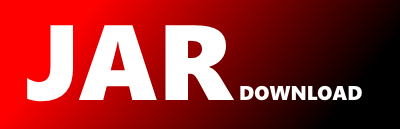
com.day.cq.dam.commons.util.DynamicMediaHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.day.cq.dam.commons.util;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import org.apache.sling.api.resource.ValueMap;
import org.apache.sling.api.wrappers.ValueMapDecorator;
import java.util.Collections;
import com.adobe.cq.dam.dm.delivery.api.ImageDelivery;
import org.osgi.framework.BundleContext;
import org.osgi.framework.BundleReference;
import org.osgi.framework.ServiceReference;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Utility helper class to facilitate access to Dynamic Media configuration
* status
*/
public class DynamicMediaHelper {
// Dynamic Media configuration node path
private static final String DYNAMIC_MEDIA_CONFIG_PATH = "/etc/dam/dynamicmediaconfig";
// Configuration node properties
private static final String PN_DYNAMIC_MEDIA_ENABLED = "dynamicMediaEnabled";
private static final Logger LOG = LoggerFactory.getLogger(DynamicMediaHelper.class);
/**
* Helper method to determine if Dynamic Media plugin is enabled or not
*
* @param resolver a {@link ResourceResolver} to be used for the operation
* @return {@code Boolean} indicating if Dynamic Media is enabled or not
* @deprecated Use isEnabled() method provided by ImageDelivery service instead
*/
@Deprecated
public static boolean isDynamicMediaEnabled(ResourceResolver resolver) {
BundleContext ctx =
BundleReference.class.cast(ImageDelivery.class.getClassLoader())
.getBundle()
.getBundleContext();
ServiceReference serviceReference = ctx.getServiceReference(ImageDelivery.class.getName());
ImageDelivery service = ImageDelivery.class.cast(ctx.getService(serviceReference));
try {
return service != null && service.isEnabled();
} finally {
ctx.ungetService(serviceReference);
}
}
/**
* Attempts to retrieve a {@link ValueMap} containing the properties of the dm config node indicated by the path
*
* @param resolver a {@link ResourceResolver} to be used in the operation
* @return a {@link ValueMap} containing the DM configuration or an empty {@link ValueMap} in case of any error
*/
private static ValueMap getDynamicMediaConfigurationProperties(ResourceResolver resolver) {
ValueMap configProps = null;
try {
Resource dmConfigResource = resolver.getResource(DYNAMIC_MEDIA_CONFIG_PATH);
if(dmConfigResource != null) {
configProps = dmConfigResource.adaptTo(ValueMap.class);
}
} catch (Exception e) {
LOG.error("Could not read the Dynamic Media configuration from node " + DYNAMIC_MEDIA_CONFIG_PATH, e);
}
// return an empty map if config map is null
if (configProps == null) {
configProps = new ValueMapDecorator(Collections.emptyMap());
}
return configProps;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy