoryException, JSONException {
TidyJSONWriter w = new TidyJSONWriter(out);
w.setTidy(tidy);
w.array();
while (it.hasNext()) {
dumpSingleNode(it.nextNode(), w, 1, 0);
}
w.endArray();
}
/**
* Dump the property in JSON
* @param p property to dump
* @param w {@link JSONWriter}
* @throws RepositoryException if some error occurs
* @throws JSONException if some error occurs
*/
public void dump(Property p, JSONWriter w) throws JSONException, RepositoryException {
w.object();
writeProperty(w, p);
w.endObject();
}
/**
* {@inheritDoc}
*/
public void dump(Property p, Writer w) throws JSONException, ValueFormatException, RepositoryException {
TidyJSONWriter jw = new TidyJSONWriter(w);
jw.setTidy(tidy);
jw.object();
writeProperty(jw, p);
jw.endObject();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy