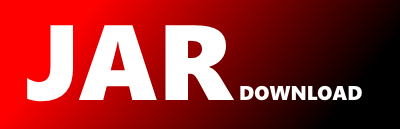
com.day.cq.dam.api.renditions.RenditionMaker Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2014 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.api.renditions;
import java.util.List;
import aQute.bnd.annotation.ProviderType;
import com.day.cq.dam.api.Asset;
import com.day.cq.dam.api.Rendition;
/**
* Service interface for creating standard thumbnail, web and other renditions
* for DAM assets.
*
* @since 6.0
*/
@ProviderType
public interface RenditionMaker {
/**
* Generates or updates multiple renditions for the given asset based on the given templates.
* All successfully generated renditions will be returned. In case one generation
* failed, it will not be part of the returned list.
*
* @param asset asset for which to create or update renditions
* @param templates a vararg list of rendition templates
* @return a list with all renditions that were successfully created or updated
*/
List generateRenditions(Asset asset, RenditionTemplate... templates);
/**
* Defines a template for a standard DAM PNG thumbnail rendition.
*
*
* The aspect ratio of the assets base image will be preserved. Depending on orientation,
* either width or height of the target size will be smaller.
*
*
* If center=true
, the rendition image will have the exact given target size
* and the resized image, which still keeps the original aspect ratio, will be centered with
* possible transparent whitespace around it to fill up to the target size.
*
* @param asset asset that is the base for the thumbnail
* (could be different from the asset on which the template will be applied)
* @param width target width of the thumbnail
* @param height target height of the thumbnail
* @param center true
if the thumbnail should have the exact target size
* and the resized image would be centered with transparent whitespace around it
* @return a rendition template
*/
RenditionTemplate createThumbnailTemplate(
Asset asset,
int width,
int height,
boolean center
);
/**
* Defines a template for a standard DAM web enabled rendition.
*
*
* The aspect ratio of the assets base image will be preserved. Depending on orientation,
* either width or height of the target size will be smaller.
*
* @param asset asset that is the base for the web rendition
* (could be different from the asset on which the template will be applied)
* @param width target width of the web rendition
* @param height target height of the web rendition
* @param quality for jpegs, the jpeg quality from 0 to 100; for gifs, the number of colors in the palette
* from 0 to 256
* @param mimeType mime type of the new web rendition unless asset mime type is in 'mimeTypesToKeep'
* @param mimeTypesToKeep list of asset mime types that will be used for the rendition as well, in which
* case the parameter 'mimeType' will be ignored
* @return a rendition template
*/
RenditionTemplate createWebRenditionTemplate(
Asset asset,
int width,
int height,
int quality,
String mimeType,
String[] mimeTypesToKeep
);
/**
* Defines a template for a standard DAM PNG thumbnail rendition from a
* given rendition.
*
* The aspect ratio of the assets base image will be preserved. Depending on
* orientation, either width or height of the target size will be smaller.
*
* If center=true
, the rendition image will have the exact
* given target size and the resized image, which still keeps the original
* aspect ratio, will be centered with possible transparent whitespace
* around it to fill up to the target size.
* @since 6.1
* @param rendition rendition that is the base for the thumbnail. It could
* be any rendition of the asset.
* @param width target width of the thumbnail
* @param height target height of the thumbnail
* @param center true
if the thumbnail should have the exact
* target size and the resized image would be centered with
* transparent whitespace around it
* @return a rendition template
*/
RenditionTemplate createThumbnailTemplate(Rendition rendition, int width, int height, boolean center);
/**
* Defines a template for a standard DAM web enabled rendition from a given
* rendition. The aspect ratio of the assets base image will be preserved.
* Depending on orientation, either width or height of the target size will
* be smaller.
* @since 6.1
* @param rendition rendition that is the base for the web rendition. It
* could be any rendition of the asset.
* @param width target width of the web rendition
* @param height target height of the web rendition
* @param quality for jpegs, the jpeg quality from 0 to 100; for gifs, the
* number of colors in the palette from 0 to 256
* @param mimeType mime type of the new web rendition unless asset mime type
* is in 'mimeTypesToKeep'
* @param mimeTypesToKeep list of asset mime types that will be used for the
* rendition as well, in which case the parameter 'mimeType' will
* be ignored
* @return a rendition template
*/
RenditionTemplate createWebRenditionTemplate(Rendition rendition, int width, int height, int quality,
String mimeType, String[] mimeTypesToKeep);
/**
* Defines a template for a rendition with the given name. The aspect ratio of the assets base image will be preserved.
* Depending on orientation, either width or height of the target size will
* be smaller.
* @param asset asset that is the base for the thumbnail. It
* could be any rendition of the asset.
* @param renditionName rendition name to be used.
* @param width target width of the web rendition
* @param height target height of the web rendition
* @param quality for jpegs, the jpeg quality from 0 to 100; for gifs, the
* number of colors in the palette from 0 to 256
* @param mimeType mime type of the new web rendition unless asset mime type
* is in 'mimeTypesToKeep'
* @param mimeTypesToKeep list of asset mime types that will be used for the
* rendition as well, in which case the parameter 'mimeType' will
* be ignored
* @return a rendition template
**/
RenditionTemplate createRenditionTemplate(Asset asset, String renditionName, int width, int height, int quality,
String mimeType, String[] mimeTypesToKeep);
}